How to Add Objects to an Array of Objects in PowerShell
-
Use the
+=
Operator to Add Objects to an Array of Objects in PowerShell -
PowerShell Add Object to Array Using the
+=
Operator With an Array -
Using the
+=
Operator With anArrayList
-
Using the
Add
Method With anArrayList
- Conclusion
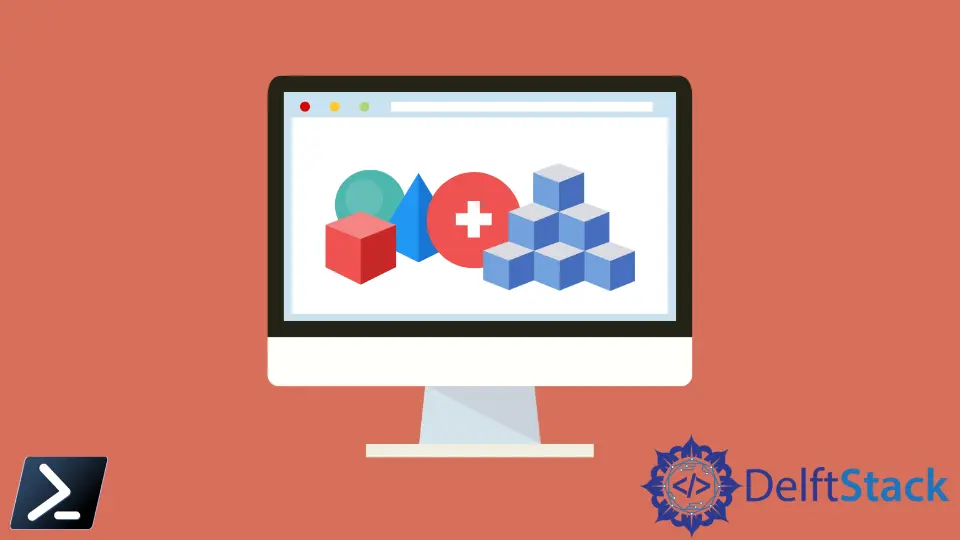
PowerShell, with its versatile scripting capabilities, allows users to manipulate arrays of objects efficiently. Adding objects to an array is a common operation, and there are various methods to achieve this.
Arrays are a data structure that stores a collection of multiple items.
Arrays can contain one or more items in PowerShell. The items can be the same or different types.
They can be a string, an integer, an object, or even another array. A single array can have any combination of these items.
Each item is stored in the index number, which starts at zero. The first item is stored at 0
, the second at 1
, the third at 2
, etc.
An array of objects is a collection of objects. This tutorial will teach you to add objects to an array of objects in PowerShell.
Here is an example of creating an array $data
containing objects in Name
and Age
.
$data = @(
[pscustomobject]@{Name = 'Rohan'; Age = '21' }
[pscustomobject]@{Name = 'John'; Age = '30' }
)
Use the +=
Operator to Add Objects to an Array of Objects in PowerShell
The +=
operator is a straightforward way to add elements to an array in PowerShell. It works by creating a new array with the additional element(s).
The plus-equals +=
is used to add items to an array. Every time you use it, it duplicates and creates a new array.
You can use the +=
to add objects to an array of objects in PowerShell.
The following example adds an array of objects $data
.
$data += [pscustomobject]@{Name = 'Sam'; Age = '26' }
Now, check the elements of $data
.
$data
Output:
Name Age
---- ---
Rohan 21
John 30
Sam 26
You can access the objects from an array by enclosing the index number in brackets.
For example:
$data[2]
Output:
Name Age
---- ---
Sam 26
As shown below, the individual objects can be accessed by specifying the property.
$data[2].Name
Output:
Sam
PowerShell Add Object to Array Using the +=
Operator With an Array
To add multiple elements to an array, the +=
operator can be combined with another array.
Example Code:
# Existing array
$array = @(1, 2, 3)
# Array of new elements
$newElements = @(4, 5, 6)
# Add new elements to the existing array
$array += $newElements
# Display the updated array
$array
Output:
1
2
3
4
5
6
In this example, we have an existing array $array
with elements 1
, 2
, and 3
. We create a new array, $newElements
, with elements 4
, 5
, and 6
.
By using the +=
operator, we add the new elements to the existing array, resulting in an updated array containing 1
, 2
, 3
, 4
, 5
, and 6
.
Using the +=
Operator With an ArrayList
The ArrayList
class in PowerShell provides a dynamic array that can be modified efficiently.
Example Code:
# Using an ArrayList
$arrayList = New-Object System.Collections.ArrayList
$arrayList.AddRange(@(1, 2, 3))
# Add new elements
$arrayList += 4
$arrayList += 5
$arrayList += 6
# Display the updated ArrayList
$arrayList
Output:
1
2
3
4
5
6
In this example, we create an ArrayList
named $arrayList
and add initial elements 1
, 2
, and 3
using the AddRange
method.
We then use the +=
operator to add new elements 4
, 5
, and 6
to the ArrayList
. The result is an updated ArrayList
containing all the added elements.
ArrayList
can be more efficient than the simple array +=
approach, especially for larger datasets.Using the Add
Method With an ArrayList
The Add
method of an ArrayList
allows for adding individual elements, making it useful for dynamic updates.
Example Code:
# Using an ArrayList
$arrayList = New-Object System.Collections.ArrayList
$arrayList.AddRange(@(1, 2, 3))
# Add new elements using the Add method
$arrayList.Add(4)
$arrayList.Add(5)
$arrayList.Add(6)
# Display the updated ArrayList
$arrayList
Output:
3
4
5
1
2
3
4
5
6
In this example, we create an ArrayList
named $arrayList
and add initial elements 1
, 2
, and 3
using the AddRange
method.
We then use the Add
method to individually add new elements 4
, 5
, and 6
to the ArrayList
. The result is an updated ArrayList
containing all the added elements.
Add
method is efficient and suitable for scenarios where elements are added individually.Conclusion
This article explores methods for adding objects to an array of objects in PowerShell. It covers the fundamentals of arrays in PowerShell, including their ability to store diverse data types and the use of index numbers for item retrieval.
The primary focus is on adding objects to arrays, and the article introduces various techniques:
- Using
+=
Operator: This straightforward method involves creating a new array with each addition, suitable for smaller datasets. - Combining Arrays with
+=
Operator: Demonstrates the use of+=
to combine existing arrays with new elements, providing flexibility for dynamic updates. - Using
ArrayList
: Introduces theArrayList
class for more efficient dynamic array manipulation, especially beneficial for larger datasets. - Using the
Add
Method withArrayList
: Illustrates the use of theAdd
method for individually adding elements to anArrayList
, emphasizing efficiency.
The examples provided showcase the flexibility of PowerShell in managing arrays of objects, and the article emphasizes the importance of selecting the most appropriate method based on performance, simplicity, and type safety considerations. Understanding these methods equips PowerShell users to make informed decisions in array manipulation for various scripting and automation tasks.