Binomial Coefficient in Python
- Binomial Coefficient in Python
-
Use the
scipy.special.binom()
Function to Calculate the Binomial Coefficient in Python -
Use the
scipy.special.comb()
Function to Calculate the Binomial Coefficient in Python -
Use the
math.comb()
Function to Calculate the Binomial Coefficient in Python -
Use the
operator
Module to Calculate the Binomial Coefficient in Python -
Use the
math.factorial()
Function to Calculate the Binomial Coefficient in Python - Conclusion
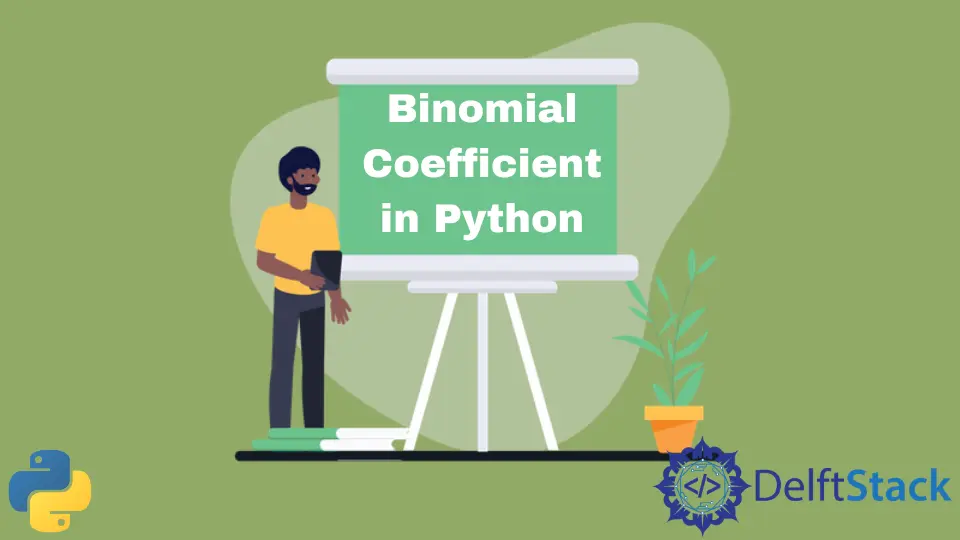
In this tutorial, we’ll dive into understanding and calculating binomial coefficients, a fundamental concept in combinatorics that holds immense importance in various areas of mathematics, statistics, and computer science.
Binomial Coefficient in Python
The binomial coefficient, denoted as "n choose k"
or "C(n, k)"
, calculates the number of ways to select 'k'
items from a set of 'n'
items without considering the order and despite its apparent simplicity, its importance lies in being a fundamental tool for solving more intricate mathematical and computational challenges.
The formula, $\binom{n}{k} = \frac{n!}{k!(n-k)!}$ quantifies unique combinations.
As an example, imagine assembling a team of 3 from 10 skilled individuals. The 'C(10, 3)'
yields 120
, representing the distinct team combinations.
Understanding binomial coefficients is vital for optimizing diverse aspects like team formation, skill distribution, and resource allocation in various fields.
Use the scipy.special.binom()
Function to Calculate the Binomial Coefficient in Python
The scipy.special.binom()
function is a powerful tool in Python for calculating the binomial coefficient. It is part of the scipy
library, which provides a wide range of scientific and mathematical functions.
Syntax:
scipy.special.binom(n, k)
The scipy.special.binom()
function takes two arguments: n
and k
, representing the number of objects in the set and the number of objects to be chosen, respectively. It returns the binomial coefficient C(n, k)
as an integer value.
With the scipy.binom()
function, we can easily compute binomial coefficients without implementing the formula from scratch.
Code Example:
import scipy.special
print(scipy.special.binom(10, 5))
Output:
252.0
The above code imports the scipy.special
module and uses the binom()
function to calculate the binomial coefficient. Specifically, it calculates C(10, 5)
, which represents the number of ways to choose 5
objects from a set of 10
.
The output is 252.0
. This implies that there are 252.0
distinct combinations of selecting 5
objects from a set of 10
, as calculated using the binomial coefficient formula.
This code demonstrates how to utilize the binom()
function from the scipy
library in Python to compute binomial coefficients easily.
Use the scipy.special.comb()
Function to Calculate the Binomial Coefficient in Python
The scipy.special.comb()
function is another powerful tool in Python for computing the binomial coefficient. It is part of the scipy.special
module, which provides various special mathematical functions.
Syntax:
scipy.special.comb(n, k, exact=False)
The scipy.special.comb()
function also takes two arguments: n
and k
, representing the number of objects in the set and the number of objects to be chosen, respectively. By default, exact=False
calculates the coefficient using floating-point arithmetic, but it can be set to True
for an exact integer result.
Compared to scipy.special.binom()
, the scipy.special.comb()
function provides additional flexibility with the exact
parameter. This allows us to obtain floating-point approximations or exact integer values, depending on our requirements.
Similar to the scipy.special.binom()
function, scipy.special.comb()
simplifies the calculation of binomial coefficients.
Code Example:
import scipy.special
print(scipy.special.comb(10, 5))
Output:
252.0
The above code imports the scipy.special
module and uses the comb()
function to calculate the binomial coefficient. Specifically, it calculates C(10, 5)
, which represents the number of ways to choose 5
objects from a set of 10
.
The output is 252.0
. This implies that there are 252.0
distinct combinations of selecting 5
objects from a set of 10
, as calculated using the binomial coefficient formula.
This code demonstrates how to utilize the scipy.special.comb()
function in Python to easily compute binomial coefficients, offering the flexibility to obtain either floating-point approximations or exact integer values based on the requirements.
Use the math.comb()
Function to Calculate the Binomial Coefficient in Python
The math.comb()
function is a built-in Python function that provides a straightforward way to calculate the binomial coefficient. It is part of the math
module, which offers various mathematical operations and functions.
Syntax:
math.comb(n, k)
The math.comb()
function takes two arguments: n
and k
, representing the number of objects in the set and the number of objects to be chosen, respectively. It returns the binomial coefficient C(n, k)
as an integer value.
Compared to the previously discussed scipy.special.binom()
and scipy.special.comb()
functions, the math.comb()
function offers a simpler alternative for computing binomial coefficients. However, it only returns exact integer values and does not provide the flexibility of obtaining floating-point approximations.
Using the math.comb()
function, we can efficiently calculate binomial coefficients in Python.
Code Example:
import math
print(math.comb(10, 5))
Output:
252
The above code imports the math
module and uses the comb()
function to calculate the binomial coefficient. Specifically, it calculates C(10, 5)
, which represents the number of ways to choose 5
objects from a set of 10
.
The result is then printed to the console. This code demonstrates how to utilize Python’s math.comb()
function to easily compute binomial coefficients, providing exact integer values without additional libraries or packages.
The output is 252
. This implies that there are 252
distinct combinations of selecting 5
objects from a set of 10
, as calculated using the binomial coefficient formula.
It’s important to note that the math.comb()
function is available in Python 3.8
and later versions. If you are using an older version of Python, you may encounter an AttributeError
as this function is not available in versions prior to Python 3.8
.
Use the operator
Module to Calculate the Binomial Coefficient in Python
In older versions of Python, math.factorial
is not present and, thus, could not be used. We can use the math
and operator
modules to compensate for this and generate the output in much less time.
While the operator
module is not specifically designed to calculate binomial coefficients, it provides a concise and efficient way to achieve the same result using other mathematical operations available in Python. The operator
module includes functions like mul
, truediv
, and sub
, which can be used with other built-in functions to compute the binomial coefficient.
Compared to the previously discussed methods, using the operator
module requires additional custom code but allows for customization and exploration of different mathematical operations to calculate binomial coefficients.
Code Example:
import operator
from functools import reduce
def product(m, n):
return reduce(operator.mul, range(m, n + 1), 1)
x = 10
y = 5
print(product(y + 1, x) / product(1, x - y))
Output:
252.0
The above code calculates the binomial coefficient C(10, 5)
using the operator
module and the reduce()
function from the functools
module. The code defines a lambda function called product
that calculates the product of a range of numbers using operator.mul
as the binary function, starting with an initial value of 1
.
The expression product(y+1, x)
computes the product of the range from 6
to 10
, while product(1, x-y)
computes the product of the range from 1
to 5
. Finally, the result of the first expression is divided by the result of the second expression, resulting in the binomial coefficient C(10, 5)
, which represents the number of ways to choose 5
objects from a set of 10
.
The output is 252.0
. This implies that there are 252.0
distinct combinations of selecting 5
objects from a set of 10
, as calculated using the binomial coefficient formula.
Use the math.factorial()
Function to Calculate the Binomial Coefficient in Python
The math.factorial()
function in Python is a built-in method that simplifies the calculation of factorials. It can also compute the binomial coefficient by dividing the factorial of n
by the product of k
and (n-k)
factorials.
Compared to the previously discussed methods, using math.factorial()
provides a basic yet reliable approach for calculating binomial coefficients. However, it may be less efficient and suitable for large numbers or scenarios that require precision control.
Code Example:
from math import factorial as fact
def binomial(n, r):
return fact(n) // fact(r) // fact(n - r)
print(binomial(10, 5))
Output:
252
The above code calculates the binomial coefficient C(10, 5)
using the factorial()
function from the math
module. The binomial(n,r)
function is defined, which takes two arguments, n
and r
, representing the number of objects in the set and the number of objects to be chosen, respectively.
Within the binomial(n,r)
function, the binomial coefficient is calculated using the formula fact(n) // fact(r) // fact(n - r)
. The double slashes (//
) ensure an integer result. Finally, the binomial(n,r)
function is called with arguments 10
and 5
, and the result is printed to the console, providing the binomial coefficient C(10, 5)
.
The output is 252
. This implies that there are 252
distinct combinations of selecting 5
objects from a set of 10
, as calculated using the binomial coefficient formula.
Conclusion
The scipy.special.binom()
function provides a convenient way to calculate the binomial coefficient, and the scipy.special.comb()
function adds flexibility to its advantages, allowing for both floating-point approximations and exact integer results. The only disadvantage is the use of an external library.
On the other hand, the math.comb()
is a built-in function but only provides integer results. It should be used in scenarios where we aren’t allowed to use external libraries and only need integer results.
The operator
module allows customization by combining different mathematical operations to calculate the binomial coefficient. It offers flexibility and control over intermediate steps but requires additional custom implementation and may need to be more intuitive than other methods.
The math.factorial()
function provides a simple and reliable way to calculate binomial coefficients. Still, this method explicitly calculates and divides factorials, which may lead to large numbers and potential precision limitations. It should be used when the precision limitations are acceptable for the specific use case.