How to Print Array Keys in PHP
-
Print Array Keys With
foreach
andarray_keys
in PHP -
Print Array Keys With
foreach
Key-Value Pairs in PHP - Print Array Keys via a Search Value in PHP
- Print Array Keys via Strict Value Search in PHP
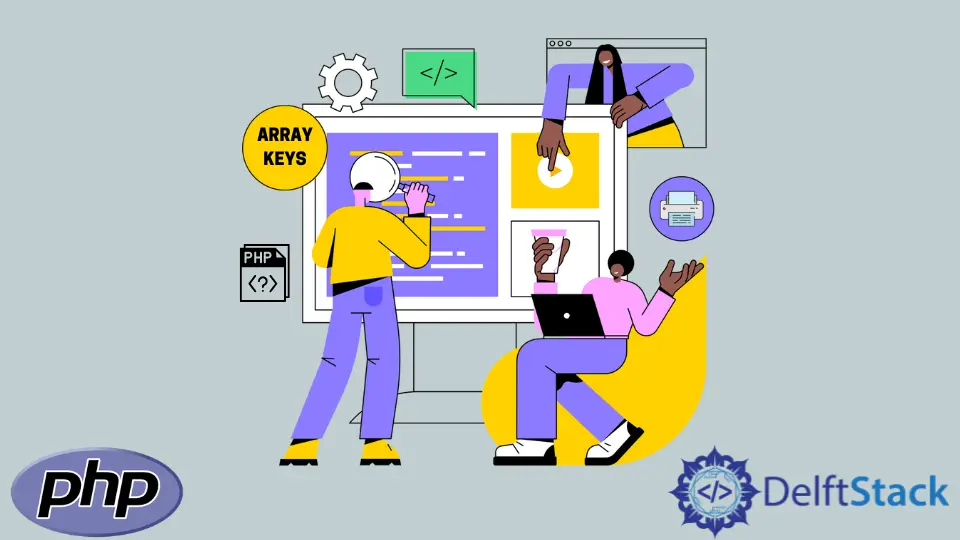
This tutorial will teach you different methods that you can use to print the keys
of an array. These methods will use PHP foreach
and array_keys
, a built-in PHP function.
Print Array Keys With foreach
and array_keys
in PHP
In this approach, we loop through the array with foreach
. During the loop, we call array_keys
on the array, which will extract the keys from the array.
We can pass the keys to a variable in the foreach
loop. Afterward, we extract the keys with foreach
and array_keys
.
<?php
$history_makers = array(
'Father USA' => 'Benjamin Franklin',
'Electromagnetism' => 'Michael Faraday',
'Enlightenment' => 'Issac Newton',
'Tesla Coil' => 'Nikola Tesla',
'Polonium' => 'Marie Curie'
);
// Use array_keys to return all the keys
// in the array
foreach (array_keys($history_makers) as $key) {
echo $key . "<br />";
}
?>
Output:
Father USA <br />
Electromagnetism <br />
Enlightenment <br />
Tesla Coil <br />
Polonium <br />
Print Array Keys With foreach
Key-Value Pairs in PHP
The foreach
loop with its key-value lets you map a key in the array to its value. You can extract and print the array keys during this process.
We print the array keys via the $key
variable in the foreach
loop in the code.
<?php
$history_makers = array(
'Father USA' => 'Benjamin Franklin',
'Electromagnetism' => 'Michael Faraday',
'Enlightenment' => 'Issac Newton',
'Tesla Coil' => 'Nikola Tesla',
'Polonium' => 'Marie Curie'
);
// Use key-value mapping to return the keys
// in the array
foreach ($history_makers as $key => $value) {
echo $key . "<br />";
}
?>
Output:
Father USA <br />
Electromagnetism <br />
Enlightenment <br />
Tesla Coil <br />
Polonium <br />
Print Array Keys via a Search Value in PHP
You can pass a string value as the second parameter to the array_keys
function. When you do this, array_keys
consider this value a needle.
As a result, it will search for every key with this value in the array. It’ll return a list of all keys that match the criteria.
<?php
$history_makers = array(
'Father USA' => 'Benjamin Franklin',
'Electromagnetism' => 'Michael Faraday',
'Enlightenment' => 'Issac Newton',
'Tesla Coil' => 'Nikola Tesla',
'Polonium' => 'Marie Curie'
);
/**
* A string second parameter tells array_keys
* to return arrays containing the parameter
*/
$needle = array_keys($history_makers, 'Marie Curie');
echo "<pre>";
var_dump($needle);
echo "</pre>";
?>
Output:
array(1) {
[0]=>
string(8) "Polonium"
}
We search for the Marie Curie
value in the code sample above. The result is an array with matching keys.
Print Array Keys via Strict Value Search in PHP
This approach builds on returning array keys via a search value, but we’ll perform a strict search in this case. This strict search means that any value that we pass to the array_keys
function must match a value in the array case-for-case.
In this method, you’ll need to pass the third parameter, which is a Boolean value, to array_keys
. Array_keys
perform a strict comparison when you set this Boolean value to true
.
<?php
$history_makers = array(
'Father USA' => 'Benjamin Franklin',
'Electromagnetism' => 'Michael Faraday',
'Enlightenment' => 'Issac Newton',
'Tesla Coil' => 'Nikola Tesla',
'Polonium' => 'Marie Curie'
);
/**
* Here, we use array_keys with a third
* parameter. The third parameter is a
* Boolean value that array_key uses for
* strict comparisons. If set to true,
* the search value must match a value
* in the array case-for-case.
*/
$needle_strict = array_keys($history_makers, 'marie curie', true);
if ($needle_strict) {
// This code block will not print anything because
// in strict comparisons "marie curie" is not
// equal to "Marie Curie"
echo "<pre>";
var_dump($needle_strict);
echo "</pre>";
} else {
echo "You have strict search enabled for
<code>array_keys</code>.<br/>
Change your search needle and try again.";
}
?>
Output:
You have strict search enabled for <code>array_keys</code>. <br/>
Change your search needle and try again.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn