How to Unpack Operator ** in Python
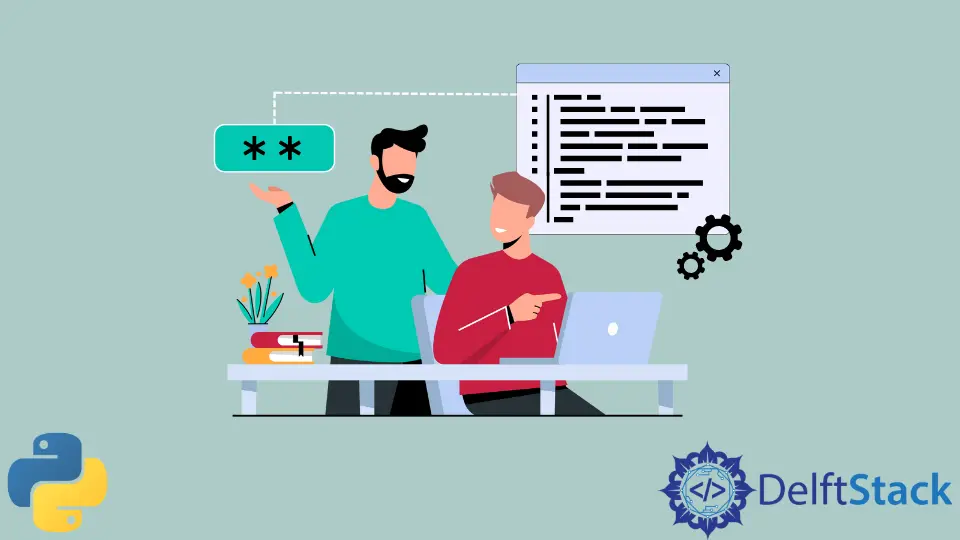
Python provides the **
and *
operators that can be used to unpack values from data structures like dictionaries, tuples, lists, and more.
Unpacking allows us to print the elements of the object or load them into other objects. The **
operator can be used to unpack values from a dictionary as key-value pairs.
This tutorial will demonstrate the use of the **
operator in Python.
Use the **
Operator in Python
We can unpack key-value pairs from a dictionary using the **
, and this unpacking technique can perform various operations. We will discuss many such operations below.
We can use this for merging dictionaries. We can create a dictionary and unpack the pairs of this dictionary into another one.
This will merge the two dictionaries. See the code below.
dict1 = {"x": 24, "y": 25}
dict2 = {"z": 26, **dict1}
print(dict2)
Output:
{'z': 26, 'x': 24, 'y': 25}
In the above example, we create a dictionary dict1
and unpack the pairs using the **
operator in the dictionary dict2
, which merges the pairs in the dict2
dictionary.
The most important use of the **
operator comes while sending multiple arguments to a function. One must have seen the use of the kwargs
keyword in function definitions.
We use this in a function definition, which is considered a standard notation when we do not know the total parameters of the function during a function call.
We can send multiple parameters to a function using the **kwargs
notation in the function definition.
Note that the keyword kwargs
is considered a standard notation and can be replaced by any logical variable name. The important thing here is the **
operator.
This operator will unpack the parameters received when multiple keyword parameters are provided during a function call. Remember that this works with keyword parameters because it will unpack the values as key-value pairs from a dictionary, and every parameter has a keyword.
It will be clear by the use of an example. See the code below.
def sample(a, **kwargs):
print("Keyword Parameters using **")
for i in kwargs:
print(i, kwargs[i])
print("Positional Parameter value", a)
sample(7, x=24, y=25, z=26)
Output:
Keyword Parameters using **
x 24
y 25
z 26
Positional Parameter value 7
In the above example, we send multiple keyword parameters to the function. They are unpacked at function call using the **
operator and used as required.
The *
operator in Python can also be used to unpack values from lists, tuples, and more. Similar to what we discussed, it can be used to send multiple positional parameters during a function call.
Conclusion
This tutorial demonstrated the use of the **
operator in Python. We demonstrated how it is used to unpack values from a dictionary in Python.
This feature allows it to be used in various operations like merging dictionaries, sending multiple keyword parameters, and more. We also discussed the *
operator that can unpack values from a list, tuple, and more similarly.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn