How to Push Items to Associative Array in PHP
- What Is an Associative Array in PHP
-
Use the
array_push()
Method to Insert Items to an Associative Array in PHP -
Use the
array_merge()
Method to Insert Items to an Associative Array in PHP
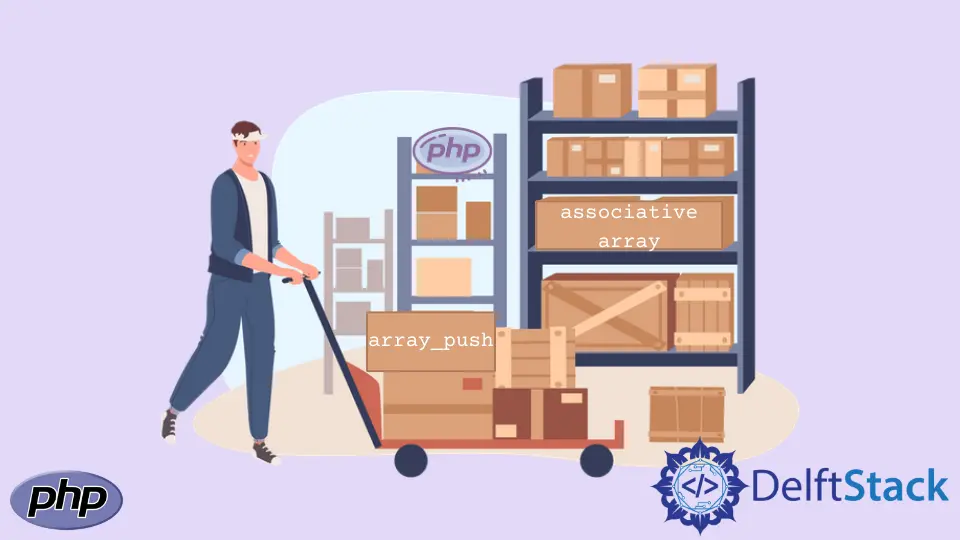
In this tutorial, we will see how to add items or elements into an associative array.
First, we will cover what and how to create an associative array. Then we will add elements into our associative array.
What Is an Associative Array in PHP
An associative array is an array with strings instead of indices. We store elements of an associative array using key values rather than linear indices.
Here is an example of an associative array and how we can use it.
<?php
// Create an array called age.
$age = array('Mike' => '24','Ann' => '19', 'Alice' => '32' );
echo "Mike is " . $age['Mike'] . ' years old.';
?>
Output:
Mike is 24 years old.
Use the array_push()
Method to Insert Items to an Associative Array in PHP
If we had an associative array shown below, how would we add new entries?
$color = array('a' => 'Red', 'b' => 'Blue' )
We will add two new colors to the above array in the example code below.
<?php
$color = array('a' => 'Red', 'b' => 'Blue' );
// Add Green and White to the array.
array_push($color, 'Green', 'White');
print_r($color);
?>
Output:
Array
(
[a] => Red
[b] => Blue
[0] => Green
[1] => White
)
Anytime you add an item to an array, it will assign numeric index keys.
Use the array_merge()
Method to Insert Items to an Associative Array in PHP
At some point, you will have an associative array like the one shown below.
$age = array("Mike" => "24","Ann" => "19", "Alice" => "19" )
How do we add an entry like John, aged 22?
The method array_push()
will not work in such a case. It would be best to use array_merge()
instead, as shown below.
<?php
$age = array("Mike" => "24","Ann" => "19", "Alice" => "19" );
$age1 = array("John" => "22");
//Merge the two arrays.
print_r(array_merge($age, $age1));
?>
Output:
Array
(
[Mike] => 24
[Ann] => 19
[Alice] => 19
[John] => 22
)
In the code above, we decided to add our new entry in the form of a new array. The function array_merge()
combines the two to form one array.
You can merge as many arrays as you want. If more elements share the same key, the last element will override the first one.
If you are confused, here is an example.
<?php
$color = array('a' => 'Red', 'b' => 'Blue' );
$color1 = array('b' => 'Neon', 'c' => 'Green');
print_r(array_merge($color,$color1));
?>
Output:
Array
(
[a] => Red
[b] => Neon
[c] => Green
)
As seen in the output, Blue
has been overwritten by Neon
.
As shown below, we use the array_merge_recursive()
to remedy this.
<?php
$color = array('a' => 'Red', 'b' => 'Blue' );
$color1 = array('b' => 'Neon', 'c' => 'Green');
print_r(array_merge_recursive($color,$color1));
?>
Output:
Array
(
[a] => Red
[b] => Array
(
[0] => Blue
[1] => Neon
)
[c] => Green
)
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn