Pandas DataFrame 재설정 색인
Suraj Joshi
2023년1월30일
Pandas
Pandas Index
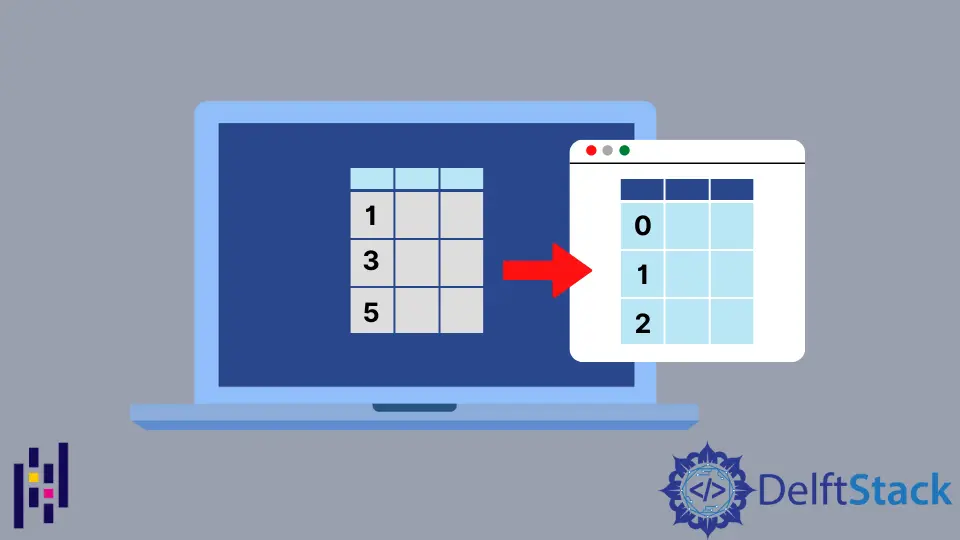
이 가이드에서는 pandas.DataFrame.reset_index()
를 사용하여 Pandas DataFrame에서 색인을 재설정하는 방법을 설명합니다. reset_index()
메소드는 DataFrame의 색인을 0
에서 (DataFrame의 행 수 - 1)
범위의 숫자로 기본 색인으로 설정합니다.
Pandas DataFrame reset_index()
메서드
통사론
DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill="")
pandas.DataFrame.reset_index()
메서드를 사용하여 DataFrame의 인덱스 재설정
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
출력:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
출력에 표시된대로 5 개의 행과 4 개의 열이있는 DataFrame이 있다고 가정합니다. 또한 DataFrame에 인덱스 세트가 있습니다.
DataFrame의 초기 인덱스를 열로 유지하는 DataFrame의 인덱스 재설정
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=False)
print(student_df)
출력:
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
index Name Age City Grade
0 501 Alice 17 New York A
1 502 Steven 20 Portland B-
2 503 Neesham 18 Boston B+
3 504 Chris 21 Seattle A-
4 505 Alice 15 Austin A
DataFrame student_df
의 색인을 기본 색인으로 재설정합니다. inplace=True
는 원래 DataFrame 자체를 변경합니다. drop=False
를 사용하면reset_index()
메소드를 사용한 후 초기 인덱스가 DataFrame에 열로 배치됩니다.
DataFrame의 인덱스 재설정 DataFrame의 초기 인덱스 제거
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=True)
print(student_df)
출력:
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Neesham 18 Boston B+
3 Chris 21 Seattle A-
4 Alice 15 Austin A
DataFrame student_df
의 색인을 기본 색인으로 재설정합니다. reset_index()
메소드에서drop=True
를 설정 했으므로 초기 인덱스는 DataFrame에서 삭제됩니다.
행 삭제 후 DataFrame의 인덱스 재설정
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
}
)
student_df.drop([2, 3], inplace=True)
print("Initial DataFrame:")
print(student_df)
print("")
student_df.reset_index(inplace=True, drop=True)
print("DataFrame after reset_index:")
print(student_df)
출력:
Initial DataFrame:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
4 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Alice 15 Austin A
출력에서 볼 수 있듯이 행을 삭제 한 후 인덱스가 누락되었습니다. 이 경우reset_index()
메소드를 사용하여 누락 된 값없이 색인을 사용할 수 있습니다.
초기 인덱스를 DataFrame의 열로 배치하려면reset_index()
메서드에서drop=False
를 사용할 수 있습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn