Conditional Assignment Operator in Python
-
Meaning of
||=
Operator in Ruby -
Implement Ruby’s
||=
Conditional Assignment Operator in Python Using thetry...except
Statement -
Implement Ruby’s
||=
Conditional Assignment Operator in Python Usinglocal
andglobal
Variables
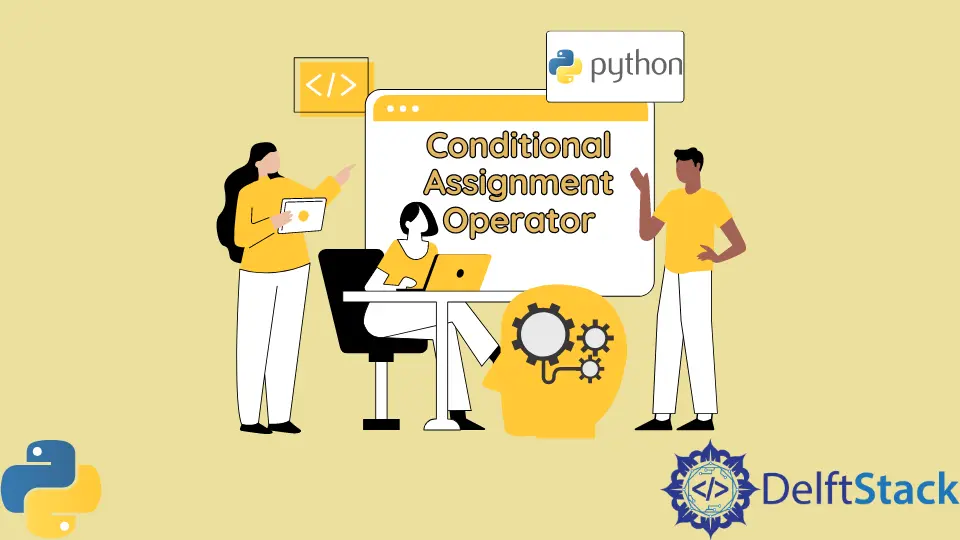
There isn’t any exact equivalent of Ruby’s ||=
operator in Python. However, we can use the try...except
method and concepts of local and global variables to emulate Ruby’s conditional assignment operator ||=
in Python.
Meaning of ||=
Operator in Ruby
x ||= y
The basic meaning of this operator is to assign the value of the variable y
to variable x
if variable x
is undefined or is falsy
value, otherwise no assignment operation is performed.
But this operator is much more complex and confusing than other simpler conditional operators like +=
, -=
because whenever any variable is encountered as undefined, the console throws out NameError
.
a+=b
evaluates to a=a+b
.
a||=b
looks as a=a||b
but actually behaves as a||a=b
.
Implement Ruby’s ||=
Conditional Assignment Operator in Python Using the try...except
Statement
We use try...except
to catch and handle errors. Whenever the try except
block runs, at first, the code lying within the try
block executes. If the block of code within the try
block successfully executes, then the except
block is ignored; otherwise, the except
block code will be executed, and the error is handled. Ruby’s ||=
operator can roughly be translated in Python’s try-catch
method as :
try:
x
except NameError:
x = 10
Here, if the variable x
is defined, the try
block will execute smoothly with no NameError
exception. Hence, no assignment operation is performed. If x
is not defined, the try
block will generate NameError
, then the except
block gets executed, and variable x
is assigned to 10
.
Implement Ruby’s ||=
Conditional Assignment Operator in Python Using local
and global
Variables
The scope of local variables is confined within a specific code scope, whereas global variables have their scope defined in the entire code space.
All the local variables in a particular scope are available as keys of the locals
dictionary in that particular scope. All the global variables are stored as keys of the globals
dictionary. We can access those variables whenever necessary using the locals
and the globals
dictionary.
We can check if a variable exists in any of the dictionaries and set its value only if it does not exist to translate Ruby’s ||=
conditional assignment operator in Python.
if x in locals().keys():
locals().get(x)
elif x in globals().keys():
globals().get(x)
else:
x = 10
Here, if the variable x
is present in either global or local scope, we don’t perform any assignment operation; otherwise, we assign the value of x
to 10
. It is similar to x||=10
in Ruby.