How to Add Items to Array in the PowerShell
- Create Arrays in PowerShell
-
Add Items to an Array in PowerShell Using the
+=
Operator -
Add Items to an Array in PowerShell Using the
ArrayList.Add()
Method - Conclusion
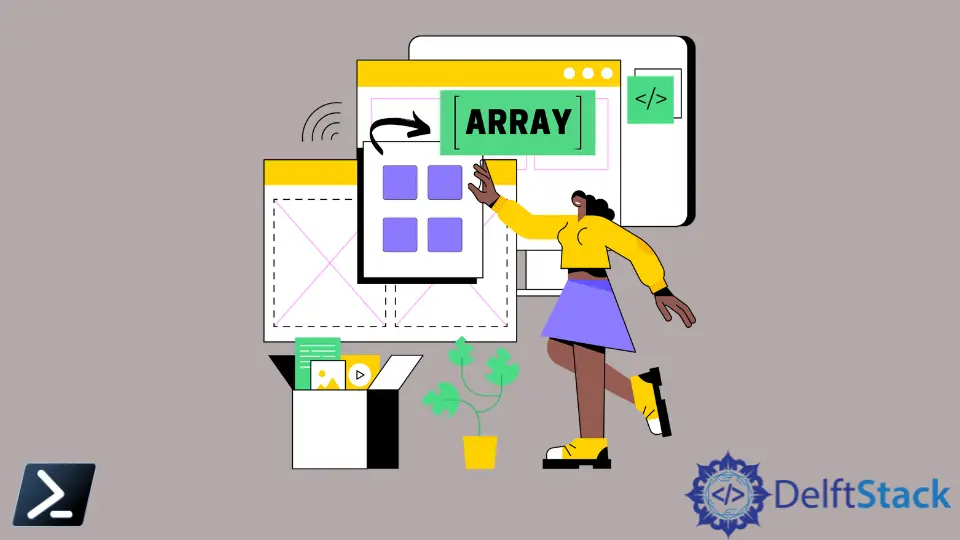
PowerShell, Microsoft’s powerful scripting language and command-line shell, provides a versatile array system for managing collections of data. Arrays are essential for storing, retrieving, and manipulating data in PowerShell scripts.
In this comprehensive article, we will explore various methods to add items to arrays in PowerShell.
Create Arrays in PowerShell
An array is a data structure that allows you to store a collection of elements in a single variable. In PowerShell, arrays can hold different types of data, such as strings, numbers, or objects.
They provide a convenient way to manage and manipulate sets of related information.
Before going into the details of adding items to an array, it’s essential to understand the basics of arrays in PowerShell. Arrays can be created using the @()
syntax, enclosing the elements within parentheses.
Here’s a simple example:
# Creating an array with three elements
$myArray = @(1, 2, 3)
Add Items to an Array in PowerShell Using the +=
Operator
One of the simplest ways to add an item to an array is by using the +=
operator. The +=
operator in PowerShell is used for concatenation or addition, depending on the data type.
When it comes to arrays, the +=
operator is used to append items to an existing array. This is particularly useful when you want to dynamically build an array by adding elements one at a time.
The syntax is straightforward:
$Array += "NewItem"
Here, $Array
represents the existing array, and "NewItem"
is the item you want to add.
Let’s go through some examples of adding items to an array using the +=
operator.
Example 1: Adding a Single Item to an Array
$Days = "Monday", "Tuesday", "Wednesday"
$Days += "Thursday"
$Days
In this example, we have an array named $Days
initially containing three days.
Using $Days += "Thursday"
, we add a new day to the array. The output will display all four days.
Output:
Monday
Tuesday
Wednesday
Thursday
Example 2: Adding Multiple Items to an Array
$Fruits = "Apple", "Banana"
$Fruits += "Orange", "Grapes"
$Fruits
Here, the array $Fruits
starts with two items.
By using $Fruits += "Orange", "Grapes"
, we add two more fruits to the array. The output will show all four fruits.
Output:
Apple
Banana
Orange
Grapes
Example 3: Adding an Array to Another Array
$Array1 = 1, 2, 3
$Array2 = 4, 5, 6
$Array1 += $Array2
$Array1
In this example, we have two arrays, $Array1
and $Array2
.
By using $Array1 += $Array2
, we concatenate the contents of $Array2
to $Array1
. The output will display all six numbers.
Output:
1
2
3
4
5
6
The +=
operator is a powerful and concise way to add items to arrays in PowerShell. It enables dynamic expansion, making it a preferred choice when dealing with evolving data structures.
Add Items to an Array in PowerShell Using the ArrayList.Add()
Method
While the +=
operator is convenient, it’s essential to understand its implications, especially when dealing with large arrays. The +=
operator creates a new array each time an item is added, copying the existing elements and appending the new one.
This process can be resource-intensive for extensive arrays, impacting performance.
For scenarios where performance is crucial, consider using the ArrayList.Add()
method provided by the System.Collections.ArrayList
class. Unlike traditional arrays, an ArrayList
dynamically adjusts its size, making it a more efficient choice for scenarios involving frequent additions or removals of elements.
The syntax of the ArrayList.Add()
method is as follows:
$ArrayList.Add($NewItem)
Here, $ArrayList
is the existing ArrayList
, and $NewItem
represents the item to be added.
Let’s walk through a few examples:
Example 1: Adding Multiple Items
$DaysList = New-Object System.Collections.ArrayList
$DaysList.Add("Monday")
$DaysList.Add("Tuesday")
$DaysList.Add("Wednesday")
$DaysList.Add("Thursday")
$DaysList
In this example, an ArrayList
named $DaysList
is created using New-Object
.
The Add()
method is then utilized to add the strings "Monday"
, "Tuesday"
, "Wednesday"
, and "Thursday"
to the ArrayList
. The resulting ArrayList
is printed.
Output:
Monday
Tuesday
Wednesday
Thursday
Example 2: Adding an Array to Another ArrayList
$FruitsList = New-Object System.Collections.ArrayList
$FruitsList.AddRange(@("Orange", "Grapes"))
$FruitsList.Add("Apple")
$FruitsList.Add("Banana")
$FruitsList
In this instance, an ArrayList
$FruitsList
is established.
The Add()
method is employed to add both individual elements and an array ("Orange", "Grapes")
to the ArrayList
. The resulting ArrayList
is then printed.
Output:
Orange
Grapes
Apple
Banana
Unlike the +=
operator, the ArrayList.Add()
method appends items to the existing array without creating a new one each time. This approach minimizes memory overhead and enhances script performance, particularly when dealing with large datasets.
Conclusion
In PowerShell scripting, the ability to manipulate arrays with precision is a fundamental skill. We’ve explored various techniques for adding items to arrays, each catering to different scenarios and requirements.
The +=
operator stands out as a simple and widely adopted approach. It seamlessly appends items to arrays, making it a suitable choice for regular arrays.
On the other hand, the ArrayList.Add()
method extends a helping hand when dealing with dynamic arrays. Its ability to resize arrays dynamically proves invaluable when working with collections of varying sizes.
It’s important to be mindful of the limitations, such as the fixed size of regular arrays, which may result in errors when attempting to use methods like Array.Add()
. This emphasizes the importance of choosing the right method based on the nature of the array you are working with.