How to Remove Pandas DataFrame Index
-
Remove Index of a Pandas DataFrame Using the
reset_index()
Method -
Remove Index of a Pandas DataFrame Using the
set_index()
Method
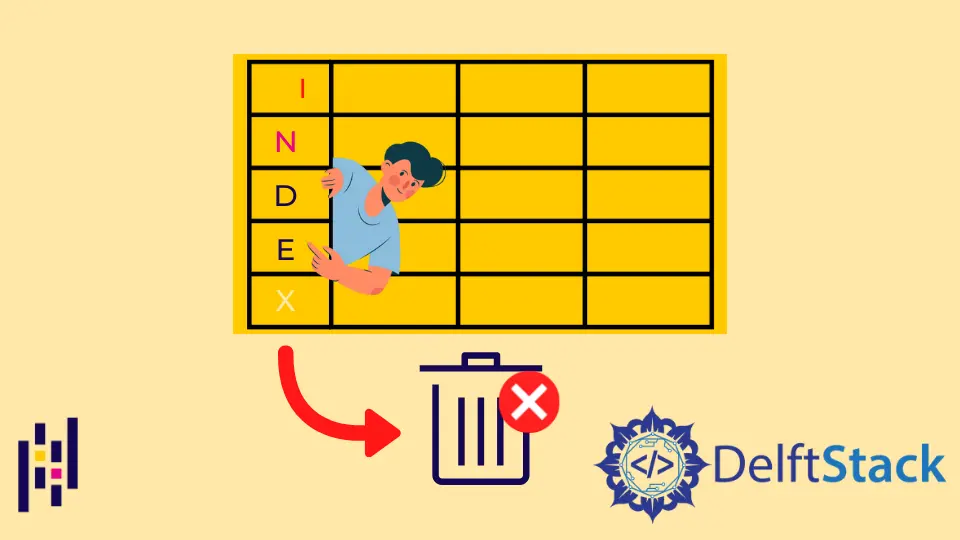
This tutorial will explain how we can remove the index of Pandas DataFrame.
We will use the DataFrame displayed below to show how we can remove the index.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
print(my_df)
Output:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
Remove Index of a Pandas DataFrame Using the reset_index()
Method
The pandas.DataFrame.reset_index()
method will reset the index of the DataFrame to the default index.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.reset_index()
print("Before reseting Index:")
print(my_df, "\n")
print("After reseting Index:")
print(df_reset)
Output:
Before reseting Index:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After reseting Index:
index Person City Mother Tongue Age
0 A Alice Berlin German 37
1 B Steven Montreal French 20
2 C Neesham Toronto English 38
3 D Chris Rome Italian 23
4 E Alice Munich German 35
It will reset the index of the my_df
DataFrame but the index will now appear as the index
column. If we want to drop the index
column, we can set drop=True
in the reset_index()
method.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.reset_index(drop=True)
print("Before reseting Index:")
print(my_df, "\n")
print("After reseting Index:")
print(df_reset)
Output:
Before reseting Index:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After reseting Index:
Person City Mother Tongue Age
0 Alice Berlin German 37
1 Steven Montreal French 20
2 Neesham Toronto English 38
3 Chris Rome Italian 23
4 Alice Munich German 35
Remove Index of a Pandas DataFrame Using the set_index()
Method
The pandas.DataFrame.set_index()
method will set the column passed as an argument as the index of the DataFrame overriding the initial index.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.set_index("Person")
print("Initial DataFrame:")
print(my_df, "\n")
print("After setting Person column as Index:")
print(df_reset)
Output:
Initial DataFrame:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After setting Person column as Index:
City Mother Tongue Age
Person
Alice Berlin German 37
Steven Montreal French 20
Neesham Toronto English 38
Chris Rome Italian 23
Alice Munich German 35
It sets the Person
column as an index of the my_df
DataFrame overriding the initial index of the DataFrame.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn