How to Loose and Strict Equality Checks in PHP
-
the Loose
Equality
Check in PHP -
the Loose
Not Equal
Check in PHP -
the Strict
Equality
Check in PHP -
the Strict
Not Equal
Check in PHP
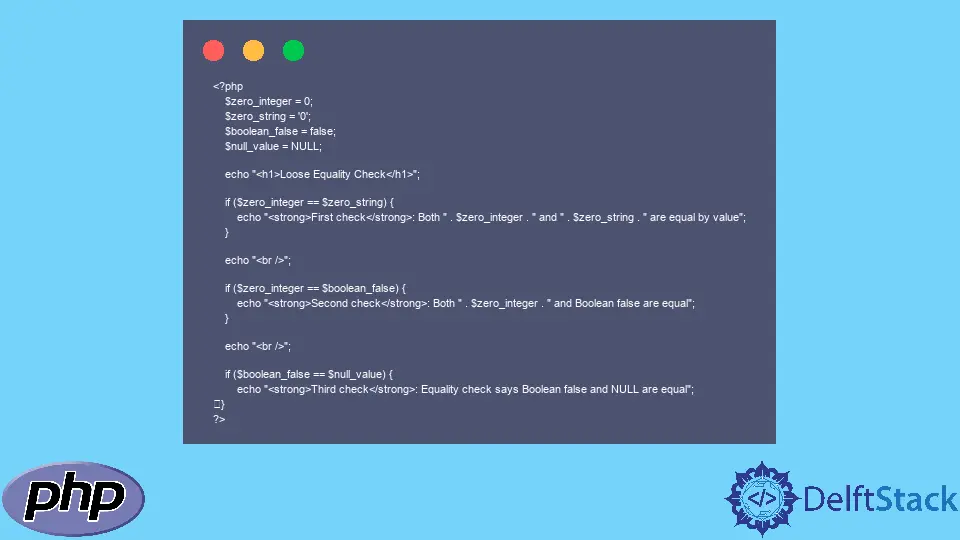
This article will explain how to perform loose and strict equality checks in PHP.
For the loose equality checks, you’ll use some built-in check operators like the equality operator ==
and the not equal operator !=
.
Also, for the strict equality checks, you’ll use the strict versions of these operators.
the Loose Equality
Check in PHP
The loose equality check in PHP uses the double equality sign ==
and not the equal sign !=
sign. Both operators are considered loose.
That is because they do not consider the data type of the compare variables. As a result, one in integer form will equal one in a string format.
We have three checks all will return true. The first check compares zero in string format and as a number. With the equality
operator, PHP will return true.
The equality
operator considers the values and not the data type. False and zero will return true for the second equality check because false means zero in computing terms.
But here, if you need to consider the data types of false and zero, an equality check will not suffice.
The final check between NULL and false should return false since NULL means an absence of value.
While false means the value is there, it’s empty or zero-value. But, the equality check returns true.
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
echo "<h1>Loose Equality Check</h1>";
if ($zero_integer == $zero_string) {
echo "<strong>First check</strong>: Both " . $zero_integer . " and " . $zero_string . " are equal by value";
}
echo "<br />";
if ($zero_integer == $boolean_false) {
echo "<strong>Second check</strong>: Both " . $zero_integer . " and Boolean false are equal";
}
echo "<br />";
if ($boolean_false == $null_value) {
echo "<strong>Third check</strong>: Equality check says Boolean false and NULL are equal";
}
?>
Output:
Loose Equality Check
First check: Both 0 and 0 are equal by value
Second check: Both 0 and Boolean false are equal
Third check: Equality check says Boolean false and NULL are equal
the Loose Not Equal
Check in PHP
Like loose equality check, loose not equal check does not consider the data type during comparison. As a result, you can get unexpected results.
Our next code example is based on the previous code example. But, here, we use the loose, not equal check to perform the comparison.
All checks will return false. This is because the loose, not equal
operator considers the value and not the data types of the variables.
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
echo "<h1>Loose Not Equal Check</h1>";
if ($zero_integer != $zero_string) {
echo "This statement will not print";
} else {
// This code will run
echo "Loose not equal thinks zero integer and zero string should be equal";
}
echo "<br />";
if ($zero_integer != $boolean_false) {
echo "This statement will not print";
} else {
echo "Loose not equal consider zero integer and Boolean false as equal";
}
echo "<br />";
if ($boolean_false != $null_value) {
echo "This statement will not print";
} else {
echo "Loose not equal thinks a null value and Boolean false are equal";
}
?>
The following outputs are from the else
block of each comparison:
Loose Not Equal Check
Loose not equal thinks zero integer and zero string should be equal
Loose not equal consider zero integer and Boolean false as equal
Loose not equal thinks a null value and Boolean false are equal
the Strict Equality
Check in PHP
As the name implies, the strict equality check is strict during comparisons. This means strict equality checks that the variables are equal in value and data types.
One string format will never equal one in number with a strict equality check. Also, Boolean false will not equal zero.
All checks will return the appropriate information about the variables during our next code block comparisons.
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
echo "<h1>Strict Equality Check</h1>";
if ($zero_integer === $zero_string) {
echo "This statement will not print";
} else {
echo "Zero integer and zero string are not equal.";
}
echo "<br />";
if ($zero_integer === $boolean_false) {
echo "This statement will not print";
} else {
echo "Zero integer and Boolean false are not the same.";
}
echo "<br />";
if ($boolean_false === $null_value) {
echo "This statement will not print";
} else {
echo "NULL and Boolean false are not the same.";
}
?
The following outputs are from the else
block of each comparison:
Strict Equality Check
Zero integer and zero string are not equal
Zero integer and Boolean false are not the same
NULL and Boolean false are not the same
the Strict Not Equal
Check in PHP
The strict, not equal
check will return the correct results when the comparison data and variables are not equal
.
When you run the next code block, you get the correct results about the comparisons.
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
// The space at the beginning of
// the string is not a mistake.
$comparison_end_quote = " do not belong to the same data type.";
echo "<h1>Strict Not Equal Check</h1>";
if ($zero_integer !== $zero_string) {
echo "True. Zero integer and zero String" . $comparison_end_quote;
}
echo "<br />";
if ($zero_integer !== $boolean_false) {
echo "True. Boolean false and zero Integer" . $comparison_end_quote;
}
echo "<br />";
if ($boolean_false !== $null_value) {
echo "True. Boolean is not NULL.";
}
?>
Output:
Strict Not Equal Check
True. Zero integer and zero String do not belong to the same data type.
True. Boolean false and zero Integer do not belong to the same data type.
True. Boolean is not NULL.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn