How to Create an Array, Hash Table, and Dictionary in PowerShell
-
Use
@()
to Create an Array in PowerShell -
Use the Index Number in Brackets
[]
Individual Access Items in an Array PowerShell -
Create a
Hash table
in PowerShell -
Create an
ordered dictionary
in PowerShell
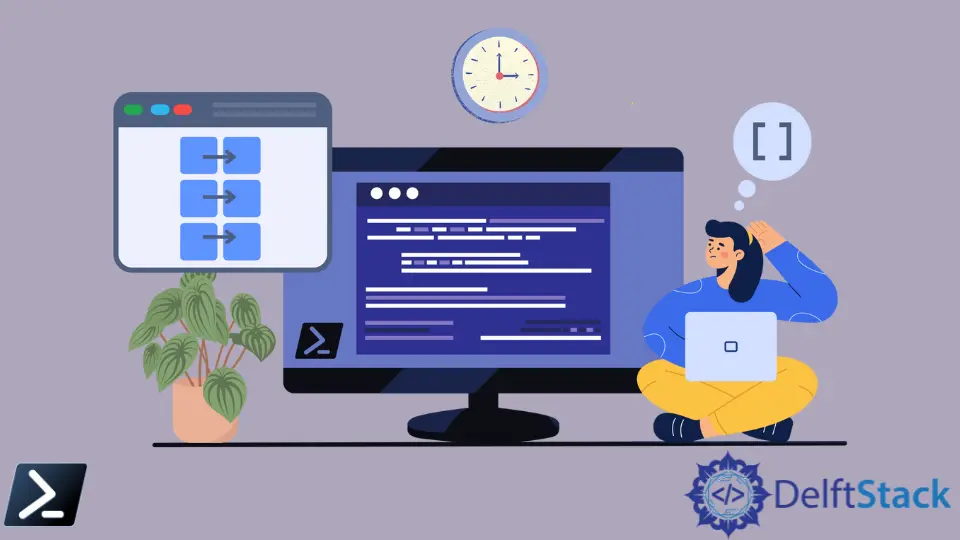
Arrays are the collection of values or objects. Most programming languages have arrays as the fundamental feature.
In PowerShell, an array is simply a data structure that serves as a collection of multiple items.
Use @()
to Create an Array in PowerShell
The items can be the same or different types. The proper way to create an array in PowerShell is by using @()
.
The items of an array are placed in the @()
parentheses. An empty array will be created when no values are placed in ()
.
The following command creates an array $data
with 3 items.
$data = @('apple', 'ball', 'cat')
Call the array variable to see items in it.
$data
Output:
apple
ball
cat
You can use the GetType
method to get the data type of a variable.
$data.GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True Object[] System.Array
Use the Index Number in Brackets []
Individual Access Items in an Array PowerShell
You can access individual items in an array by using the index number in brackets []
. The items are stored in the index number in ascending integer order starting from zero.
To get the first item in an array, you have to use [0]
:
$data[0]
Output:
apple
Create a Hash table
in PowerShell
A hash table is a compact data structure that stores each value using a key. It is also known as dictionary
or associative array
.
It is a simple key/value store. For example, a hash table might contain students’ names and roll numbers, where the students’ names are the keys and the roll numbers are values, or vice-versa.
You can create a hash table in PowerShell by using @{}
. The keys and values are added in the {}
brackets.
The keys and values in hash tables are .NET objects
. They can have any object type.
The following command creates a hash table $hash
with three keys and values.
$hash = @{Fruit = "Apple"; Color = "Red"; Count = 5 }
A hash table is displayed in the tabular format having one column for keys and another for values.
$hash
Output:
Name Value
---- -----
Fruit Apple
Color Red
Count 5
Get the data type of $hash
.
$hash.GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True Hashtable System.Object
Hash tables have Keys
and Values
properties. You can access the items of a hash table by using the Key
name as an index.
$hash['Color']
Output:
Red
Or, you can use the dot notation to display values in keys.
$hash.Count
Output:
5
Create an ordered dictionary
in PowerShell
You can create an ordered dictionary using the [ordered]
attribute. It would help to place the attribute before the @
symbol.
$dict = [ordered]@{Fruit = "Apple"; Color = "Red"; Count = 5 }
Ordered dictionaries can be used in the same way as a hash table.
$dict
Output:
Name Value
---- -----
Fruit Apple
Color Red
Count 5
The keys in an ordered dictionary always appear. But there is no such order of keys in a hash table.
We hope this article helps you understand how to create an array, hash table, and ordered dictionary in PowerShell.