Binomial Distribution in Python
- What is Binomial Distribution?
- Using Scipy to Calculate Binomial Distribution
- Visualizing Binomial Distribution with Matplotlib
- Cumulative Distribution Function with Scipy
- Conclusion
- FAQ
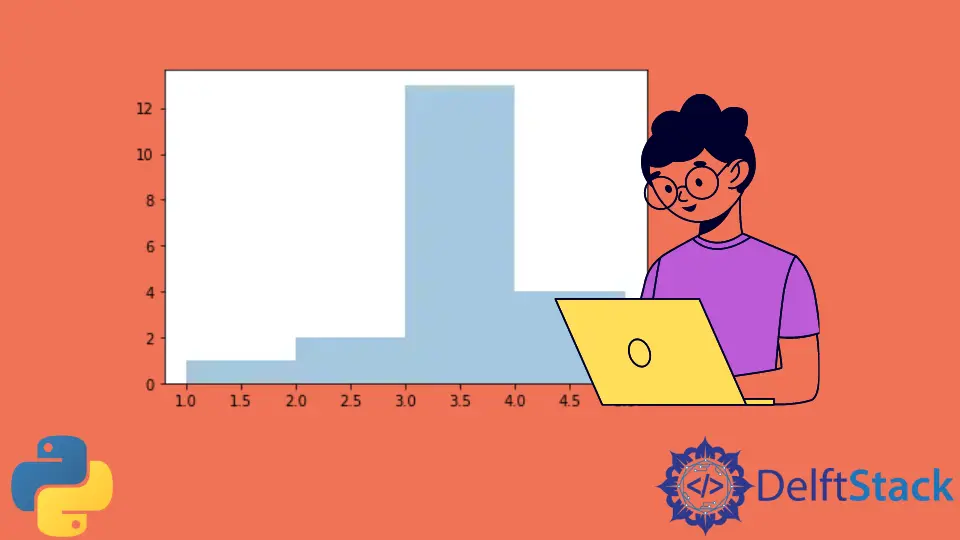
Understanding statistical concepts can be daunting, but with the right tools and explanations, it becomes much easier.
In this tutorial, we will dive into the binomial distribution, a fundamental concept in statistics, and explore how to implement it in Python. The binomial distribution models the number of successes in a fixed number of independent Bernoulli trials, each with the same probability of success. Whether you’re a data scientist, a student, or just someone curious about statistics, this guide will provide you with a solid foundation. We will cover the essentials of the binomial distribution and demonstrate how to use Python libraries to work with it effectively. By the end of this article, you’ll have a clear understanding of how to apply the binomial distribution in your projects.
What is Binomial Distribution?
Before we jump into coding, let’s briefly discuss what a binomial distribution is. The binomial distribution describes the probability of obtaining a fixed number of successes in a given number of trials. For example, if you flip a coin 10 times, the binomial distribution can help you calculate the probability of getting exactly 4 heads. The two key parameters of a binomial distribution are:
- n: The number of trials.
- p: The probability of success on each trial.
The formula for the binomial probability is given by:
[ P(X = k) = \binom{n}{k} p^k (1-p)^{n-k} ]
where ( k ) is the number of successes, and ( \binom{n}{k} ) is the binomial coefficient.
Using Scipy to Calculate Binomial Distribution
One of the most popular libraries for statistical calculations in Python is Scipy. It provides a straightforward way to work with the binomial distribution. To calculate probabilities, we can use the binom.pmf
function, which computes the probability mass function.
Here’s how you can use Scipy to calculate the probability of getting a specific number of successes in a series of trials:
from scipy.stats import binom
n = 10 # number of trials
p = 0.5 # probability of success
k = 4 # number of successes
probability = binom.pmf(k, n, p)
print(probability)
Output:
0.205078125
In this example, we set the number of trials ( n ) to 10 and the probability of success ( p ) to 0.5, which is typical for a fair coin flip. We want to find the probability of getting exactly 4 heads (successes). The binom.pmf
function computes the probability, which turns out to be approximately 0.205. This means there is a 20.5% chance of getting exactly four heads in ten flips of a fair coin.
Visualizing Binomial Distribution with Matplotlib
Visual representation can greatly enhance our understanding of statistical concepts. By using Matplotlib, we can create a visual plot of the binomial distribution. This helps in seeing how the probabilities are distributed across different numbers of successes.
Here’s how you can visualize the binomial distribution:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import binom
n = 10 # number of trials
p = 0.5 # probability of success
x = np.arange(0, n + 1)
pmf = binom.pmf(x, n, p)
plt.bar(x, pmf)
plt.title('Binomial Distribution PMF')
plt.xlabel('Number of Successes')
plt.ylabel('Probability')
plt.xticks(x)
plt.show()
Output:
[Bar plot of the binomial distribution]
In this code, we first import the necessary libraries. We define the number of trials ( n ) and the probability of success ( p ). Using numpy
, we create an array ( x ) that contains all possible numbers of successes from 0 to ( n ). The probability mass function is calculated using binom.pmf
. Finally, we use Matplotlib to create a bar plot that displays the probabilities of obtaining each number of successes. This visualization allows us to see the distribution shape, which is particularly useful for understanding how probabilities behave as we change the number of trials or the probability of success.
Cumulative Distribution Function with Scipy
The cumulative distribution function (CDF) is another important aspect of the binomial distribution. It provides the probability that the random variable is less than or equal to a certain value. Using Scipy, we can easily compute the CDF using the binom.cdf
function.
Here’s how to calculate and visualize the CDF:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import binom
n = 10 # number of trials
p = 0.5 # probability of success
x = np.arange(0, n + 1)
cdf = binom.cdf(x, n, p)
plt.step(x, cdf, where='post')
plt.title('Binomial Distribution CDF')
plt.xlabel('Number of Successes')
plt.ylabel('Cumulative Probability')
plt.xticks(x)
plt.show()
Output:
[Step plot of the cumulative distribution function]
In this example, we again set the number of trials ( n ) and the probability of success ( p ). We calculate the CDF using binom.cdf
and create a step plot using Matplotlib. The step plot visually represents the cumulative probabilities, allowing us to see how the likelihood of achieving a certain number of successes accumulates with each additional success. This is particularly useful for understanding the likelihood of various outcomes in experiments.
Conclusion
In this article, we explored the binomial distribution and how to implement it in Python using popular libraries like Scipy and Matplotlib. We covered the probability mass function, cumulative distribution function, and even visualized these concepts to enhance our understanding. Whether you’re analyzing data or working on a project that requires statistical modeling, the binomial distribution is a powerful tool in your arsenal. With the knowledge gained from this tutorial, you can confidently apply the binomial distribution in your work and further explore the world of statistics.
FAQ
-
What is the binomial distribution used for?
The binomial distribution is used to model the number of successes in a fixed number of independent trials, such as coin flips or quality control testing. -
How do you calculate the probability of success in Python?
You can calculate the probability of success using thebinom.pmf
function from the Scipy library. -
What is the difference between PMF and CDF?
PMF gives the probability of a specific number of successes, while CDF provides the cumulative probability of achieving a certain number of successes or fewer.
-
Can I visualize the binomial distribution?
Yes, you can visualize the binomial distribution using Matplotlib to create plots for both PMF and CDF. -
Is the binomial distribution applicable to all types of experiments?
The binomial distribution is applicable to experiments that meet the criteria of having a fixed number of trials, two possible outcomes, and constant probability of success.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn