How to Determine if Two Objects Are Identical in PowerShell
-
Use the
Compare-Object
Cmdlet to Compare Two Strings in PowerShell -
Use the
Compare-Object
Cmdlet to ComparePSCustomObject
in PowerShell -
Use the
Compare-Object
Cmdlet to Compare Source and Destination Files in PowerShell -
Use the
Compare-Object
Cmdlet to Compare Properties of Files in PowerShell
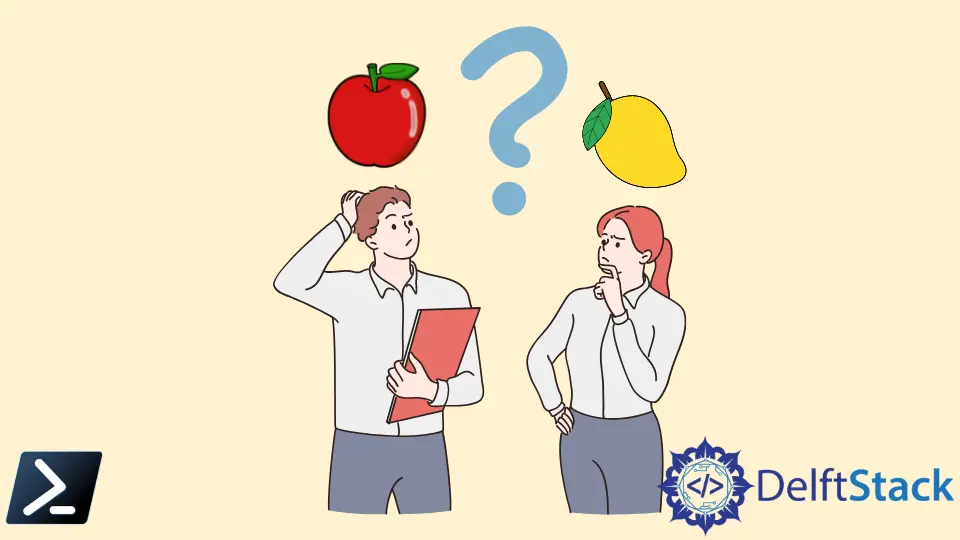
Sometimes, it can be challenging to compare two objects and tell if they are identical, but it can be easily done with the help of PowerShell. The objects can be of various types such as strings, files, files or variables content, processes, etc.
You can use the Compare-Object
cmdlet to compare two sets of objects in PowerShell. This tutorial will help you compare two objects and determine if they are identical using PowerShell.
Use the Compare-Object
Cmdlet to Compare Two Strings in PowerShell
You can use Compare-Object
to determine the differences between two strings.
Before getting started, let’s understand the side indicators used by the Compare-Object
cmdlet to show the differences between the two objects.
Side Indicators Meaning
== It indicates both the source and destination objects are equal or contain the same values.
=> It indicates that the destination object is different or the content only exists on the destination object.
<= It indicates that the source object is different or the content only exists on the source object.
The following command is an example of a comparison of two different strings.
Compare-Object "Apple" "Mango"
Output:
InputObject SideIndicator
----------- -------------
Mango =>
Apple <=
The above output shows that both the strings are different. The string Mango
shows the right indicator, which means it is different from the source object, and the string Apple
shows the left indicator, which means it is different from the destination object.
But if two strings are equal, the command does not show any output unless used with the -IncludeEqual
parameter. It shows the ==
indicator for equal strings.
The following command compares two similar strings.
Compare-Object "Apple" "apple" -IncludeEqual
Output:
InputObject SideIndicator
----------- -------------
Apple ==
The above example is not case sensitive as it displayed equal indicators although they have different letter cases. You will need to use the -CaseSensitive
parameter to compare case-sensitive strings.
Compare-Object "Apple" "apple" -CaseSensitive
Output:
InputObject SideIndicator
----------- -------------
apple =>
Apple <=
As you can see, it shows differences in the two strings this time.
Use the Compare-Object
Cmdlet to Compare PSCustomObject
in PowerShell
You can also use Compare-Object
to compare PSCustomObject
in PowerShell.
The following example demonstrates using Compare-Object
to compare two PSCustomObject
: $obj1
and $obj2
.
$obj1 = [PSCustomObject]@{ a = 10; b = 20 }
$obj2 = [PSCustomObject]@{ a = 10; b = 20 }
function Test-Object {
param(
[Parameter(Mandatory = $true)]
$obj1,
[Parameter(Mandatory = $false)]
$obj2
)
return !(Compare-Object $obj1.PSObject.Properties $obj2.PSObject.Properties)
}
Test-Object $obj1 $obj2
Output:
True
It returns True
, which means both objects have the same values and are identical.
Use the Compare-Object
Cmdlet to Compare Source and Destination Files in PowerShell
For this tutorial, we have created two folders, Folder1
and Folder2
, containing some .txt
files in the C
drive of the computer.
The following script compares files in the source folder Folder1
and the destination folder Folder2
.
$sourcefiles = Get-ChildItem C:\Folder1
$destinationfiles = Get-ChildItem C:\Folder2
Compare-Object $sourcefiles $destinationfiles -IncludeEqual
The Get-ChildItem
cmdlet is used to get the content from folders Folder1
and Folder2
, stored in two variables $sourcefiles
and $destinationfiles
, respectively.
Output:
InputObject SideIndicator
----------- -------------
Text3.txt ==
Text4.txt =>
Text1.txt <=
Text2.txt <=
The above output shows that Test3.txt
is present in both folders. The Test4.txt
is only present in the destination folder, whereas the Test1.txt
and Test2.txt
are only present in the source folder.
Use the Compare-Object
Cmdlet to Compare Properties of Files in PowerShell
You can also use Compare-Object
to compare files by properties like lastaccesstime
, lastwritetime
, length
, etc. The -Property
parameter is used to specify the properties of the objects to compare.
The following command compares files by properties Name
and Length
using Compare-Object
.
Compare-Object $sourcefiles $destinationfiles -Property Name, Length -IncludeEqual
Output:
Name Length SideIndicator
---- ------ -------------
Test3.txt 5 ==
Test4.txt 12 =>
Test1.txt 12 <=
Test2.txt 8 <=
The Compare-Object
is a simple and useful command to compare two objects. We hope this tutorial gave you an idea of how to check if two objects are equal using PowerShell.