How to Get and Set Pandas DataFrame Index Name
- Get the Name of the Index Column of a DataFrame
-
Set the Name of the Index Column of a DataFrame by Setting the
name
Attribute -
Set the Name of Index Column of a DataFrame Using
rename_axis()
Method
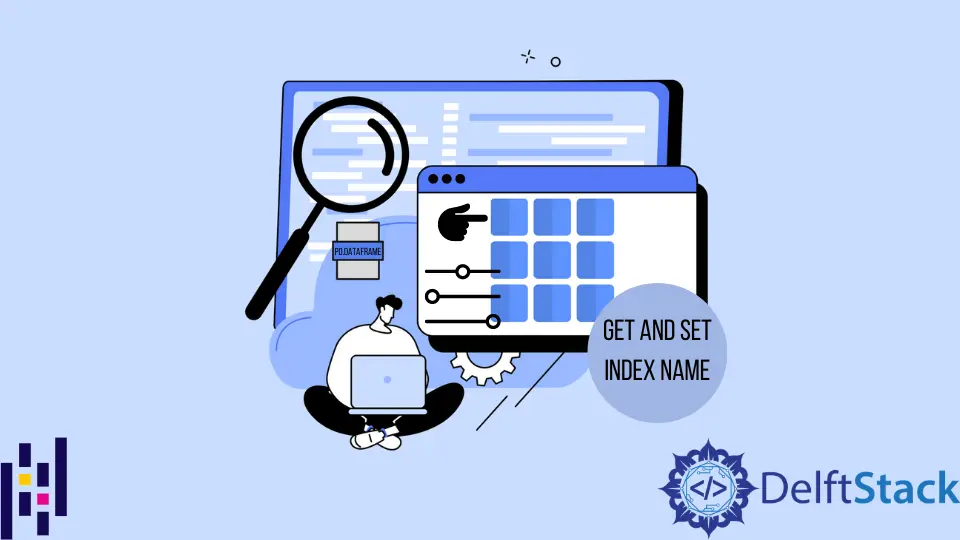
This tutorial explains how we can set and get the name of the index column of a Pandas DataFrame. We will use the below example DataFrame in the article.
import pandas as pd
my_df = pd.DataFrame(
{
"Applicant": ["Ratan", "Anil", "Mukesh", "Kamal"],
"Hometown": ["Delhi", "Pune", "Dhangadi", "Kolkata"],
"Score": [85, 87, 90, 89],
},
index=["2021-01-03", "2021-01-04", "2021-01-05", "2021-01-06"],
)
print(my_df)
Output:
Applicant Hometown Score
2021-01-03 Ratan Delhi 85
2021-01-04 Anil Pune 87
2021-01-05 Mukesh Dhangadi 90
2021-01-06 Kamal Kolkata 89
Get the Name of the Index Column of a DataFrame
We can get the name of the index column of the DataFrame using the name
attribute of the index column.
import pandas as pd
my_df = pd.DataFrame(
{
"Applicant": ["Ratan", "Anil", "Mukesh", "Kamal"],
"Hometown": ["Delhi", "Pune", "Dhangadi", "Kolkata"],
"Score": [85, 87, 90, 89],
},
index=["2021-01-03", "2021-01-04", "2021-01-05", "2021-01-06"],
)
print("The DataFrame is:")
print(my_df, "\n")
print("Name of Index Column of the DataFrame is:")
print(my_df.index.name)
Output:
The DataFrame is:
Applicant Hometown Score
2021-01-03 Ratan Delhi 85
2021-01-04 Anil Pune 87
2021-01-05 Mukesh Dhangadi 90
2021-01-06 Kamal Kolkata 89
Name of Index Column of the DataFrame is:
None
It gets the name of the index column of my_df
DataFrame as None
as we have not set the index column’s name for my_df
DataFrame.
Set the Name of the Index Column of a DataFrame by Setting the name
Attribute
We simply set the value of the name
attribute of the index
of the DataFrame to set the name of the index column of the DataFrame.
import pandas as pd
my_df = pd.DataFrame(
{
"Applicant": ["Ratan", "Anil", "Mukesh", "Kamal"],
"Hometown": ["Delhi", "Pune", "Dhangadi", "Kolkata"],
"Score": [85, 87, 90, 89],
},
index=["2021-01-03", "2021-01-04", "2021-01-05", "2021-01-06"],
)
print("Initial DataFrame:")
print(my_df, "\n")
my_df.index.name = "Date"
print("DataFrame after setting the name of Index Column:")
print(my_df, "\n")
print("Name of Index Column of the DataFrame is:")
print(my_df.index.name)
Output:
Initial DataFrame:
Applicant Hometown Score
2021-01-03 Ratan Delhi 85
2021-01-04 Anil Pune 87
2021-01-05 Mukesh Dhangadi 90
2021-01-06 Kamal Kolkata 89
DataFrame after setting the name of Index Column:
Applicant Hometown Score
Date
2021-01-03 Ratan Delhi 85
2021-01-04 Anil Pune 87
2021-01-05 Mukesh Dhangadi 90
2021-01-06 Kamal Kolkata 89
Name of Index Column of the DataFrame is:
Date
It sets the name of index
of my_df
to Date
.
Set the Name of Index Column of a DataFrame Using rename_axis()
Method
We can pass the index column’s name as an argument to the rename_axis()
method to set the name of the index column of the DataFrame.
import pandas as pd
my_df = pd.DataFrame(
{
"Applicant": ["Ratan", "Anil", "Mukesh", "Kamal"],
"Hometown": ["Delhi", "Pune", "Dhangadi", "Kolkata"],
"Score": [85, 87, 90, 89],
},
index=["2021-01-03", "2021-01-04", "2021-01-05", "2021-01-06"],
)
print("Initial DataFrame:")
print(my_df, "\n")
my_df = my_df.rename_axis("Date")
print("DataFrame after setting the name of Index Column:")
print(my_df, "\n")
print("Name of Index Column of the DataFrame is:")
print(my_df.index.name)
Output:
Initial DataFrame:
Applicant Hometown Score
2021-01-03 Ratan Delhi 85
2021-01-04 Anil Pune 87
2021-01-05 Mukesh Dhangadi 90
2021-01-06 Kamal Kolkata 89
DataFrame after setting the name of Index Column:
Applicant Hometown Score
Date
2021-01-03 Ratan Delhi 85
2021-01-04 Anil Pune 87
2021-01-05 Mukesh Dhangadi 90
2021-01-06 Kamal Kolkata 89
Name of Index Column of the DataFrame is:
Date
It sets the name of the index
column of the DataFrame my_df
to Date
using the rename_axis()
method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn