How to Revert From MultiIndex to Single Index in Pandas
- Rename the Columns to Standard Columns to Convert MultiIndex to Single Index in Pandas
- Reset the Levels of the Column to Convert MultiIndex to Single Index in Pandas
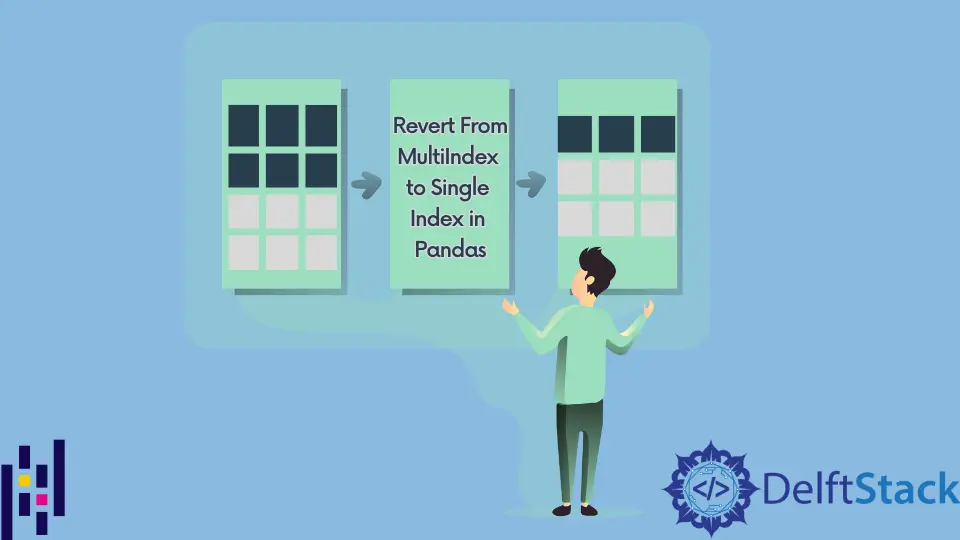
This tutorial teaches how to revert from MultiIndex to a single index dataframe in Pandas using Python.
A MultiIndex
dataframe, also called a multi-level and hierarchical dataframe, lets the user have multiple columns that can identify a row while having each column index related to each other through a different parent-child relationship in the table.
We can use two methods to convert multi-level indexing to single indexing. We will learn both the flexibility and increased efficiency in manipulating dataframes in Pandas.
Rename the Columns to Standard Columns to Convert MultiIndex to Single Index in Pandas
We must first create a dataframe consisting of MultiIndex columns in this method. After that, we can change the name of the columns, that is, the standard columns, so we can get rid of the MultiIndex with ease and without any errors.
Below is a code where the entire process for this method is followed right from the beginning.
import pandas as pd
import numpy as np
# build an example DataFrame
midx = pd.MultiIndex(
levels=[["zero", "one"], ["x", "y"]],
codes=[
[
1,
1,
0,
],
[
1,
0,
1,
],
],
)
df = pd.DataFrame(np.random.randn(2, 3), columns=midx)
print(df)
The code will give us the following output.
one zero
y x y
0 0.785806 -0.679039 0.513451
1 -0.337862 -0.350690 -1.423253
So, as we can observe, a dataframe has been created with multi-level index columns. To revert this column to single-level indexed, we need to rename them in the following method below.
df.columns = ["A", "B", "C"]
print(df)
The output for the above code is as follows.
A B C
0 0.785806 -0.679039 0.513451
1 -0.337862 -0.350690 -1.423253
The hierarchical indexing has been removed, and only new names are shown, replacing the older names of the columns.
Reset the Levels of the Column to Convert MultiIndex to Single Index in Pandas
In this method, we would just reset the levels of the MultiIndex columns to convert them into single-level columns.
The reset_index()
method lets the user reset the index of the dataframe and consider the default index again. One can use this method to remove one or more levels simultaneously.
We would do that by adding a single line to the previously used code snippet. Let us consider a different example to learn the technique more clearly and flexibly.
index = pd.MultiIndex.from_tuples(
[("bird", "falcon"), ("bird", "parrot"), ("mammal", "lion"), ("mammal", "monkey")],
names=["class", "name"],
)
columns = pd.MultiIndex.from_tuples([("speed", "max"), ("species", "type")])
df = pd.DataFrame(
[(389.0, "fly"), (24.0, "fly"), (80.5, "run"), (np.nan, "jump")],
index=index,
columns=columns,
)
print(df)
The above code will give us the following output.
speed species
max type
class name
bird falcon 389.0 fly
parrot 24.0 fly
mammal lion 80.5 run
monkey NaN jump
We would get the following output using the reset_index()
method.
print(df.reset_index(level="class"))
class speed species
max type
name
falcon bird 389.0 fly
parrot bird 24.0 fly
lion mammal 80.5 run
monkey mammal NaN jump
The reset_index()
method would reset the columns’ indexes through which the hierarchical levels are diluted and converted into a single-level column dataframe. The output to the above code is given as follows.
So in this tutorial, we have learned how to convert a MultiIndex column back to a single level column without bugs and easily without confusion.