Pandas DataFrame restablecer índice
-
Método Pandas DataFrame
reset_index()
-
Restablecer el índice de un DataFrame usando el método
pandas.DataFrame.reset_index()
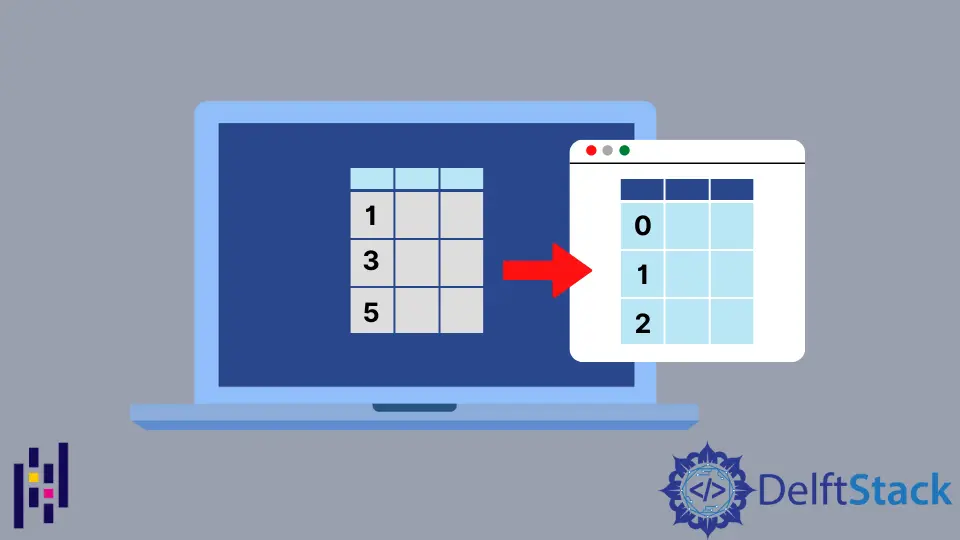
Este tutorial explica cómo podemos restablecer el índice en Pandas DataFrame utilizando pandas.DataFrame.reset_index()
. El método reset_index()
establece el índice del DataFrame al índice por defecto con números que van desde 0
hasta (número de filas en el DataFrame-1)
.
Método Pandas DataFrame reset_index()
Sintaxis
DataFrame.reset_index(level=None, drop=False, inplace=False, col_level=0, col_fill="")
Restablecer el índice de un DataFrame usando el método pandas.DataFrame.reset_index()
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
Resultado:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Supongamos que tenemos un DataFrame con cinco filas y cuatro columnas como se muestra en la salida. También tenemos un índice establecido en el DataFrame.
Restablecer el índice de un DataFrame manteniendo el índice inicial del DataFrame como columna
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=False)
print(student_df)
Producción :
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
index Name Age City Grade
0 501 Alice 17 New York A
1 502 Steven 20 Portland B-
2 503 Neesham 18 Boston B+
3 504 Chris 21 Seattle A-
4 505 Alice 15 Austin A
Restablece el índice del DataFrame student_df
al índice por defecto. El inplace=True
hace el cambio en el propio DataFrame original. Si utilizamos drop=False
, el índice inicial se coloca como una columna en el DataFrame después de utilizar el método reset_index()
.
Restablecer el índice de un DataFrame Eliminando el índice inicial del DataFrame
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("Initial DataFrame:")
print(student_df)
print("")
print("DataFrame after reset_index:")
student_df.reset_index(inplace=True, drop=True)
print(student_df)
Producción :
Initial DataFrame:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Neesham 18 Boston B+
3 Chris 21 Seattle A-
4 Alice 15 Austin A
Restablece el índice del DataFrame student_df
al índice por defecto. Como hemos establecido drop=True
en el método reset_index()
, se elimina el índice inicial del DataFrame.
Restablecer el índice de un DataFrame después de eliminar filas
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
}
)
student_df.drop([2, 3], inplace=True)
print("Initial DataFrame:")
print(student_df)
print("")
student_df.reset_index(inplace=True, drop=True)
print("DataFrame after reset_index:")
print(student_df)
Resultado:
Initial DataFrame:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
4 Alice 15 Austin A
DataFrame after reset_index:
Name Age City Grade
0 Alice 17 New York A
1 Steven 20 Portland B-
2 Alice 15 Austin A
Como podemos ver en la salida, tenemos índices perdidos después de borrar filas. En estos casos, podemos utilizar el método reset_index()
para utilizar el índice sin valores perdidos.
Si queremos que el índice inicial se coloque como la columna del DataFrame, podemos utilizar drop=False
en el método reset_index()
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn