Python Bitwise NOT
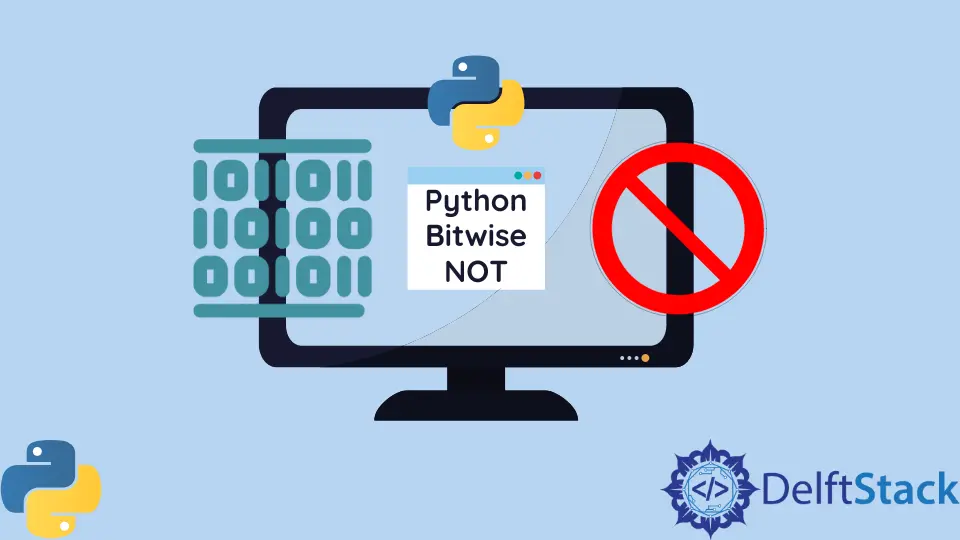
Bitwise operations in Python allow you to do the most precise manipulations on those individual data bits. Python operators support bitwise logical operations on integers.
In this article, we’ll discuss the Bitwise NOT
Operator in Python.
Bitwise NOT
Operator in Python
A Bitwise NOT
(or complement), a unary operation that conducts logical negation on each bit to create the one complement of the provided binary value, is a bitwise operation. Bits that are 0 change to 1, whereas bits that are 1 change to 0.
Bitwise NOT
is equivalent to the value’s two’s complement less one. A particular bit called the sign bit is used to store whether an integer is positive or negative (the integer’s sign), and any positive number becomes a negative number and vice versa since this bit is also affected by the Bitwise NOT
.
Syntax:
~a
The Bitwise NOT
operator’s symbol is ~
.
In the following example, we’ve declared a variable a with a value of 1289
. Then, assign it to variable x
, in which you add the value of and complement value of a
, and then print the value of x
, which is -1
.
Example Code:
# Python 3.x
a = 1289
x = a + ~a
print(x)
Output:
#Python 3.x
-1
In this next code example, we declared a variable x
and assigned 20
. Then, we performed the Bitwise NOT
operation, which gives -21
, as shown in the output.
Example Code:
# Python 3.x
x = 20
print("~20 =", ~x)
Output:
#Python 3.x
~20 = -21
In this last example, we’ll initialize the data using the special init()
method for classes. The invert()
function computes bitwise inversion of data by reassigning the self attribute to the compliment.
Assign the data 3
to variable x
. The complement of x
is stored in the res
variable.
By printing the res. data
will give you -4
as the output.
Example Code:
# Python 3.x
class Data:
def __init__(self, data):
self.data = data
def __invert__(self):
return Data(~self.data)
x = Data(3)
res = ~x
print(res.data)
Output:
#Python 3.x
-4
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn