How to Check the Beginning of a String Using PowerShell
-
Using the
-like
Logical Operator to Check the Beginning of a String in PowerShell -
Using the
-clike
Logical Operator to Check the Beginning of a String in PowerShell -
Using the
StartsWith()
Function to Check the Beginning of a String in PowerShell - Comparison With Substring to Check the Beginning of a String in PowerShell
- Conclusion
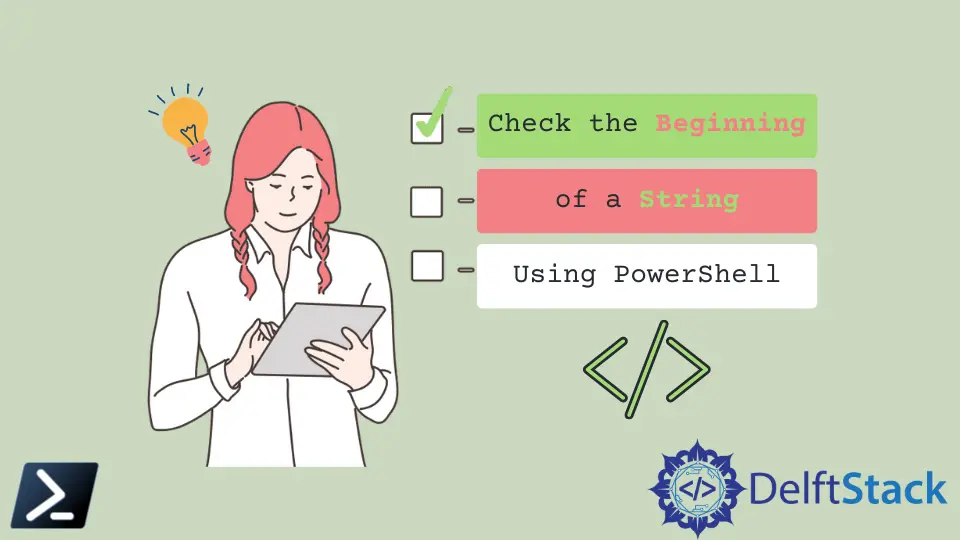
There may be instances where we encounter a use case that may require functionality to check if a string variable starts with a character or a string. Checking a string if it begins with a specific character or string is a common practice when making scripts and is also reasonably easy when written in Windows PowerShell.
This article will demonstrate how to check the beginning of a string variable using different methods in Windows PowerShell.
Using the -like
Logical Operator to Check the Beginning of a String in PowerShell
In PowerShell, the -Like
logical operator is a powerful tool for pattern matching. When utilized to check the beginning of a string, it allows for flexible comparisons based on wildcards.
By default, the -Like
operator ignores the case-sensitive statement. However, if we use logical operators, it must be partnered with an asterisk wildcard (*
).
Code:
$strVal = 'Hello World'
if ($strVal -like 'hello*') {
Write-Host "Your string starts with hello."
}
else {
Write-Host "Your string does not start with hello."
}
Output:
Your string starts with hello.
In the code, we initialize a string variable, $strVal = 'Hello World'
. We then use the -like
operator to check if the string ($strVal
) starts with the prefix 'hello'
.
The -like
operator allows for pattern matching using wildcards, and in this case, the asterisk (*
) represents zero or more characters. The conditional statement evaluates whether the string matches the pattern 'hello*'
.
In this case, the condition is true, and the output is Your string starts with hello
.
Using the -clike
Logical Operator to Check the Beginning of a String in PowerShell
The -clike
operator in PowerShell is used to perform a case-sensitive string comparison. It checks if a string matches a specified pattern, similar to the -like
operator, but it is case-sensitive.
This means that the -clike
operator will only return True
if the case of the characters in the string matches the case of the characters in the pattern exactly. We may use the -cLike
operator to perform a case-sensitive comparison.
Code:
$strVal = 'Hello World!'
if ($strVal -clike 'h*') {
Write-Host "Your string starts with lowercase h."
}
else {
Write-Host "Your string starts with uppercase H."
}
Output:
Your string starts with uppercase H.
In this code, we initialize a string variable, $strVal
, with the value 'Hello World!'
. We employ the -clike
operator, which is used for case-sensitive string comparison, to check if the string begins with the lowercase letter 'h'
.
The conditional statement evaluates whether the string matches the pattern 'h*'
in a case-sensitive manner. Since the condition is false, indicating that the string begins with an uppercase 'H'
, we output Your string starts with uppercase H
.
Remember, the -clike
operator is case-sensitive, so it will not match strings if the case of the characters does not match exactly. If we want to perform a case-insensitive comparison, we can use the -like
operator instead.
Using the StartsWith()
Function to Check the Beginning of a String in PowerShell
We can also use the .NET framework’s string extension function called StartsWith()
to check whether a string starts with a set of characters.
The StartsWith()
function in PowerShell is a method to verify if a string starts with a specified prefix. The StartsWith()
function is a built-in method for strings in PowerShell that returns a Boolean value indicating whether the given string starts with the specified substring.
Code:
$strVal = 'Hello World!'
if ($strVal.StartsWith('Hello')) {
Write-Host 'Your string starts with hello.'
}
else {
Write-Host 'Your string does not start with hello.'
}
Output:
Your string starts with hello.
In the above code, we check if the string variable $strVal
starts with 'Hello'
using the StartsWith()
method. Since the condition is true, we output Your string starts with hello
.
The StartsWith
function also accepts another argument that we can use to check for case-sensitive characters. This argument is CurrentCultureIgnoreCase
.
We’ll use the following method if we want to perform a case-sensitive comparison.
Code:
$strVal = 'Hello world'
if ($strVal.StartsWith('hello', 'CurrentCultureIgnoreCase')) {
Write-Host 'True'
}
else {
Write-Host 'False'
}
Output:
True
In this code, we check if the string variable $strVal
starts with 'hello'
in a case-insensitive manner using the StartsWith()
method with the 'CurrentCultureIgnoreCase'
parameter. Since the condition is true, we output True
.
Comparison With Substring to Check the Beginning of a String in PowerShell
In this approach, the Substring()
function is employed to compare a specified prefix with the initial portion of a given string. This comparison helps determine whether the string begins with the predefined sequence of characters.
In PowerShell, the Substring method allows extraction of a portion from a string based on the specified starting index and length. By using this method, we can acquire a substring from the original string and then compare it with a desired prefix to check if the string starts with that specific sequence.
Basic Syntax:
$substringToCompare = $strVal.Substring(0, $prefix.Length)
Parameters:
$strVal
- This is the variable holding the original string that we want to extract a substring from.0
- The0
is the starting index.$prefix.Length
- This is the length of the substring to be extracted. In our example, it’s the length of the prefix we want to check.$substringToCompare
- This is a variable that stores the result of the Substring operation. It will contain the substring extracted from the original string.
The syntax above essentially creates a substring from the original string, starting from the beginning, with a length equal to the length of the specified prefix.
Code:
$strVal = 'Hello World!'
$prefix = 'Hello'
if ($strVal.Substring(0, $prefix.Length) -eq $prefix) {
Write-Output "String starts with $prefix"
}
else {
Write-Output "String does not start with $prefix"
}
Output:
String starts with Hello
In this code, we have a string variable, $strVal = 'Hello World!'
and a prefix, $prefix = 'Hello'
. We use the Substring()
method to extract a portion of the original string starting from index 0
with a length identical to the length of the prefix.
The script then compares this extracted substring with the specified prefix using the -eq
operator. Since the condition is true, indicating that the string starts with 'Hello'
, we output String starts with Hello
.
Conclusion
In conclusion, this article has demonstrated various methods to check the beginning of a string variable in Windows PowerShell. We explored the use of the -like
logical operator for pattern matching, showcasing its flexibility with wildcards.
Additionally, we delved into the -clike
logical operator for case-sensitive comparisons. Furthermore, we covered the StartsWith()
function, utilizing both case-sensitive and case-insensitive checks to determine if the string starts with a specified prefix.
Finally, we examined the Substring()
function as an alternative approach, illustrating its application in comparing a substring with a predefined sequence and outputting results based on the evaluation. These methods offer versatility for different use cases, enhancing string comparison capabilities in PowerShell scripts.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell