PHP 中的鬆散和嚴格相等檢查
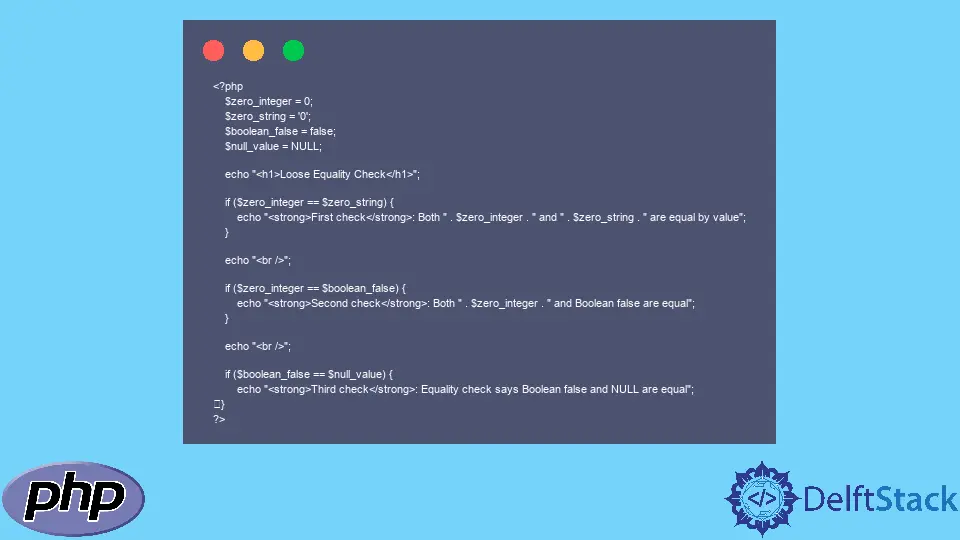
本文將解釋如何在 PHP 中執行鬆散和嚴格的相等檢查。
對於鬆散的相等檢查,你將使用一些內建的檢查運算子,例如相等運算子 ==
和不相等運算子!=
。
此外,對於嚴格的相等檢查,你將使用這些運算子的嚴格版本。
PHP 中的鬆散相等
檢查
PHP 中的鬆散相等檢查使用雙重等號 ==
,而不是等號!=
。兩個運算子都被認為是鬆散的。
那是因為他們沒有考慮比較變數的資料型別。因此,整數形式的一個將等於字串格式的一個。
我們有三個檢查都將返回 true。第一個檢查比較字串格式的零和一個數字。使用相等運算子,PHP 將返回 true。
equality
運算子考慮值而不是資料型別。False 和零將在第二次相等檢查中返回 true,因為 false 在計算方面意味著零。
但是在這裡,如果需要考慮 false 和 0 的資料型別,相等性檢查是不夠的。
NULL 和 false 之間的最終檢查應該返回 false,因為 NULL 意味著沒有值。
雖然 false 表示該值存在,但它是空值或零值。但是,相等性檢查返回 true。
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
echo "<h1>Loose Equality Check</h1>";
if ($zero_integer == $zero_string) {
echo "<strong>First check</strong>: Both " . $zero_integer . " and " . $zero_string . " are equal by value";
}
echo "<br />";
if ($zero_integer == $boolean_false) {
echo "<strong>Second check</strong>: Both " . $zero_integer . " and Boolean false are equal";
}
echo "<br />";
if ($boolean_false == $null_value) {
echo "<strong>Third check</strong>: Equality check says Boolean false and NULL are equal";
}
?>
輸出:
Loose Equality Check
First check: Both 0 and 0 are equal by value
Second check: Both 0 and Boolean false are equal
Third check: Equality check says Boolean false and NULL are equal
PHP 中的鬆散不等於
檢查
與鬆散相等檢查一樣,鬆散不相等檢查在比較時不考慮資料型別。因此,你可以獲得意想不到的結果。
我們的下一個程式碼示例基於前面的程式碼示例。但是,在這裡,我們使用鬆散的、不相等的檢查來執行比較。
所有檢查都將返回 false。這是因為鬆散的不等於
運算子考慮變數的值而不是資料型別。
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
echo "<h1>Loose Not Equal Check</h1>";
if ($zero_integer != $zero_string) {
echo "This statement will not print";
} else {
// This code will run
echo "Loose not equal thinks zero integer and zero string should be equal";
}
echo "<br />";
if ($zero_integer != $boolean_false) {
echo "This statement will not print";
} else {
echo "Loose not equal consider zero integer and Boolean false as equal";
}
echo "<br />";
if ($boolean_false != $null_value) {
echo "This statement will not print";
} else {
echo "Loose not equal thinks a null value and Boolean false are equal";
}
?>
以下輸出來自每個比較的 else
塊:
Loose Not Equal Check
Loose not equal thinks zero integer and zero string should be equal
Loose not equal consider zero integer and Boolean false as equal
Loose not equal thinks a null value and Boolean false are equal
PHP 中嚴格的相等
檢查
顧名思義,嚴格相等檢查在比較期間是嚴格的。這意味著嚴格相等檢查變數的值和資料型別是否相等。
通過嚴格的相等性檢查,一種字串格式在數量上永遠不會等於一。此外,布林 false 將不等於零。
在我們的下一個程式碼塊比較期間,所有檢查都將返回有關變數的適當資訊。
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
echo "<h1>Strict Equality Check</h1>";
if ($zero_integer === $zero_string) {
echo "This statement will not print";
} else {
echo "Zero integer and zero string are not equal.";
}
echo "<br />";
if ($zero_integer === $boolean_false) {
echo "This statement will not print";
} else {
echo "Zero integer and Boolean false are not the same.";
}
echo "<br />";
if ($boolean_false === $null_value) {
echo "This statement will not print";
} else {
echo "NULL and Boolean false are not the same.";
}
?
以下輸出來自每個比較的 else
塊:
Strict Equality Check
Zero integer and zero string are not equal
Zero integer and Boolean false are not the same
NULL and Boolean false are not the same
PHP 中的嚴格不等於
檢查
當比較資料和變數不等於
時,嚴格的不等於
檢查將返回正確的結果。
當你執行下一個程式碼塊時,你將獲得有關比較的正確結果。
<?php
$zero_integer = 0;
$zero_string = '0';
$boolean_false = false;
$null_value = NULL;
// The space at the beginning of
// the string is not a mistake.
$comparison_end_quote = " do not belong to the same data type.";
echo "<h1>Strict Not Equal Check</h1>";
if ($zero_integer !== $zero_string) {
echo "True. Zero integer and zero String" . $comparison_end_quote;
}
echo "<br />";
if ($zero_integer !== $boolean_false) {
echo "True. Boolean false and zero Integer" . $comparison_end_quote;
}
echo "<br />";
if ($boolean_false !== $null_value) {
echo "True. Boolean is not NULL.";
}
?>
輸出:
Strict Not Equal Check
True. Zero integer and zero String do not belong to the same data type.
True. Boolean false and zero Integer do not belong to the same data type.
True. Boolean is not NULL.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn