How to Sort Multidimensional Array in PHP
- Use the Spaceship Operator to Sort the Multidimensional Array in PHP
-
Use the
usort()
Function With an Anonymous Comparision Function to Sort Multidimensional Array in PHP -
Use the
usort()
Function With a User-Defined Comparision Function to Sort Multidimensional Array in PHP
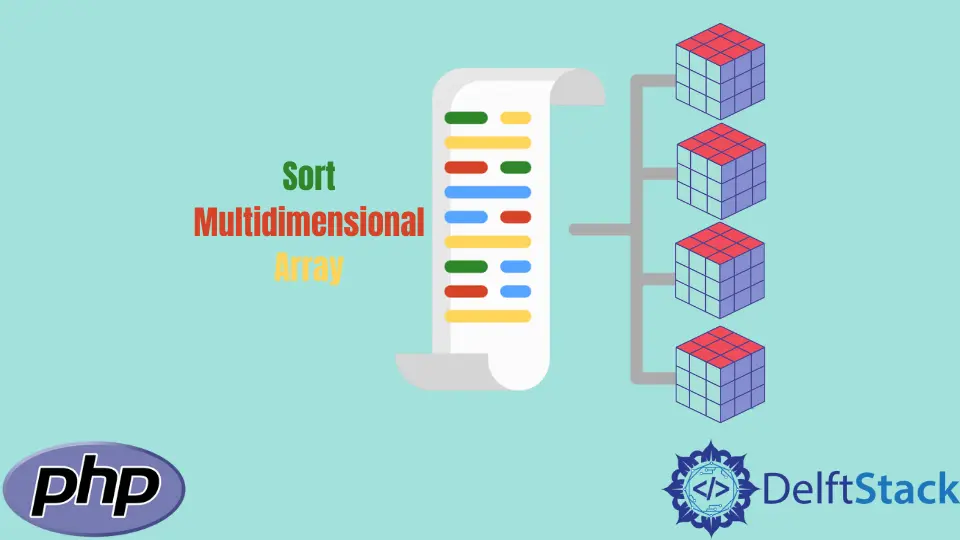
This article will introduce three methods to sort the multidimensional array by value in PHP.
Use the Spaceship Operator to Sort the Multidimensional Array in PHP
We can use the spaceship operator <=>
to sort the multidimensional array with values in PHP. We use the usort()
function along with the spaceship operator. The operator was introduced in PHP 7. It is used to compare the two values. It results in an integer value 0
if both the operands are equal, results in less than 0
if the left operand is less than the right operand, and results greater than 0
if the left operand is greater than the right operand. Likewise, the usort()
function sorts an array with values considering the user-defined comparison function. The syntax of the function is as below.
usort($array, $callback)
Here, $array
is the array that is to be sorted. The option $callback
is an anonymous callback function that compares the first and second arguments of the array.
For example, consider the following array to be sorted. We will sort the array with the value of the age
key.
$info = array(
array('name' => 'Jadon', 'age' => 21, 'country' => 'England'),
array('name' => 'Marcus', 'age' => 23, 'country' => 'England'),
array('name' => 'Anthony', 'age' => 24, 'country' => 'France'),
array('name' => 'Mason', 'age' => 19, 'country' => 'England'),
array('name' => 'Dan', 'age' => 23, 'country' => 'Wales'),
);
Inside the PHP opening tag, write the usort()
function with the $info
array and an anonymous function as the two arguments. The anonymous function has $x
and $y
as the parameters. Inside the body of the function, use the spaceship operator to compare the ages of two arguments as $x['age'] <=> $y['age']
and return the value. Next, close the PHP opening tag. Then, use the print_r()
PHP function to print the $info
array inside the HTML <pre>
tag.
Thus, the array is sorted according to the value age
as shown in the output section.
Example Code:
<?php
usort($info, function($x, $y) {
return $x['age'] <=> $y['age'];
});
?>
<pre><?php print_r($info);?> </pre>
Output:
Array
(
[0] => Array
(
[name] => Mason
[age] => 19
[country] => England
)
[1] => Array
(
[name] => Jadon
[age] => 21
[country] => England
)
[2] => Array
(
[name] => Marcus
[age] => 23
[country] => England
)
[3] => Array
(
[name] => Dan
[age] => 23
[country] => Wales
)
[4] => Array
(
[name] => Anthony
[age] => 24
[country] => France
)
)
Use the usort()
Function With an Anonymous Comparision Function to Sort Multidimensional Array in PHP
We can use the usort()
function used in the first method to sort the multidimensional array with values along with an anonymous callback function. In this method, we use the subtraction operator instead of the spaceship <=>
operator. We can subtract the values of the array according to which the array is sorted. Thus, we can sort the array. We will use the same array $info
to sort it by the value $age
. This method can be used before PHP 7 as the spaceship operator was only introduced in PHP 7.
For example, write the usort()
function with the parameters as above. In the body of the anonymous function, return the subtraction of the two arguments as $x['age'] - $y['age']
. Print the array with print_r()
as done above. We will receive the same output as in the first method.
Example Code:
<?php
usort($info, function($x, $y) {
return $x['age'] - $y['age'];
});
?>
<pre><?php print_r($info);?> </pre>
Use the usort()
Function With a User-Defined Comparision Function to Sort Multidimensional Array in PHP
This method is quite similar to the second method. The only difference is we will use a user-defined comparison function instead of the anonymous function. This method can be used before the PHP 5.3 version. It is because the concept of anonymous function was introduced in PHP 5.3.
For example, create an user-defined function sortByAge()
with $x
and $y
as the parameters. Inside the function body, written the subtraction of the ages as above. Outside the function write the usort()
function with the $info
array and sortByAge
as the arguments. Lastly, print the array.
The example below displays the same output as in the first method.
Example Code:
<?php
function sortByAge($x, $y) {
return $x['age'] - $y['age'];
}
usort($info, 'sortByAge');
?>
<pre><?php print_r($info);?> </pre>
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn