PHP Array Sum
-
Use the
array_sum()
Function to Sum the Values of an Array in PHP - Calculate the Sum of All Values of a Multidimensional Array in PHP
-
Calculate the Sum of a Particular Column of a Multidimensional Array Using
sum_array()
Function in PHP
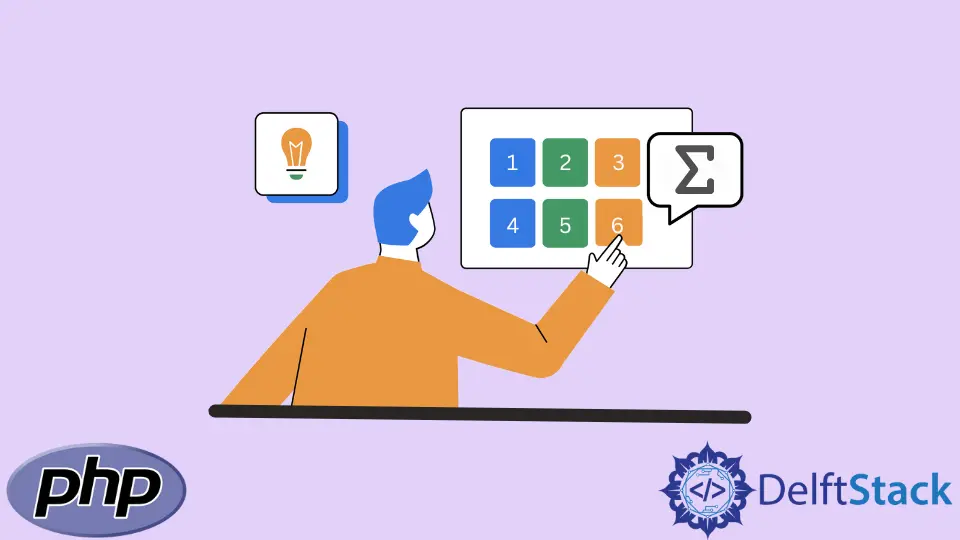
PHP has a built-in function array_sum()
, which is used to sum all the values of an array.
This function takes one parameter, the array you want to sum values. It only takes a one-dimensional and associative array as a parameter.
The array_sum()
returns an integer or float value after adding all the values; if the array is empty, it will return 0
.
This tutorial demonstrates the uses of array_sum()
and how to sum the values of a multidimensional array.
Use the array_sum()
Function to Sum the Values of an Array in PHP
The array_sum()
can only be used for one-dimensional and associative arrays.
// Sum of the values of a one dimensional array.
$sum_array=array(10,20,30,40,50);
echo "Sum of the one-dimensional array is: "
echo array_sum($sum_array);
echo "<br>";
// Sum of the values of one associative array.
$sum_array=array("val1"=>10.5,"val2"=>20.5,"val3"=>30.5,"val4"=>40.5,"val5"=>50.5);
echo "Sum of the associative array is: "
echo array_sum($sum_array);
The above code will sum the values of the corresponding array. In the case of an associative array, the array_sum()
will only sum the values, not the keys.
Output:
Sum of the one-dimensional array is: 150
Sum of the associative array is: 152.5
Calculate the Sum of All Values of a Multidimensional Array in PHP
We can use foreach loops for a multidimensional array to calculate the sum of all values.
<?php
$multi_array = [
["val1"=>10,"val2"=>20,"val3"=>30,"val4"=>40,"val5"=>50],
["val1"=>10.5,"val2"=>20.5,"val3"=>30.5,"val4"=>40.5,"val5"=>50.5],
["val1"=>60,"val2"=>70,"val3"=>80,"val4"=>90,"val5"=>100],
["val1"=>60.5,"val2"=>70.5,"val3"=>80.5,"val4"=>90.5,"val5"=>100.5]
];
$sum_array = array();
foreach ($multi_array as $k=>$sub_array) {
foreach ($sub_array as $id=>$val) {
$sum_array[$id]+=$val;
}
}
print "Sum of full Array : ".array_sum($sum_array)."<br/>";
?>
The code above will first calculate the sum of columns of a multidimensional array and return an array with the sum of columns values. Then we use sum_array()
function.
Output:
Sum of full Array : 1105
Calculate the Sum of a Particular Column of a Multidimensional Array Using sum_array()
Function in PHP
As we know, sum_array()
cannot return the sum of a multidimensional array, but it can be used with another built-in function, array_column()
.
The array_column()
will return an array with keys and values with a column from the given multidimensional array. It takes two mandatory parameters, the array and column or index key.
<?php
$multi_array = [
["val1"=>10,"val2"=>20,"val3"=>30,"val4"=>40,"val5"=>50],
["val1"=>10.5,"val2"=>20.5,"val3"=>30.5,"val4"=>40.5,"val5"=>50.5],
["val1"=>60,"val2"=>70,"val3"=>80,"val4"=>90,"val5"=>100],
["val1"=>60.5,"val2"=>70.5,"val3"=>80.5,"val4"=>90.5,"val5"=>100.5]
];
$sum_val1 = array_sum(array_column($multi_array, "val1"));
print "Sum of column val1 : ".$sum_val1."<br/>";
$sum_val2 = array_sum(array_column($multi_array, "val2"));
print "Sum of column val2 : ".$sum_val2."<br/>";
$sum_val3 = array_sum(array_column($multi_array, "val3"));
print "Sum of column val3 : ".$sum_val3."<br/>";
$sum_val4 = array_sum(array_column($multi_array, "val4"));
print "Sum of column val4 : ".$sum_val4."<br/>";
$sum_val5 = array_sum(array_column($multi_array, "val5"));
print "Sum of column val5 : ".$sum_val5."<br/>";
?>
Output:
Sum of column val1 : 141
Sum of column val2 : 181
Sum of column val3 : 221
Sum of column val4 : 261
Sum of column val5 : 301
We can also use loops with array_sum
and array_column()
functions to calculate the sum of all values of a multidimensional array.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook