How to Reset Array in PHP
-
Use the
array_diff()
Function to Reset Array in PHP -
Use the
unset()
Function to Reset an Array in PHP
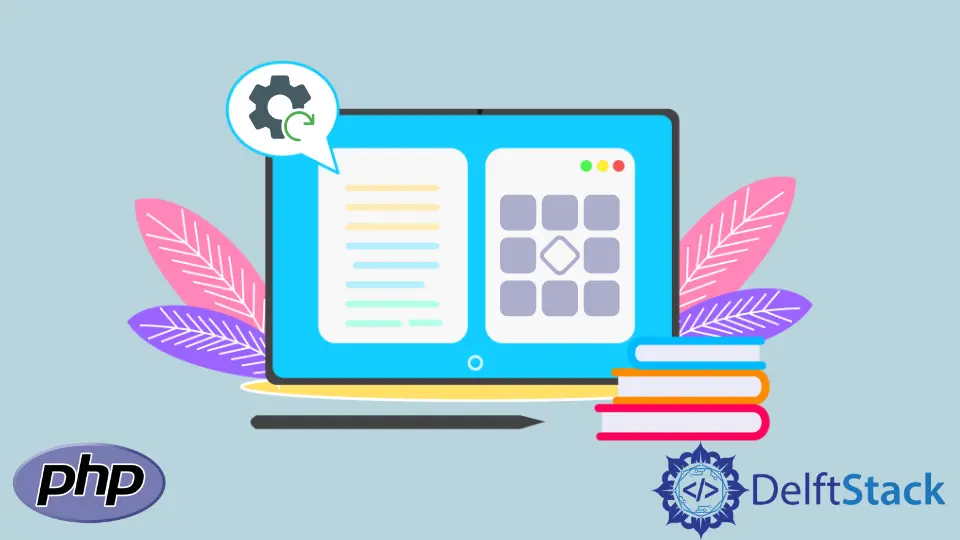
This article will introduce different methods to reset an array in PHP.
Use the array_diff()
Function to Reset Array in PHP
In PHP, we can use the array_diff()
function to reset an array. This function computes the difference of a given array with another array. The correct syntax to use this function is as follows.
array_diff($array, $Arr1, $Arr2, ...,$ArrN);
The function array_diff()
accepts N+1 parameters. The detail of its parameters is as follows.
Parameters | Description | |
---|---|---|
$array |
mandatory | It is the main array from which we want to remove a value. |
$Arr1 , $Arr2 , $ArrN |
mandatory | It is the array that we want to remove. The function will take its difference with the array and remove it from the array if it is present. |
The program that clears array values is as follows.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy");
echo("Array before deletion: \n");
var_dump($array);
$array = array_diff( $array, $array);
echo("Array after deletion: \n");
var_dump($array);
?>
Output:
Array before deletion:
array(6) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}
Array after deletion:
array(0) {
}
Use the unset()
Function to Reset an Array in PHP
We will use the unset()
function to clear array values. The unset()
functions resets a variable. The correct syntax to use this function is as follows.
unset($variable1, $variable2, ..., $variableN);
The built-in function unset()
has N parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
$variable1 , $variable2 , $variableN |
mandatory | It is the variable or data structure that we want to unset. At least one variable is mandatory. |
This function returns nothing.
The program below shows the way by which we can use the unset()
function to clear array values in PHP.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy");
echo("Array before deletion: \n");
var_dump($array);
unset($array);
echo("Array after deletion: \n");
var_dump($array);
?>
Output:
Array before deletion:
array(6) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}
Array after deletion:
NULL