How to Display Array Values in PHP
-
Use the
foreach
Loop to Display Array Values in PHP -
Use the
print_r()
andvar_dump()
to Display the Information of an Array in PHP -
Use the
implode()
orjson_encode()
to Convert Array Into String in PHP -
Use the
foreach
Loop andarray_map()
Function to Display Values of a Multidimensional Array in PHP
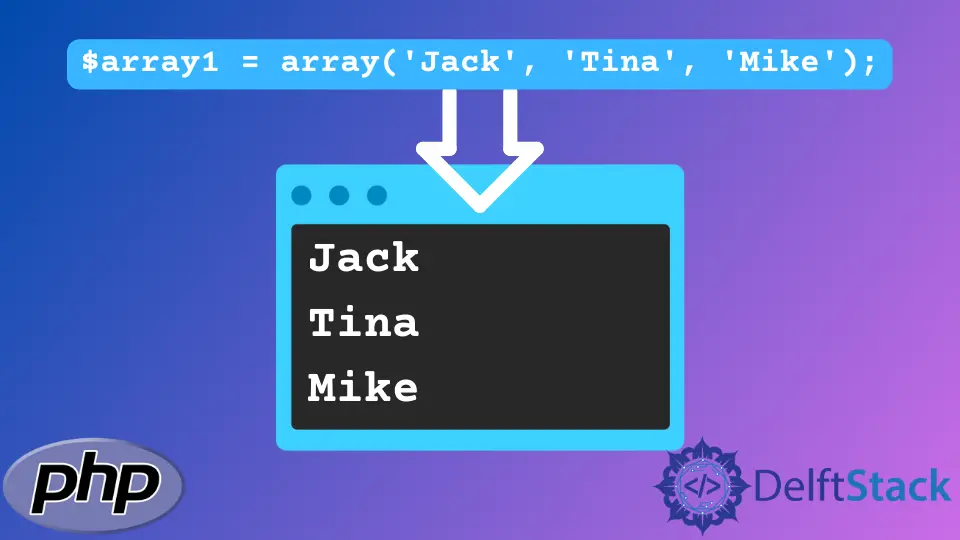
This article will introduce how to display array values in PHP.
The foreach
loop can be used to display each value. The built-in functions like print_r()
or var_dump
can dump all the information of an array.
We can also convert arrays to strings to display the values.
Use the foreach
Loop to Display Array Values in PHP
The foreach
loop can echo each value of an array. As the associative arrays have both keys and values, we display both.
<?php
//Simple one dimensional array
$demo_array1 = array('Jack', 'Shawn', 'Michelle', 'Maria');
//associative array
$demo_array2 = array(1 => 'Jack', 2 => 'Shawn', 3 => 'Michelle', 4 => 'Maria');
echo "The values for first array are: <br>";
foreach($demo_array1 as $value){
echo $value."<br>";
}
echo "The values for associative array: <br>";
foreach($demo_array2 as $key => $value){
echo "The Key <b>". $key."</b> has the value <b>".$value."</b><br>" ;
}
?>
Output:
The values for first array are:
Jack
Shawn
Michelle
Maria
The values for associative array:
The Key **1** has the value **Jack**
The Key **2** has the value **Shawn**
The Key **3** has the value **Michelle**
The Key **4** has the value **Maria**
Use the print_r()
and var_dump()
to Display the Information of an Array in PHP
The built-in functions print_r()
and var_dump()
are used to dump the information of arrays in PHP.
<?php
//Simple one dimensional array
$demo_array1 = array('Jack', 'Shawn', 'Michelle', 'Maria');
//associative array
$demo_array2 = array('Name1' => 'Jack', 'Name2' => 'Shawn', 'Name3' => 'Michelle', 'Name4' => 'Maria');
echo "The values for first array using print_r: <br>";
print_r($demo_array1);
echo "The values for first array using var_dump: <br>";
var_dump($demo_array1);
echo "The values for associative array using print_r: <br>";
print_r($demo_array2);
echo "The values for associative array using var_dump: <br>";
var_dump($demo_array2);
?>
Output:
The values for first array using print_r:
Array ( [0] => Jack [1] => Shawn [2] => Michelle [3] => Maria )
The values for first array using var_dump:
array(4) { [0]=> string(4) "Jack" [1]=> string(5) "Shawn" [2]=> string(8) "Michelle" [3]=> string(5) "Maria" }
The values for associative array using print_r:
Array ( [Name1] => Jack [Name2] => Shawn [Name3] => Michelle [Name4] => Maria )
The values for associative array using var_dump:
array(4) { ["Name1"]=> string(4) "Jack" ["Name2"]=> string(5) "Shawn" ["Name3"]=> string(8) "Michelle" ["Name4"]=> string(5) "Maria" }
The print_r
will only display key values of the array. However, var_dump
will display array length, value length, keys, and values.
Use the implode()
or json_encode()
to Convert Array Into String in PHP
The built-in function implode()
or json_encode()
can be used to convert arrays to strings to display values.
<?php
//Simple one dimensional array
$demo_array1 = array('Jack', 'Shawn', 'Michelle', 'Maria');
//associative array
$demo_array2 = array('Name1' => 'Jack', 'Name2' => 'Shawn', 'Name3' => 'Michelle', 'Name4' => 'Maria');
echo "The values for first array using implode: <br>";
$implode_string=implode(",",$demo_array1);
echo $implode_string."<br>";
$implode_string1=implode(",",$demo_array2);
echo $implode_string1."<br>";
echo "The values for associative array using json_encode: <br>";
$json_string=json_encode($demo_array1);
echo $json_string."<br>";
$json_string1=json_encode($demo_array2);
echo $json_string1."<br>";
?>
Output:
The values for the first array using implode:
Jack,Shawn,Michelle,Maria
Jack,Shawn,Michelle,Maria
The values for associative array using json_encode:
["Jack","Shawn","Michelle","Maria"]
{"Name1":"Jack","Name2":"Shawn","Name3":"Michelle","Name4":"Maria"}
As we can see, implode()
does not show keys for the associative array, but json_encode()
also shows the keys for an associative array.
Use the foreach
Loop and array_map()
Function to Display Values of a Multidimensional Array in PHP
We will display the values as a list from a 2D array using foreach
loops or array_map
built-in function.
<?php
$demo_array = Array (
0 => Array ( "Name" => "Jack" , "Salary" => 4000 ) ,
1 => Array ( "Name" => "Mike" , "Salary" => 3500 ) ,
2 => Array ( "Name" => "Tina" , "Salary" => 3000 ) );
//Using foreach loop
echo "<pre>";
echo "Name\tSalary";
foreach ( $demo_array as $value ) {
echo "\n", $value['Name'], "\t", $value['Salary'];
}
//Using array_map() function
echo "<pre>" ;
echo "Name\tSalary";
array_map(function ($value) {
echo "\n", $value['Name'], "\t", $value['Salary'];
}, $demo_array);
?>
The code above will display a list of name names with salaries.
Output:
Name Salary
Jack 4000
Mike 3500
Tina 3000
Name Salary
Jack 4000
Mike 3500
Tina 3000
Similarly, we can set multiple foreach
loops for multidimensional arrays with more layers or set a function in the array_map()
parameter.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook