Pandas DataFrame 제거 인덱스
Suraj Joshi
2023년1월30일
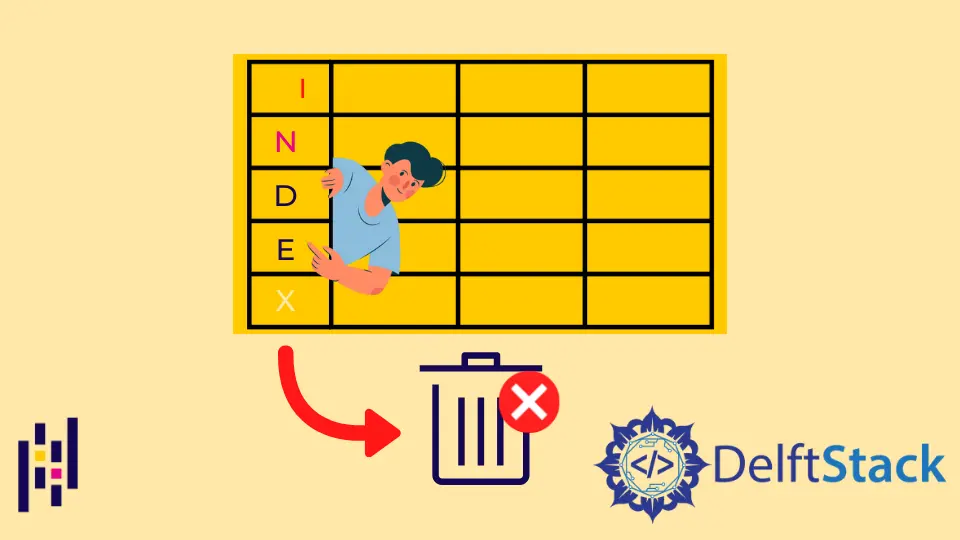
이 튜토리얼은 Pandas DataFrame의 인덱스를 제거하는 방법을 설명합니다.
아래에 표시된 DataFrame을 사용하여 색인을 제거하는 방법을 보여 드리겠습니다.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
print(my_df)
출력:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
reset_index()
메서드를 사용하여 Pandas DataFrame의 인덱스 제거
pandas.DataFrame.reset_index()
는 DataFrame의 색인을 기본 색인으로 재설정합니다.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.reset_index()
print("Before reseting Index:")
print(my_df, "\n")
print("After reseting Index:")
print(df_reset)
출력:
Before reseting Index:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After reseting Index:
index Person City Mother Tongue Age
0 A Alice Berlin German 37
1 B Steven Montreal French 20
2 C Neesham Toronto English 38
3 D Chris Rome Italian 23
4 E Alice Munich German 35
my_df
DataFrame의 색인을 재설정하지만 색인은 이제index
열로 표시됩니다. index
열을 삭제하려면reset_index()
메소드에서drop = True
를 설정할 수 있습니다.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.reset_index(drop=True)
print("Before reseting Index:")
print(my_df, "\n")
print("After reseting Index:")
print(df_reset)
출력:
Before reseting Index:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After reseting Index:
Person City Mother Tongue Age
0 Alice Berlin German 37
1 Steven Montreal French 20
2 Neesham Toronto English 38
3 Chris Rome Italian 23
4 Alice Munich German 35
set_index()
메서드를 사용하여 Pandas DataFrame의 인덱스 제거
pandas.DataFrame.set_index()
는 인수로 전달 된 열을 초기 색인을 재정의하는 DataFrame의 색인으로 설정합니다.
import pandas as pd
my_df = pd.DataFrame(
{
"Person": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"City": ["Berlin", "Montreal", "Toronto", "Rome", "Munich"],
"Mother Tongue": ["German", "French", "English", "Italian", "German"],
"Age": [37, 20, 38, 23, 35],
},
index=["A", "B", "C", "D", "E"],
)
df_reset = my_df.set_index("Person")
print("Initial DataFrame:")
print(my_df, "\n")
print("After setting Person column as Index:")
print(df_reset)
출력:
Initial DataFrame:
Person City Mother Tongue Age
A Alice Berlin German 37
B Steven Montreal French 20
C Neesham Toronto English 38
D Chris Rome Italian 23
E Alice Munich German 35
After setting Person column as Index:
City Mother Tongue Age
Person
Alice Berlin German 37
Steven Montreal French 20
Neesham Toronto English 38
Chris Rome Italian 23
Alice Munich German 35
Person
열을 DataFrame의 초기 색인을 재정의하는 my_df
DataFrame의 색인으로 설정합니다.
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn