How to Post Array From a Form in PHP
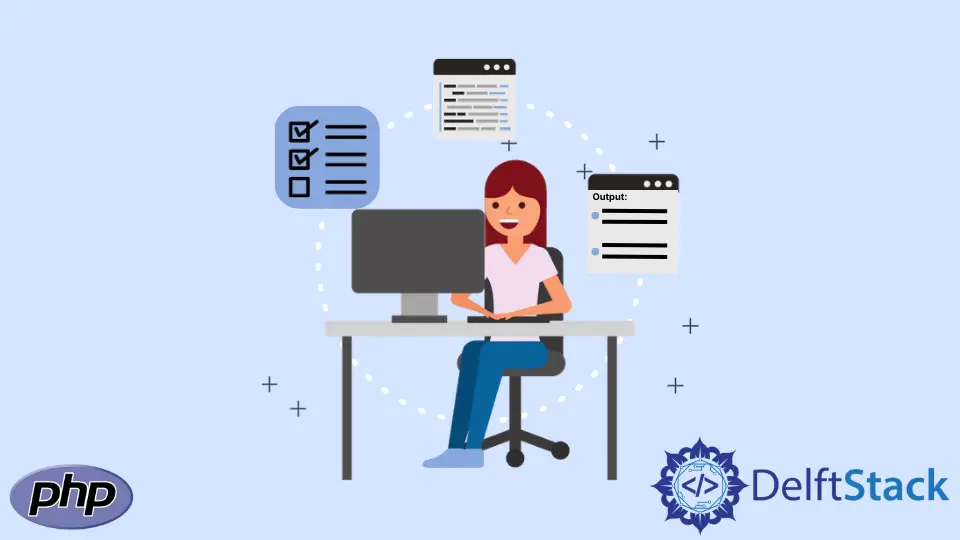
When working with forms in PHP, one common requirement is to send arrays from the client to the server. This can be particularly useful for scenarios like collecting multiple items, such as checkboxes or multiple fields for the same input type.
In this tutorial, we will explore how to effectively post an array from a form in PHP. You’ll learn the steps involved, including setting up your HTML form, retrieving the data in PHP, and processing it. By the end of this article, you’ll have a solid understanding of how to handle array data in PHP forms.
Setting Up Your HTML Form
To begin, we need to create a simple HTML form that allows users to input multiple values. For this example, let’s say we want to collect a list of favorite fruits. We will use checkboxes, which naturally allow for multiple selections.
<form action="process.php" method="post">
<label for="fruits">Choose your favorite fruits:</label><br>
<input type="checkbox" name="fruits[]" value="Apple"> Apple<br>
<input type="checkbox" name="fruits[]" value="Banana"> Banana<br>
<input type="checkbox" name="fruits[]" value="Cherry"> Cherry<br>
<input type="checkbox" name="fruits[]" value="Date"> Date<br>
<input type="submit" value="Submit">
</form>
In this form, we’ve defined checkboxes for different fruits. Notice the name
attribute for each checkbox is set to fruits[]
. The square brackets indicate that we are submitting an array. When the form is submitted, all selected values will be sent as an array to the specified action URL, which in this case is process.php
.
Processing the Array in PHP
Once the form is submitted, we need to handle the incoming data in our process.php
file. Here’s how we can retrieve and process the array of fruits.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
if (isset($_POST['fruits'])) {
$fruits = $_POST['fruits'];
foreach ($fruits as $fruit) {
echo $fruit . "<br>";
}
} else {
echo "No fruits selected.";
}
}
?>
In this PHP script, we first check if the request method is POST. This ensures that we are only processing data when the form is submitted. Next, we check if the fruits
array is set. If it is, we loop through each selected fruit and print it out. If no fruits were selected, we provide a message indicating that.
Output:
Apple
Banana
Cherry
This output displays each selected fruit on a new line. If no fruits were selected, the output would simply state “No fruits selected.” This simple script demonstrates how to handle an array from a form submission in PHP effectively.
Validating the Submitted Array
Validation is an essential aspect of handling form data. In our case, we want to ensure that the user has selected at least one fruit. Here’s how we can implement some basic validation in our process.php
file.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
if (isset($_POST['fruits']) && !empty($_POST['fruits'])) {
$fruits = $_POST['fruits'];
foreach ($fruits as $fruit) {
echo htmlspecialchars($fruit) . "<br>";
}
} else {
echo "Please select at least one fruit.";
}
}
?>
In this updated version, we added an additional check to ensure that the fruits
array is not only set but also not empty. If no fruits are selected, the user receives a prompt to select at least one fruit. Additionally, we are using htmlspecialchars()
to prevent XSS attacks by escaping special characters.
Output:
Banana
Cherry
This approach not only enhances security but also improves user experience by providing immediate feedback.
Conclusion
Posting an array from a form in PHP is a straightforward process that can significantly enhance your web applications. By using checkboxes or similar input types, you can easily collect multiple values and process them effectively. With the examples provided, you should now feel confident in creating forms that handle arrays and validating the submitted data. Remember, always ensure user inputs are sanitized to maintain security and integrity in your applications.
FAQ
-
How do I post an array using a different input type?
You can use input types like select with the multiple attribute or radio buttons, but remember that only one value will be sent for radio buttons unless you use checkboxes. -
Can I send arrays via GET instead of POST?
Yes, you can send arrays via the GET method by using the same naming convention (e.g.,name="fruits[]"
). However, keep in mind that GET requests have length limitations. -
What is the importance of using htmlspecialchars()?
Usinghtmlspecialchars()
helps prevent XSS attacks by converting special characters to HTML entities, ensuring that user input is safely displayed. -
Can I handle nested arrays in PHP forms?
Yes, you can handle nested arrays by using a naming convention likename="fruits[0][name]"
for your input fields. PHP will automatically convert this into a multi-dimensional array. -
What happens if no checkboxes are selected?
If no checkboxes are selected, thefruits
array will not be set, and you can handle this case by providing appropriate feedback to the user.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn