Polymorphism in Python
- Polymorphism in Python
- Use Class Methods for Polymorphism in Python
- Use Method Overriding for Polymorphism in Python
- Use Function and Operator Overloading for Polymorphism in Python
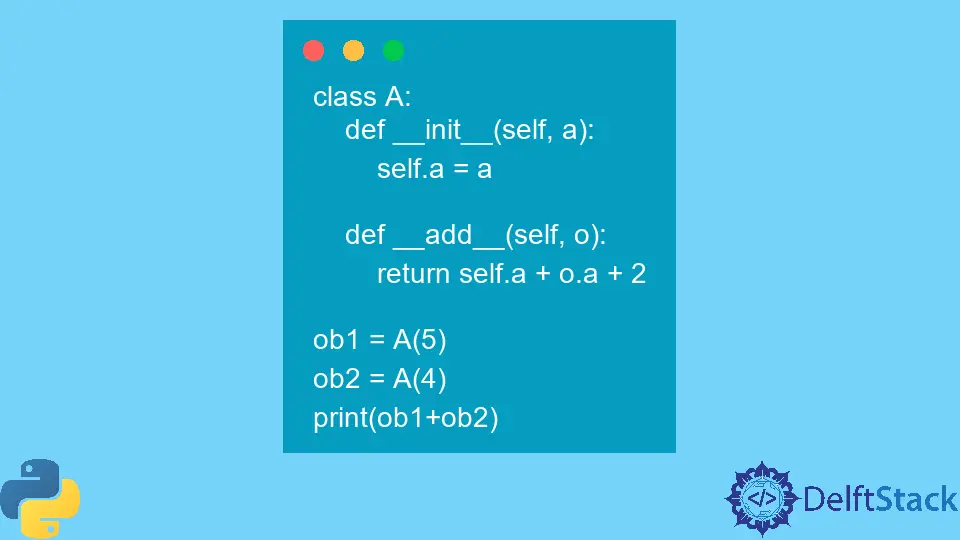
Polymorphism is a characteristic of OOPs, which signifies that a single name can have different functions. A single entity can take different forms.
This tutorial will demonstrate how Polymorphism is implemented in Python.
Polymorphism in Python
The best way to understand this is by using the len()
function. This function is interpreted differently for different objects.
For a list, it will return the total elements present and for a string, and the total characters present.
Code:
lst = ["delft", "stack"]
s = "delftstack"
print(len(lst), len(s))
Output:
2 10
Another common functionality that illustrates Polymorphism in Python is the +
operator. This operator can perform addition between two numbers in Python.
However, when used with a string, it acts as a concatenation operator to combine two strings.
Code:
a = 5 + 6
s = "delft" + "stack"
print(a, s)
Output:
11 delftstack
Use Class Methods for Polymorphism in Python
Everything is an object in Python and belongs to some class. The methods are associated with the objects of the class.
That is why the +
operator and len()
function perform different functions for different objects. The +
operator calls a magic function defined differently for different objects.
We can create our class and achieve this. Two classes can have functions with the same name but have different purposes and different definitions.
Code:
class A:
def fun(self):
print("Class A")
class B:
def fun(self):
print("Class B")
ob1 = A()
ob2 = B()
for i in (ob1, ob2):
i.fun()
Output:
Class A
Class B
Use Method Overriding for Polymorphism in Python
Polymorphism is also associated in Python with class inheritance. Inheritance refers to a class accessing the features of another class.
A class and its subclass may contain the function with the same name, and we can use function overriding in Python. Using method overriding, we can implement the already defined method in the child class.
We can redefine the function according to the child class.
Code:
class A:
def fun(self):
print("Class A")
class B(A):
def fun(self):
print("Class B")
ob1 = A()
ob2 = B()
for i in (ob1, ob2):
i.fun()
Output:
Class A
Class B
In the above code example, class B
inherits from the class A
, but the fun()
method is overridden in class B
and is defined according to the class.
We can also access the function fun()
from class A
in the overridden function.
Code:
class A:
def fun(self):
print("Class A")
class B(A):
def fun(self):
A.fun(self)
print("Class B")
ob2 = B()
ob2.fun()
Output:
Class A
Class B
Use Function and Operator Overloading for Polymorphism in Python
Function overloading is an interesting feature that implements Polymorphism. This feature is not available in Python, and the most recent defined function will be called in Python.
It is possible to overload operators in Python. We know that different operators can perform different functions based on the object type, clearly visible with the +
operator.
This happens because it invokes a magic function internally whenever an operator is used. For example, the +
operator invokes the __add__
method internally.
This magic function has double underscores before and after its name. Magic functions are not meant to be invoked directly, but we can define them for different classes to modify the behavior of different operators.
Code:
class A:
def __init__(self, a):
self.a = a
def __add__(self, o):
return self.a + o.a + 2
ob1 = A(5)
ob2 = A(4)
print(ob1 + ob2)
Output:
11
In the above example, we overloaded the +
operator by modifying the magic function of class A
and adding 2
to the result.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn