Pandas DataFrame 重置索引
Suraj Joshi
2023年1月30日
Pandas
Pandas Index
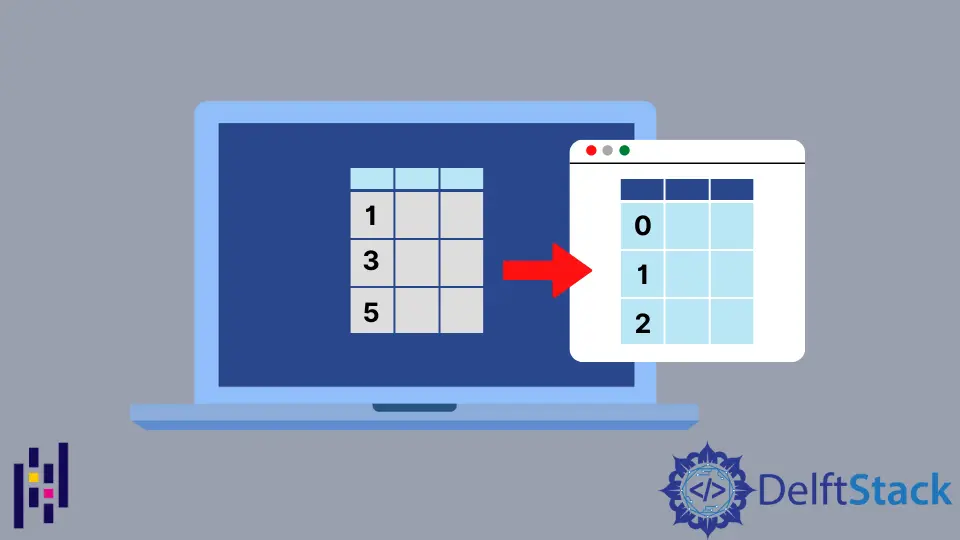
本教程介紹瞭如何使用 pandas.DataFrame.reset_index()
來重置 Pandas DataFrame 中的索引。reset_index()
方法將 DataFrame 的索引設定為預設索引,數字範圍從 0
到 (DataFrame 中的行數-1)
。
Pandas DataFrame reset_index()
方法
語法
DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill="")
使用 pandas.DataFrame.reset_index()
方法重置 DataFrame 的索引
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
輸出:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
假設我們有一個 DataFrame,有 5 行 4 列,如輸出所示。我們在 DataFrame 中還設定了一個索引。
重置 DataFrame 的索引,保持 DataFrame 的初始索引為列
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=False)
print(student_df)
輸出:
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
index Name Age City Grade
0 501 Alice 17 New York A
1 502 Steven 20 Portland B-
2 503 Neesham 18 Boston B+
3 504 Chris 21 Seattle A-
4 505 Alice 15 Austin A
它將 DataFrame student_df
的索引重置為預設索引。inplace=True
會在原 DataFrame 本身進行更改,如果我們使用 drop=False
,初始索引會被放置在 DataFrame 中作為列。如果我們使用 drop=False
,在使用 reset_index()
方法後,初始索引會被放置在 DataFrame 中作為一列。
重置 DataFrame 的索引,刪除 DataFrame 的初始索引
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=True)
print(student_df)
輸出:
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Neesham 18 Boston B+
3 Chris 21 Seattle A-
4 Alice 15 Austin A
它將 DataFrame student_df
的索引重置為預設索引。由於我們在 reset_index()
方法中設定了 drop=True
,初始索引從 DataFrame 中被刪除。
刪除行後重置 DataFrame 的索引
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
}
)
student_df.drop([2, 3], inplace=True)
print("Initial DataFrame:")
print(student_df)
print("")
student_df.reset_index(inplace=True, drop=True)
print("DataFrame after reset_index:")
print(student_df)
輸出:
Initial DataFrame:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
4 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Alice 15 Austin A
正如我們在輸出中所看到的,我們在刪除行後有缺失的索引。在這種情況下,我們可以使用 reset_index()
方法來使用沒有缺失值的索引。
如果我們希望將初始索引作為 DataFrame 的列,我們可以在 reset_index()
方法中使用 drop=False
。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn