How to Combine Two Arrays in PHP
-
Use the
array_merge()
Function to Combine Two Arrays in PHP -
Use the
+
Operator to Combine Two Arrays in PHP
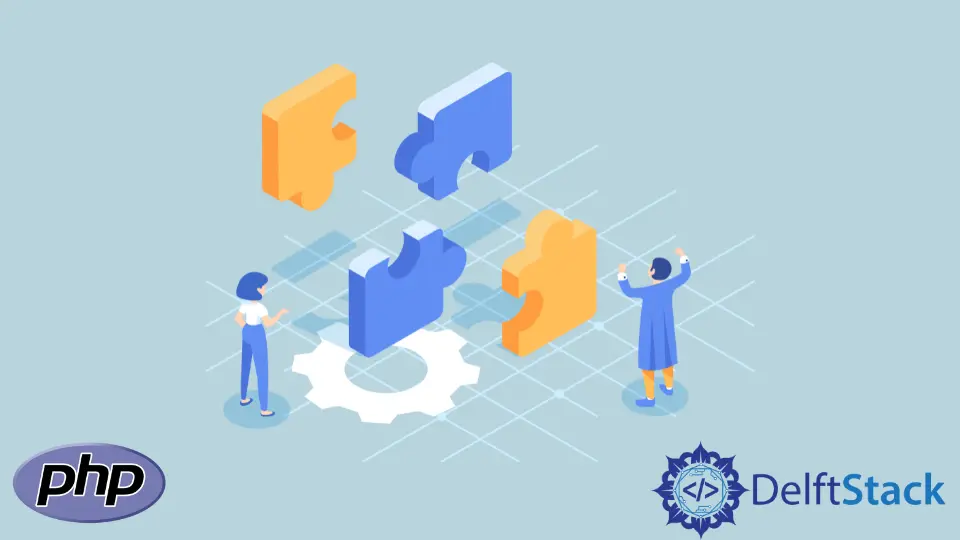
This article will introduce different methods to combine two arrays in PHP.
Use the array_merge()
Function to Combine Two Arrays in PHP
We can use the array_merge()
function to combine two arrays. This function merges two or more arrays. If the input arrays have the same string keys, then the later value for that key will overwrite the previous one. If the arrays contain numeric keys, then the latter value will not overwrite the original value but will be appended. The correct syntax to use this function is as follows.
array_merge($array1, $array2, $array3, ..., $arrayN);
The array_merge()
function has N parameters. The details of its parameters are as follows.
Variables | Description |
---|---|
$array1 , $array2 , $array3 , …, $arrayN |
Arrays to be merged. |
This function returns the merged array. The program below shows how we can use the array_merge()
function to combine two arrays in PHP.
<?php
$array1=array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$array2=array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy");
$output = array_merge($array1, $array2);
var_dump($output);
?>
Output:
array(14) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(5) "Tulip"
[5]=>
string(10) "Sun Flower"
[6]=>
string(8) "Daffodil"
[7]=>
string(5) "Daisy"
[8]=>
string(4) "Rose"
[9]=>
string(4) "Lili"
[10]=>
string(7) "Jasmine"
[11]=>
string(8) "Hibiscus"
[12]=>
string(8) "Daffodil"
[13]=>
string(5) "Daisy"
}
The function has returned the merged $output
array.
Use the +
Operator to Combine Two Arrays in PHP
We can also use the sum operator +
to combine two arrays in PHP. The correct syntax to use this operator is as follows.
$output = $array1 + $array2 + $array3 + ... + $arrayN;
This is one of the simplest methods to combine two arrays. The output array does not contain any duplicate values. The program below shows how we can use the +
operator to combine two arrays in PHP.
<?php
$array1=array( "Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$array2=array(
"Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy"
);
$output = $array1 + $array2;
var_dump($output);
?>
Output:
array(8) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(5) "Tulip"
[5]=>
string(10) "Sun Flower"
[6]=>
string(8) "Daffodil"
[7]=>
string(5) "Daisy"
}