How to Count Objects in PowerShell
-
Use the
Count
Method in PowerShell to Count Objects -
Count in PowerShell Using the
Measure-Object
Command - Conclusion
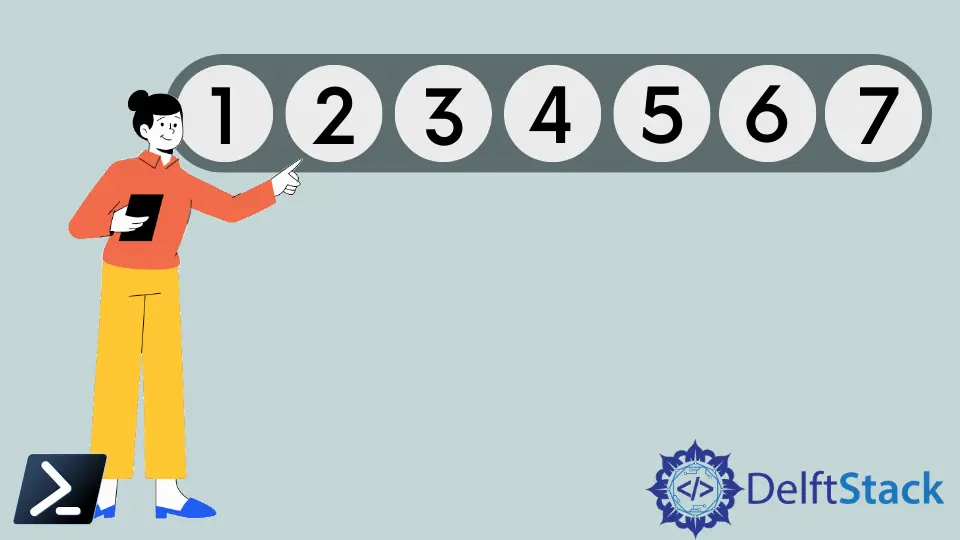
PowerShell, with its vast array of operators and cmdlets, offers an efficient and flexible way to manage and manipulate data.
Among these operators, the Count
operator and the Measure-Object
cmdlet are powerful tools that allow you to quickly determine the number of objects in an array or collection, as well as calculate various properties of those objects.
By leveraging these features, you can effortlessly retrieve counts and other valuable statistics, making PowerShell a versatile tool for data analysis and management.
This article delves into practical applications of both the Count
operator and the Measure-Object
cmdlet by demonstrating how to count files in a folder and perform other essential counting tasks using PowerShell.
Whether you’re a system administrator looking to manage your resources or a developer seeking to automate data-related tasks, understanding how to count and measure objects is a valuable skill in your PowerShell toolkit.
Use the Count
Method in PowerShell to Count Objects
Syntax:
(PSObject).Count
We can access the PowerShell Count
operator by wrapping the object in parentheses (()
). Then, add a period (.
), followed by the count.
Use the Count
Operator to Count Files
Whether you’re a system administrator or a developer, there are scenarios where you need to count files within a directory. PowerShell simplifies this task with the Count
operator, allowing you to quickly determine the number of files in a folder. In this article, we will explore how to count files in a folder using the Count
operator, step by step.
Step 1: Specify the Folder Path
First, we need to specify the path to the folder we want to count files in. Replace "C:\YourFolderPath"
with the actual path of your folder.
$folderPath = "C:\YourFolderPath"
Step 2: Retrieve the Files
Next, we’ll use the Get-ChildItem
cmdlet to retrieve the files within the specified folder. The -File
parameter ensures that only files (not directories) are retrieved.
$files = Get-ChildItem -Path $folderPath -File
Step 3: Count the Files
Now that we have retrieved the files, we can use the Count
operator to determine the number of files.
$fileCount = $files.Count
Step 4: Display the Result
Finally, we can display the file count to the user or use it in our script as needed.
Write-Host "Total files in $folderPath: $fileCount"
Complete PowerShell Script
Here’s the complete PowerShell script for counting files in a folder:
# Step 1: Specify the Folder Path
$folderPath = "C:\YourFolderPath"
# Step 2: Retrieve the Files
$files = Get-ChildItem -Path $folderPath -File
# Step 3: Count the Files
$fileCount = $files.Count
# Step 4: Display the Result
Write-Host "Total files in $folderPath: $fileCount"
Use the Count
Operator to Count Files Recursively (including Subfolder) in A Folder
What if we also need to count all files in the folder and its subfolders?
Below is the complete code.
# Define the folder path
$folderPath = "C:\YourFolderPath"
# Count the files in the folder and subfolders
$fileCount = (Get-ChildItem -Path $folderPath -File -Recurse).Count
# Display the result
Write-Host "Total files in the folder and subfolders: $fileCount"
Now, let’s break down the code and explain each part in detail:
1. Count the Files in the Folder and Subfolders
# Count the files in the folder and subfolders
$fileCount = (Get-ChildItem -Path $folderPath -File -Recurse).Count
Here, we use the Get-ChildItem
cmdlet to retrieve all files in the specified folder and its subfolders (-Recurse
). The -File
parameter ensures that only files are included in the result, excluding directories.
We wrap this command in parentheses ( ... )
to immediately apply the Count
operator to the result. This operator counts the elements in the collection, giving us the total file count.
Use the Count
Operator to Count Objects in An Array
Counting objects in an array is a common task in PowerShell, and it’s quite straightforward thanks to the Count
operator.
This operator allows you to quickly determine the number of elements in an array or collection. We’ll introduce how to count objects in a string array using the Count
operator.
It works with various data types, including string arrays. Here’s how you can use it:
# Create a string array
$stringArray = "Item1", "Item2", "Item3", "Item4"
# Count the objects in the array
$count = $stringArray.Count
# Display the result
Write-Host "Total items in the array: $count"
In the above code, we first create a string array named $stringArray
, and then we use the Count
operator to determine the number of items in the array. Finally, we display the count using Write-Host
.
Count in PowerShell Using the Measure-Object
Command
PowerShell’s Measure-Object
cmdlet, often referred to as Measure
, calculates various properties of objects, including the count of items.
Count Files in A Folder Using the Measure-Object
cmdlet
To count files in a folder, we’ll utilize the Measure-Object
cmdlet in combination with other PowerShell commands.
Here’s a step-by-step guide on counting files in a folder using Measure-Object
:
Step 1: Get the Files
To count the files, use the Get-ChildItem
cmdlet, which retrieves a list of files and folders in the specified directory. By default, it includes subfolders as well. Here’s the command:
$fileList = Get-ChildItem -Path $folderPath -File
In this command:
$fileList
contains the file objects.Measure-Object
calculates properties of the objects..Count
retrieves the count of objects.
Now, the variable $fileCount
holds the number of files in the specified folder.
Example Code
Here’s the complete PowerShell script:
# Step 1: Define the folder path
$folderPath = "C:\YourFolderPath"
# Step 2: Get the files
$fileList = Get-ChildItem -Path $folderPath -File
# Step 3: Measure the object count
$fileCount = ($fileList | Measure-Object).Count
# Step 4: Display the result
Write-Host "Number of files in $folderPath: $fileCount"
Replace "C:\YourFolderPath"
with the path to your folder, and run the script to count the files.
Counting Files in Subfolders
If you want to count files in subfolders as well, you can modify the Get-ChildItem
command. By using the -Recurse
parameter, you instruct PowerShell to include subdirectories. Here’s how:
$fileList = Get-ChildItem -Path $folderPath -File -Recurse
This single modification extends the counting to all files in the specified folder and its subfolders.
Using Measure-Object for Line Count in A File
The Measure-Object
cmdlet in PowerShell is a versatile tool for calculating properties of objects, including the number of lines in text files. To count lines in a text file, we’ll leverage the Get-Content
cmdlet in conjunction with Measure-Object
.
Here’s a step-by-step guide on counting lines in a text file using Measure-Object
:
Step 1: Read the File Content
To count the lines, use the Get-Content
cmdlet to read the file’s content. This cmdlet reads each line of the file and stores them in an array. Here’s the command:
$fileContent = Get-Content -Path $filePath
In this command:
-Path
specifies the path to the text file.$fileContent
stores the lines of the text file as an array.
Now, you have the contents of the text file loaded into the $fileContent
variable.
Step 2: Measure the Line Count
Utilize the Measure-Object
cmdlet to count the lines in the text file. Here’s the command:
$lineCount = ($fileContent | Measure-Object -Line).Lines
In this command:
$fileContent
contains the lines from the text file.Measure-Object -Line
calculates the number of lines..Lines
retrieves the line count.
Now, the variable $lineCount
holds the total number of lines in the text file.
Complete Example Code
Here’s the complete PowerShell script:
# Step 1: Define the file path
$filePath = "C:\YourFilePath\YourFile.txt"
# Step 2: Read the file content
$fileContent = Get-Content -Path $filePath
# Step 3: Measure the line count
$lineCount = ($fileContent | Measure-Object -Line).Lines
# Step 4: Display the result
Write-Host "Number of lines in $filePath: $lineCount"
Replace "C:\YourFilePath\YourFile.txt"
with the path to your text file, and run the script to count the lines.
Using Measure-Object
for Process Count
The Measure-Object
cmdlet in PowerShell is not limited to counting lines or numbers. It can also count objects, making it a valuable tool for counting processes. We’ll use Get-Process
to retrieve the list of processes and then apply Measure-Object
to count them.
Here’s a step-by-step guide on counting processes using Measure-Object
:
Step 1: Retrieve the Process List
Start by using the Get-Process
cmdlet to obtain a list of running processes. Store this list in a variable for further analysis:
$processList = Get-Process
In this command:
$processList
stores the list of running processes.
Now, you have the list of processes available in the $processList
variable.
Step 2: Measure the Process Count
Next, apply the Measure-Object
cmdlet to count the processes in the list. Here’s the command:
$processCount = ($processList | Measure-Object).Count
In this command:
$processList
contains the list of processes.Measure-Object
calculates various properties of the objects in the list..Count
retrieves the count of objects.
The variable $processCount
now holds the total number of processes running on your system.
Step 3: Display the Result
To see the process count, display the value stored in the $processCount
variable:
Write-Host "Number of processes running: $processCount"
This command prints a message that includes the process count.
Complete Example Code
Here’s the complete PowerShell script:
# Step 1: Retrieve the process list
$processList = Get-Process
# Step 2: Measure the process count
$processCount = ($processList | Measure-Object).Count
# Step 3: Display the result
Write-Host "Number of processes running: $processCount"
Run the script to count the processes running on your system.
Using Measure-Object for Registry Key Count
When it comes to counting registry keys, we can leverage this cmdlet along with the Get-Item
cmdlet, which allows us to access registry keys as if they were file system paths.
Here’s a step-by-step guide on counting registry keys using Measure-Object
:
Step 1: Access the Registry Key
Start by using the Get-Item
cmdlet to access the specific registry key you want to count. You can specify the registry path as a string:
$registryPath = "HKLM:\Software\YourRegistryKeyPath"
$registryKey = Get-Item -LiteralPath $registryPath
In this command:
$registryPath
stores the path to the registry key you want to count.$registryKey
accesses the registry key.
Make sure to replace "HKLM:\Software\YourRegistryKeyPath"
with the actual registry key path you want to count.
Step 2: Measure the Registry Key Count
Apply the Measure-Object
cmdlet to count the registry keys in the specified path:
$keyCount = (Get-ChildItem -Path $registryKey.PSPath).Count
In this command:
(Get-ChildItem -Path $registryKey.PSPath)
retrieves the child items (registry keys) within the specified path..Count
retrieves the count of registry keys.
The variable $keyCount
now holds the total number of registry keys within the specified path.
Complete Example Code
Here’s the complete PowerShell script:
# Step 1: Access the registry key
$registryPath = "HKLM:\Software\YourRegistryKeyPath"
$registryKey = Get-Item -LiteralPath $registryPath
# Step 2: Measure the registry key count
$keyCount = (Get-ChildItem -Path $registryKey.PSPath).Count
# Step 3: Display the result
Write-Host "Number of registry keys: $keyCount"
Replace "HKLM:\Software\YourRegistryKeyPath"
with the actual path of the registry key you want to count, and run the script to count the registry keys.
Using Measure-Object to Count Items
When used with arrays, Measure-Object
efficiently counts the items within the array.
Here’s how to count items in an array using Measure-Object
:
Step 1: Create an Array
Start by defining an array that contains the items you want to count. You can manually create an array or use cmdlets or functions to populate it. For example, let’s create a simple array:
$myArray = 1, 2, 3, 4, 5
In this array, we have five items (numbers 1 to 5) that we want to count.
Step 2: Use Measure-Object
Apply the Measure-Object
cmdlet to count the items in the array:
$itemCount = ($myArray | Measure-Object).Count
In this command:
$myArray
is the array you want to count.Measure-Object
processes the array and provides information about it..Count
retrieves the count of items within the array.
The variable $itemCount
now holds the total number of items in the array.
Example Code
Here’s the complete PowerShell script:
# Step 1: Create an array
$myArray = 1, 2, 3, 4, 5
# Step 2: Use Measure-Object to count items
$itemCount = ($myArray | Measure-Object).Count
# Step 3: Display the result
Write-Host "Number of items in the array: $itemCount"
Run the script, and it will count the items in the array and display the count.
Using Measure-Object to Count Lines
To count lines in a string using Measure-Object
, follow these steps:
Step 1: Create a String
Start by defining a PowerShell string that contains the text you want to count lines for. This string can be manually created or obtained from various sources, such as files, user input, or web requests. Here’s an example string:
$text = @"
This is line 1.
This is line 2.
This is line 3.
"@
In this command:
$text
is the string you want to count lines for.Measure-Object
processes the string and provides line count information.-Line
specifies that we want to count lines..Lines
retrieves the count of lines from the measurement.
The variable $lineCount
now holds the total number of lines in the string.
Step 3: Display the Result
To see the count of lines, you can display the value stored in the $lineCount
variable:
Write-Host "Number of lines in the string: $lineCount"
This command prints a message that includes the line count of the string.
Example Code
Here’s the complete PowerShell script:
# Step 1: Create a string
$text = @"
This is line 1.
This is line 2.
This is line 3.
"@
# Step 2: Use Measure-Object to count lines
$lineCount = ($text | Measure-Object -Line).Lines
# Step 3: Display the result
Write-Host "Number of lines in the string: $lineCount"
Output:
Number of lines in the string: 3
Run the script, and it will count the lines in the string and display the line count.
Using Measure-Object to Count Characters
To count characters in a string using Measure-Object
, follow these steps:
Step 1: Create a String
Start by defining a PowerShell string that contains the text for which you want to count characters. This string can be manually created or obtained from various sources, such as user input or data files. Here’s an example string:
$text = "Hello, DelftStack!"
In this command:
$text
is the string you want to count characters for.Measure-Object
processes the string and provides character count information.-Character
specifies that we want to count characters..Characters
retrieves the count of characters from the measurement.
The variable $charCount
now holds the total number of characters in the string.
Step 3: Display the Result
To see the character count, you can display the value stored in the $charCount
variable:
Write-Host "Number of characters in the string: $charCount"
This command prints a message that includes the character count of the string.
Example Code
Here’s the complete PowerShell script:
# Step 1: Create a string
$text = "Hello, DelftStack!"
# Step 2: Use Measure-Object to count characters
$charCount = ($text | Measure-Object -Character).Characters
# Step 3: Display the result
Write-Host "Number of characters in the string: $charCount"
Output:
Number of characters in the string: 18
Running this script will count the characters in the string and display the character count.
Conclusion
In this article, we explored two essential tools in PowerShell: the Count
operator and the Measure-Object
cmdlet.
These tools empower you to efficiently count and measure objects within arrays or collections, making PowerShell a versatile solution for data analysis and management.
By following the step-by-step instructions and examples provided in this article, you can confidently apply both the Count
operator and the Measure-Object
cmdlet in your PowerShell scripts.
Whether you’re working with files, registry keys, processes, arrays, or strings, these tools are indispensable for your PowerShell scripting endeavors.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn