How to Compare Two Arrays in PHP
-
Use the
==
and!=
Operators to Compare Two Arrays in PHP -
Use the
===
and!==
Operators to Compare Two Arrays in PHP -
Use the
sort()
Function to Compare Two Arrays When Order Is Not Important in PHP -
Use the
array_diff()
Function to Compare Two Arrays and Return the Difference in PHP -
Use the
array_intersect()
Function to Compare Two Arrays and Return the Matches in PHP
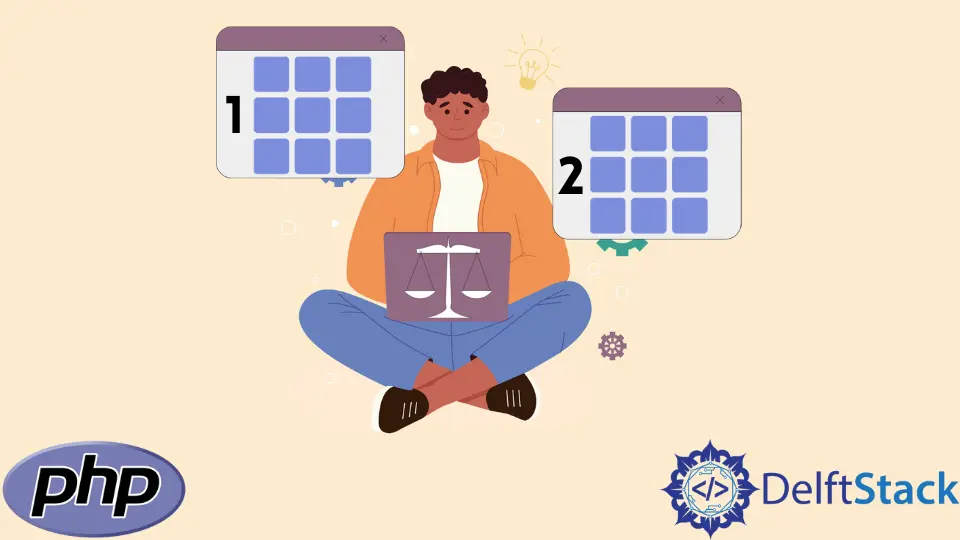
All sorts of operations are done with arrays with different complexities, and comparison operations are no different. When we compare two values, we intend to understand how similar or dissimilar they are.
With numbers (integers and float), characters, and strings, it can be straightforward. However, with arrays, it can become a little bit complicated.
Don’t fret because it is easy, and there are different operators that we can use. Whether the comparison is strict or not, there is an operator.
This article will consider four operators and three functions that make comparing two arrays easy and how to make it happen with excellent examples.
Use the ==
and !=
Operators to Compare Two Arrays in PHP
The first operator is the ==
operator. It is the equality operator, and when we compare two arrays with this operator, we intend to know whether the first array is equal to the second array.
Comparison operations focus on finding the similarities or dissimilarities between two values. So, with the ==
operator, you obtain a Boolean response, where TRUE represents similarity and FALSE represents dissimilarity.
Here, the code uses the ==
operator to compare two arrays.
<?php
$arr1 = array(4, 5, 'hello', 2.45, 3.56);
$arr2 = array(5, 2.45, 'hello', 3.56, 4);
// Check for equality
if ($arr1 == $arr2)
echo "Similar Arrays";
else
echo "Not Similar Arrays";
?>
Output:
Not Similar Arrays
You can use the !=
operator, an inequality operator. When we compare two arrays with this operator, we intend to know whether the first array is not equal to the second array.
It releases a Boolean response, where TRUE represents dissimilarity and FALSE represents similarity.
<?php
$arr1 = array(4, 5, 'hello', 2.45, 3.56);
$arr2 = array(5, 2.45, 'hello', 3.56, 4);
// Check for inequality
if ($arr1 != $arr2)
echo "Not Similar Arrays";
else
echo "Similar Arrays";
?>
Output:
Not Similar Arrays
These two operators work with associative and multi-dimensional arrays and will only compare the values, not the keys.
Use the ===
and !==
Operators to Compare Two Arrays in PHP
Similar to the ==
operators, the ===
operator allows for comparing two values for similarity. However, the ==
operator will return TRUE if the first array and second array, in the context of associative arrays, have the same key/value pairs.
However, ===
will return TRUE if the first array and second array have the same key/value pairs in the same order and of the same types.
Using the same codes in the previous section, we will make some type changes and use both the ==
and ===
operators.
<?php
$arr1 = array(4, 5, 'hello', 2.45, 3.56);
$arr2 = array('4', '5', 'hello', '2.45', '3.56');
// Check for equality
if ($arr1 == $arr2)
echo "Similar Arrays";
else
echo "Not Similar Arrays";
?>
Output:
Similar Arrays
When we use the ===
operator
<?php
$arr1 = array(4, 5, 'hello', 2.45, 3.56);
$arr2 = array('4', '5', 'hello', '2.45', '3.56');
// Check for equality
if ($arr1 === $arr2)
echo "Similar Arrays";
else
echo "Not Similar Arrays";
?>
When we use it with associative arrays, we see the same behavior. In the code below, we will compare the two arrays in terms of their value and not keys.
<?php
$arr1 = array("first" => 4, "second" => 5, "type" => 'hello');
$arr2 = array("one" => '4', "two" => '5', "third" => 'hello', );
// Check for equality
if ($arr1 === $arr2)
echo "Similar Arrays";
else
echo "Not Similar Arrays";
?>
Output:
Not Similar Arrays
Use the sort()
Function to Compare Two Arrays When Order Is Not Important in PHP
For cases where the order of the element isn’t important, you can use the sort()
function and compare the two arrays. Therefore, you apply the sort()
function to both arrays and then compare the sorted arrays for equality.
<?php
$arr1 = array(4, 5, 'hello', 2.45, 3.56);
$arr2 = array(5, 2.45, 'hello', 3.56, 4);
// Sort the array elements
sort($arr1);
sort($arr2);
// Check for equality
if ($arr1 == $arr2)
echo "Both arrays are same, when sorted";
else
echo "Both arrays are not same, even when sorted";
?>
Output:
Both arrays are same, when sorted
Use the array_diff()
Function to Compare Two Arrays and Return the Difference in PHP
We can compare two arrays and return the difference between the two arrays, and do so, we use the array_diff()
function. With this function, we can compare two arrays and expect a return array that contains elements in the first array that are not in the second array.
<?php
$first = array("12", 34, true, 45, 67);
$second = array("12", 21, 89, 45);
print_r(array_diff($first, $second));
?>
Output:
Array
(
[1] 34
[2] 1
[4] 67
)
true
You have to know the order you place the arrays in the array_diff()
functions impacts that return an array. With the same code, if we change the order of the arrays, the output below is the result.
Array
(
[1] 21
[2] 89
)
true
And if there is no difference, the code will return an empty array.
Use the array_intersect()
Function to Compare Two Arrays and Return the Matches in PHP
With the array_intersect()
function, you can compare two arrays and return the elements that are present in both elements. Using the same two arrays in the previous section, you will find 12
and 45
in the return array.
<?php
$first = array("12", 34, true, 45, 67);
$second = array("12", 21, 89, 45);
print_r(array_intersect($first, $second));
?>
Output:
Array
(
[0] 12
[3] 45
)
true
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn