Constant Arrays in PHP
- What are Constant Arrays in PHP?
- Defining Constant Arrays in PHP
- Benefits of Using Constant Arrays
- Conclusion
- FAQ
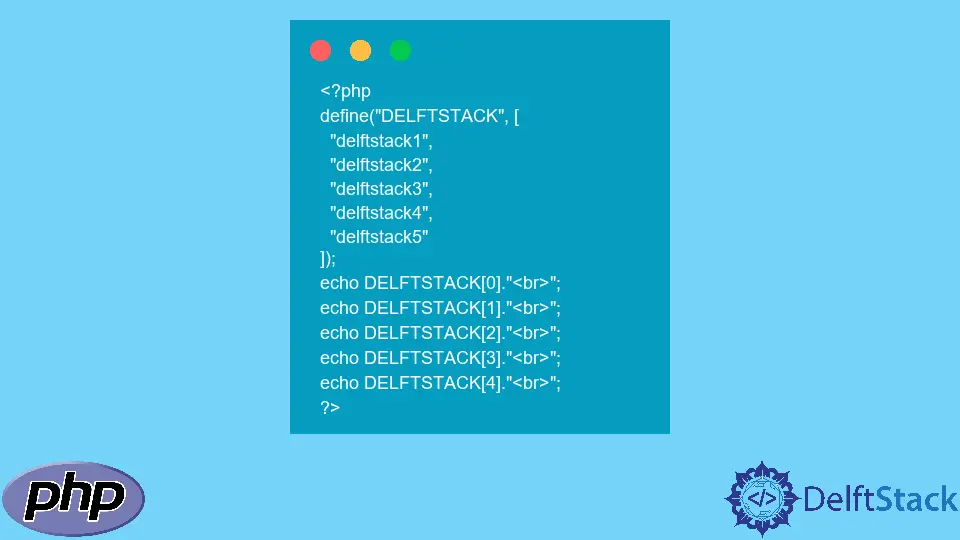
When it comes to programming in PHP, understanding how to work with constant arrays can significantly enhance your coding practices. Constant arrays allow developers to define arrays that cannot be modified after their initial declaration. This feature can be particularly useful when you want to ensure that certain values remain unchanged throughout the execution of your script.
In this tutorial, we will explore the concept of constant arrays in PHP, how to define them, and why they are beneficial in various programming scenarios. By the end of this article, you will have a solid grasp of constant arrays and how to implement them effectively in your PHP projects.
What are Constant Arrays in PHP?
In PHP, a constant array is an array that is defined using the define()
function or the const
keyword. Once set, the values in a constant array cannot be altered, thus providing a layer of data integrity. This is particularly useful in applications where certain configurations or settings should remain static, ensuring that your code behaves predictably.
For instance, if you have a set of configuration values that your application relies on, defining them as constant arrays can prevent accidental changes that could lead to bugs or unexpected behavior. Here’s how you can define a constant array in PHP:
define('CONFIG', [
'db_host' => 'localhost',
'db_user' => 'root',
'db_pass' => 'password',
]);
// Attempting to modify the constant array will result in an error
// CONFIG['db_user'] = 'admin'; // This will cause an error
Output:
Error: Cannot modify constant array
In the example above, we define a constant array called CONFIG
that holds database connection parameters. If you try to change any of these values later in your code, PHP will throw an error, ensuring that the integrity of your configuration remains intact.
Defining Constant Arrays in PHP
To define a constant array in PHP, you can use either the define()
function or the const
keyword. Both methods achieve the same goal, but they have different syntax and usage contexts. Let’s take a closer look at each method.
Using the define()
function
The define()
function allows you to create a constant that can hold an array. This is particularly useful for global constants that you want to access from anywhere in your application. Here’s how to do it:
define('FRUITS', ['apple', 'banana', 'cherry']);
// Accessing the constant array
echo FRUITS[0]; // Outputs: apple
Output:
apple
In this example, we define a constant array called FRUITS
containing three fruit names. You can access the elements of the array just like any other array in PHP. However, remember that you cannot change the contents of FRUITS
after its declaration.
Using the const keyword
Alternatively, you can use the const
keyword to define a constant array, but this method can only be used in a class context. Here’s an example:
class FruitBasket {
const FRUITS = ['apple', 'banana', 'cherry'];
}
// Accessing the constant array
echo FruitBasket::FRUITS[1]; // Outputs: banana
Output:
banana
In this scenario, we create a class called FruitBasket
and define a constant array called FRUITS
within it. To access the array, you must reference it through the class name. This method is particularly useful when you want to encapsulate constants within a class structure.
Benefits of Using Constant Arrays
Using constant arrays in your PHP applications comes with several advantages. Here are a few key benefits that highlight their importance:
-
Data Integrity: By defining an array as constant, you ensure that its values remain unchanged throughout the script. This is crucial for configurations, settings, or any data that should not be altered once set.
-
Improved Readability: Constant arrays can make your code more readable. When you see a constant name, you immediately understand that its value is fixed, which can help in understanding the flow of the application.
-
Error Prevention: By preventing modifications to constant arrays, you reduce the likelihood of introducing bugs into your code. This is particularly important in larger applications where tracking changes can become cumbersome.
-
Global Access: Using the
define()
function allows you to create constants that can be accessed globally throughout your application. This can simplify your code and make it easier to manage configurations.
In summary, constant arrays are a powerful feature in PHP that can enhance your coding practices by promoting data integrity, improving readability, and preventing errors. Whether you choose to use the define()
function or the const
keyword, leveraging constant arrays can lead to cleaner and more maintainable code.
Conclusion
In this tutorial, we explored the concept of constant arrays in PHP. We learned how to define them using both the define()
function and the const
keyword, and we discussed the benefits of using constant arrays in your applications. By implementing constant arrays, you can ensure data integrity, improve code readability, and prevent errors, making your PHP projects more robust and maintainable. As you continue your journey in PHP programming, consider incorporating constant arrays into your coding practices for a more structured and reliable approach to managing data.
FAQ
-
What is a constant array in PHP?
A constant array in PHP is an array defined using thedefine()
function or theconst
keyword, where its values cannot be modified after declaration. -
How do I define a constant array using the define() function?
You can define a constant array using thedefine()
function by passing the constant name and an array as arguments. -
Can I modify a constant array after it is defined?
No, once a constant array is defined, its values cannot be modified, and attempting to do so will result in an error. -
What are the advantages of using constant arrays in PHP?
The advantages include data integrity, improved readability, error prevention, and global access to the defined constants. -
Can I use constant arrays in classes?
Yes, you can define constant arrays in classes using theconst
keyword, which allows you to encapsulate constants within a class structure.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook