How to Initialize Empty Array in PHP
-
Use the Square Brackets
[]
to Initialize an Empty Array in PHP -
Use the
array()
Function to Initialize an Empty Array in PHP
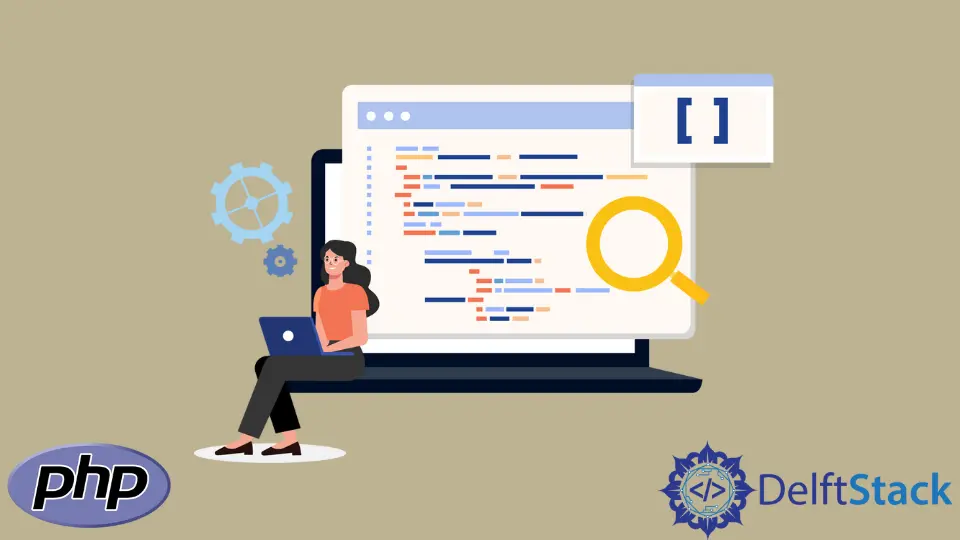
This article will introduce different methods to initialize an empty array in PHP.
Use the Square Brackets []
to Initialize an Empty Array in PHP
In PHP, we have multiple methods and functions for initializing an empty array. We can use square brackets []
to initialize the array. The correct syntax to use square brackets is as follows.
$arrayName = [];
The above syntax will create an empty array. We can also add elements to this array using square brackets. The program below shows the way by which we can use the square brackets []
to initialize an empty array in PHP.
<?php
$myarray = [];
echo("This is an empty array.\n");
var_dump($myarray);
?>
Output:
This is an empty array.
array(0) {
}
We can also use []
to add elements to the array.
<?php
$myarray = [];
echo("This is an empty array.\n");
var_dump($myarray);
$myarray = [
0=> "Sara",
1=> "John",
2=> "Melissa",
3=> "Tom",
];
echo("Now the array has four elements.\n");
var_dump($myarray);
?>
Output:
This is an empty array.
array(0) {
}
Now the array has four elements.
array(4) {
[0]=>
string(4) "Sara"
[1]=>
string(4) "John"
[2]=>
string(7) "Melissa"
[3]=>
string(3) "Tom"
}
Use the array()
Function to Initialize an Empty Array in PHP
We can also use the array()
function to initialize an empty array in PHP. This function is a specialized function for creating an array. The correct syntax to use this function is as follows.
array($index1=>$value1, $index2=>$value2, ...,$indexN=>$valueN);
The array function has N parameters. N is the number of elements the array will have. The details of its parameters are as follows.
Variables | Description |
---|---|
$index1 , $index2 , …, $indexN |
It is the index of the array elements. It can be an integer or a string. |
$value1 , $value2 , …, $valueN |
It is the value of the array elements. |
The program below shows the way by which we can use the array()
function to initialize an empty array in PHP.
<?php
$myarray = array();
echo("This is an empty array.\n");
var_dump($myarray);
?>
Output:
This is an empty array.
array(0) {
}
Likewise, we can use this operator to write N multi-line strings.
<?php
$myarray = array();
echo("This is an empty array.\n");
var_dump($myarray);
$myarray = array(
0=> "Sara",
1=> "John",
2=> "Melissa",
3=> "Tom",
);
echo("Now the array has four elements.\n");
var_dump($myarray);
?>
Output:
This is an empty array.
array(0) {
}
Now the array has four elements.
array(4) {
[0]=>
string(4) "Sara"
[1]=>
string(4) "John"
[2]=>
string(7) "Melissa"
[3]=>
string(3) "Tom"
}