How to Select the Values of One Property on All Objects of an Array in PowerShell
-
Use
%{$_.Property}
to Select the Values of One Property on All Objects of an Array in PowerShell -
Use
Select-Object
to Select the Values of One Property on All Objects of an Array in PowerShell -
Use
$array.Property
to Select the Values of One Property on All Objects of an Array in PowerShell -
Use
foreach
Statement to Select the Values of One Property on All Objects of an Array in PowerShell
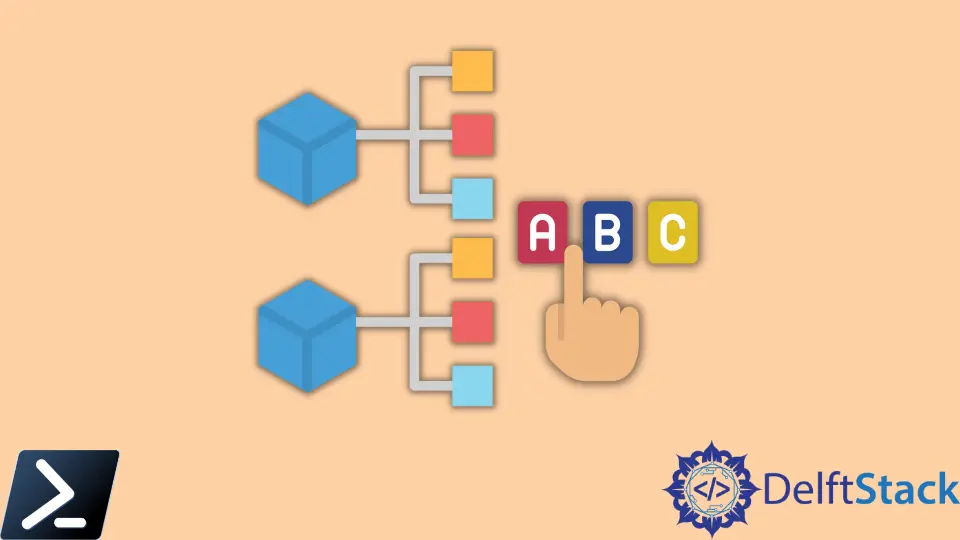
Arrays are the collection of values or objects. Most programming languages have arrays as the fundamental feature. An array is simply a data structure that contains a group of multiple items. An item can be an integer, a string, an object, or an array itself. A single array can include any combination of these items. Each item is stored in an index, always starting from 0
. The first item of an array is stored in the index 0
, the second item in 1
, the third item in 2
, and so on.
You can create an array in PowerShell using @()
.
$data = @()
The above command creates an empty array because we have not given any values.
Here is an example of creating an array $data
containing objects in the Name and Age property.
$data = @(
[pscustomobject]@{Name = 'James'; Age = '21' }
[pscustomobject]@{Name = 'David'; Age = '30' }
)
We have assigned different values to the Name
and Age
property. This tutorial will teach you to select the values of one property on all objects of an array in PowerShell.
Use %{$_.Property}
to Select the Values of One Property on All Objects of an Array in PowerShell
This is one of the easy method to select the values of one property on all objects of an array in PowerShell.
$select = @($data | % { $_.Name })
$select
Output:
James
David
Or, you can use only %Name
like this:
$data | % Name
Output:
James
David
Use Select-Object
to Select the Values of One Property on All Objects of an Array in PowerShell
The Select-Object
cmdlet selects specified properties of an object or set of objects. It helps select unique objects, a specified number of objects, or objects in a specified position in an array. Thus, you can use Select-Object
to select the values of one property on all objects of an array in PowerShell.
$data | Select-Object -ExpandProperty Name
Output:
James
David
Use $array.Property
to Select the Values of One Property on All Objects of an Array in PowerShell
This is the simplest method to select the values of one property on all objects of an array in PowerShell.
$data.Age
Output:
21
30
Use foreach
Statement to Select the Values of One Property on All Objects of an Array in PowerShell
The ForEach
statement is also known as the foreach
loop. It is used to traverse all the items in a collection of items. It is a language command used for looping through a series of values in an array or a collection of objects. You can run one or more commands against each item in an array within a ForEach
loop.
$data.foreach('Age')
Output:
21
30
Or, you can run the command like this.
$data.foreach({ $_.Age })
Output:
21
30
Alternatively, you can also use the below foreach
command to select the values of one property on all objects of an array in PowerShell.
foreach ($object in $data) { $object.Age }
Output:
21
30