How to Flatten Array in PHP
-
Use the
Recursiveiteratoriterator
andRecursivearrayiterator
to Operate Flatenning the Array in PHP -
Use the
array_walk_recursive
to Flatten a Multidimensional Array in PHP -
Use the
for
Loop to Flatten a Multidimensional Array in PHP -
Use the
while
Loop to Flatten a Multidimensional Array in PHP -
Use the
foreach
Loop to Flatten a Multidimensional Array in PHP
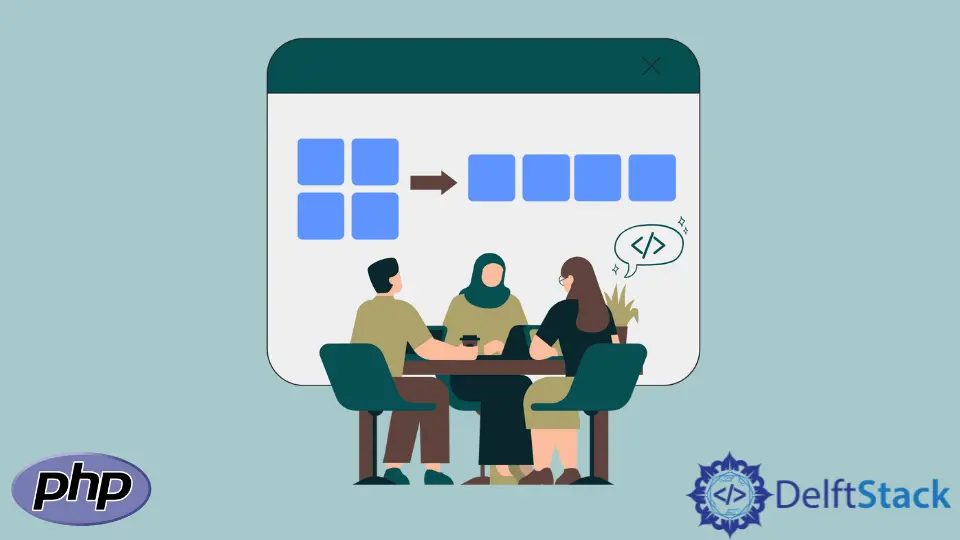
Flatten array means converting a multidimensional array into a one-dimensional array. PHP has different ways to flatten an array, and we will discuss them here.
Use the Recursiveiteratoriterator
and Recursivearrayiterator
to Operate Flatenning the Array in PHP
The SPL library has iterators that are used to flatten the multidimensional arrays. We used two iterators, RecursiveIteratorIterator
and RecursiveArrayIterator
, to operate flattening the array.
<?php
$demo_array = array('a', 'b', 'c', 'd', array('e', 'f', 'g', 'h', array('i', 'j', 'k'), 'l', 'm', 'n', 'o'), 'p', 'q', 'r', 's');
$iter_object = new RecursiveIteratorIterator(new RecursiveArrayIterator($demo_array));
$flatten_array= array();
foreach($iter_object as $value) {
array_push($flatten_array,$value);
}
print_r($flatten_array);
?>
Output:
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h [8] => i [9] => j [10] => k [11] => l [12] => m [13] => n [14] => o [15] => p [16] => q [17] => r [18] => s )
The code contains a three-layer array that will be flattened in a one-dimensional array.
Use the array_walk_recursive
to Flatten a Multidimensional Array in PHP
The built-in function array_walk_recursive
can be used with a closure function to flatten a multidimensional array in PHP.
<?php
function flatten_array(array $demo_array) {
$new_array = array();
array_walk_recursive($demo_array, function($array) use (&$new_array) { $new_array[] = $array; });
return $new_array;
}
$demo_array = array('a', 'b', 'c', 'd', array('e', 'f', 'g', 'h', array('i', 'j', 'k'), 'l', 'm', 'n', 'o'), 'p', 'q', 'r', 's');
$new_array = flatten_array($demo_array);
print_r($new_array);
?>
Output:
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h [8] => i [9] => j [10] => k [11] => l [12] => m [13] => n [14] => o [15] => p [16] => q [17] => r [18] => s )
The array_walk_recursive
with a closure function flattens the given multidimensional array.
Use the for
Loop to Flatten a Multidimensional Array in PHP
We can use for
loops to flatten multidimensional arrays; additional built-in functions may be required.
<?php
function flatten_array($demo_array,$new_array) {
for($i = 0; $i < count($demo_array); $i++) {
if(is_array($demo_array[$i])) {
$new_array = flatten_array($demo_array[$i], $new_array);
}
else {
if(isset($demo_array[$i])) {
$new_array[] = $demo_array[$i];
}
}
}
return $new_array;
}
$demo_array = array('a', 'b', 'c', 'd', array('e', 'f', 'g', 'h', array('i', 'j', 'k'), 'l', 'm', 'n', 'o'), 'p', 'q', 'r', 's');
print_r(flatten_array($demo_array, array()));
?>
Output:
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h [8] => i [9] => j [10] => k [11] => l [12] => m [13] => n [14] => o [15] => p [16] => q [17] => r [18] => s )
The above code will generate a flattened array by iterating the given array with the help of the for
loop.
Use the while
Loop to Flatten a Multidimensional Array in PHP
<?php
function flatten_array ($demo_array){
$x = 0;
while ($x < count ($demo_array)){
while (is_array ($demo_array[$x])){
if (!$demo_array[$x]){
array_splice ($demo_array, $x, 1);
--$x;
break;
}
else{
array_splice ($demo_array, $x, 1, $demo_array[$x]);
}
}
++$x;
}
return $demo_array;
}
$demo_array = array('a', 'b', 'c', 'd', array('e', 'f', 'g', 'h', array('i', 'j', 'k'), 'l', 'm', 'n', 'o'), 'p', 'q', 'r', 's');
print_r(flatten_array($demo_array));
?>
Output:
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h [8] => i [9] => j [10] => k [11] => l [12] => m [13] => n [14] => o [15] => p [16] => q [17] => r [18] => s )
The given array flattens with the help of the while
loop and array_splice
function, which is used to remove a part of an array and replace it with something else.
Use the foreach
Loop to Flatten a Multidimensional Array in PHP
<?php
function flatten_array($demo_array) {
if (!is_array($demo_array)) {
// make sure the input is an array
return array($demo_array);
}
$new_array = array();
foreach ($demo_array as $value) {
$new_array = array_merge($new_array, flatten_array($value));
}
return $new_array;
}
$demo_array = array('a', 'b', 'c', 'd', array('e', 'f', 'g', 'h', array('i', 'j', 'k'), 'l', 'm', 'n', 'o'), 'p', 'q', 'r', 's');
print_r(flatten_array($demo_array));
?>
Output:
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h [8] => i [9] => j [10] => k [11] => l [12] => m [13] => n [14] => o [15] => p [16] => q [17] => r [18] => s )
The given array flattens using the foreach
loop and array_merge()
function, which combines two arrays into one.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook