How to Perform Array Delete by Value Not Key in PHP
-
Use the
array_search()
andunset()
Function to Perform Array Delete by Value Not Key in PHP -
Use the
array_diff()
Function to Perform Array Delete by Value Not Key in PHP
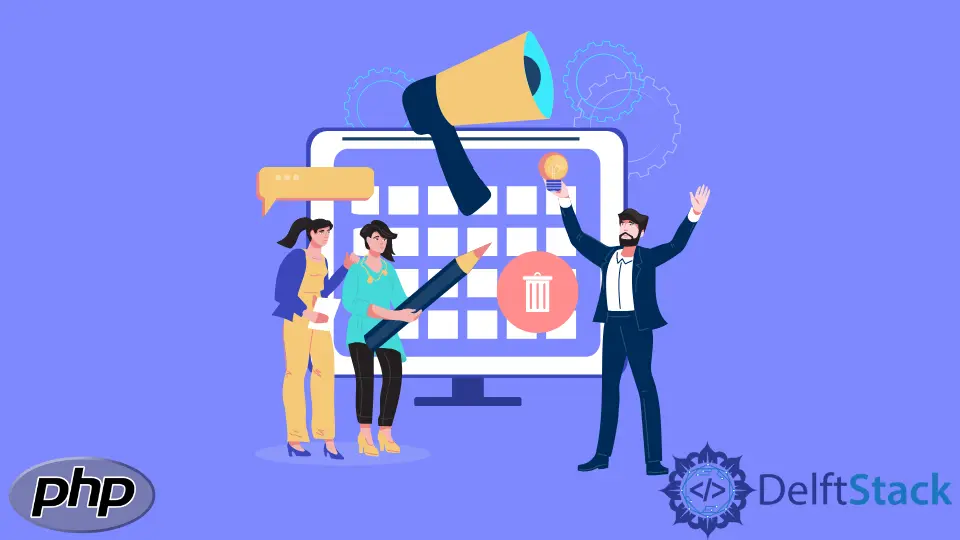
This article will introduce different methods to remove value from an array in PHP.
Use the array_search()
and unset()
Function to Perform Array Delete by Value Not Key in PHP
The main procedure to perform array delete by value, but not a key, is to find the value first. We could delete the value after it is found. We will find the value using array_search()
function and delete it using unset()
function. The unset()
functions resets a variable. The correct syntax to use these functions is as follows.
Syntax of array_search()
array_search($value, $array, $strict);
The built-in function array_search()
has three parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$value |
mandatory | It is the value we want to search in the array. |
$array |
mandatory | It is the array in which we will search the given value. |
$strict |
optional | If this parameter is set to True , then the function will also search the identical elements in the array. |
This function returns the key of the given value.
Syntax of unset()
unset($variable1, $variable2, ..., $variableN);
The built-in function unset()
has multiple parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$variable1 , $variable2 , $variableN |
mandatory | It is the variable or data structure that we want to unset. At least one variable is mandatory. |
This function returns nothing.
Example of Removing Values From an Array in PHP
The program below shows how we can use these functions to perform array delete by value, but not key, in PHP.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy");
echo("Array before deletion: \n");
var_dump($array);
$value = "Jasmine";
if (($key = array_search($value, $array)) !== false) {
unset($array[$key]);
}
echo("Array after deletion: \n");
var_dump($array);
?>
Output:
Array before deletion:
array(6) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}
Array after deletion:
array(5) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}
Use the array_diff()
Function to Perform Array Delete by Value Not Key in PHP
In PHP, we can also use the array_diff()
function to perform array delete by value not key. This function computes the difference of a given array with another array. The correct syntax to use this function is as follows.
Syntax
array_diff($array, $Arr1, $Arr2, ...,$ArrN);
The function array_diff()
accepts N+1 parameters. The detail of its parameters is as follows.
Parameters | Description | |
---|---|---|
$array |
mandatory | It is the main array from which we want to remove a value |
$Arr1 , $Arr2 , $ArrN |
mandatory | It is the array that we want to remove. The function will take its difference with the array and remove it from the array if it is present. |
The program that performs array delete by value, but not key, is as follows.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy");
echo("Array before deletion: \n");
var_dump($array);
$value = array("Jasmine");
$array = array_diff( $array, $value);
echo("Array after deletion: \n");
var_dump($array);
?>
Output:
Array before deletion:
array(6) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}
Array after deletion:
array(5) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}