How to Check if Key Exists in Array in PHP
-
Check if Key Exists in PHP Array Using the
array_key_exists()
Function -
Check if Key Exists in PHP Array Using the
isset()
Function -
array_key_exists()
vsisset()
in PHP
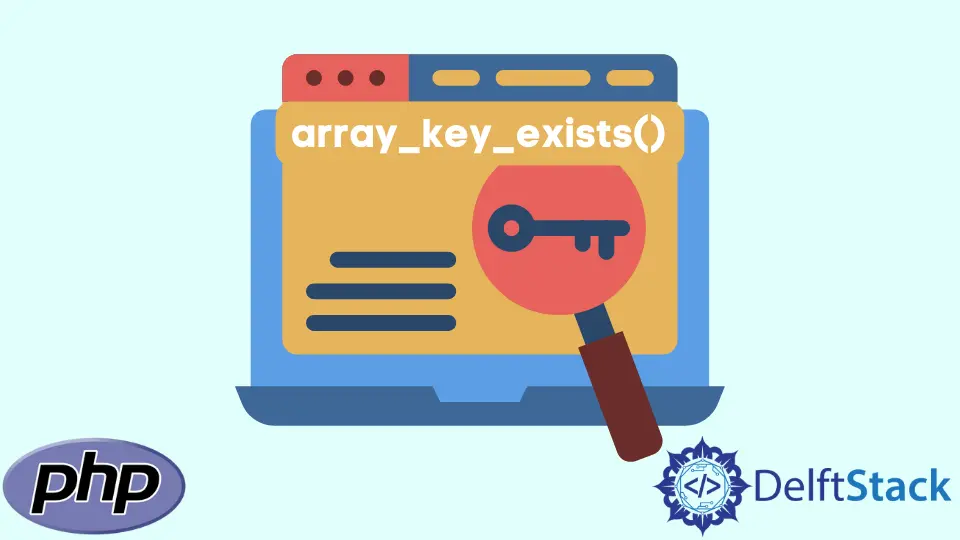
An array is a single variable in PHP which holds many elements inside it. Each element stored inside the array has a unique index like the primary key in DB assigned to it. You can access the element of an array using that index. Your script may need the check if a particular key exists or not to perform an operation on value. In this tutorial article, we will discuss how to check if a specific key exists or not in your array.
PHP supports three types of arrays:
- Indexed arrays - Arrays with a numeric index, where only values are provided. Eg.
array(1,2,3)
. - Associative arrays - Arrays with named keys, where the key is also defined along with value like a JSON object. Eg.
array("first" => 1, "second" => 2)
. - Multidimensional arrays - Arrays containing one or more nested arrays. Eg.
array(array("a", "b", "c"), array("d", "e", "f"), array("g", "h", "i"))
.
PHP provides two ways to find out the array contains a key or not. First, we will understand those two methods and then compare them to get our result.
Check if Key Exists in PHP Array Using the array_key_exists()
Function
PHP provides in-built function array_key_exists
, which checks if the given key or index exists in the provided array. array_key_exists()
function works with indexed arrays and associative arrays, but nested keys in multidimensional arrays won’t be found. array_key_exists()
will look for the keys within the first dimension only. If no key-value pair exits, then the array considers the numeric keys as default keys starting from zero.
Syntax of array_key_exists()
array_key_exists(string|int $key, array $array): bool
Parameters
$key (mandatory)
: This parameter refers to the key/index needed to be searched for in an input array.$array (mandatory)
: This parameter refers to the original array in which we want to search the given key/index$key
.
Return Values
It returns true if key/index is found or false if key/index is not found.
Example Code
<?php
$search_array = array('first' => 1, 'second' => 2);
if (array_key_exists('first', $search_array)) {
echo "The 'first' element is found in the array";
} else {
echo "Key does not exist";
}
?>
Output:
The 'first' element is found in the array
Check if Key Exists in PHP Array Using the isset()
Function
PHP provides function isset()
, which determines if a variable is set; this means if a variable is declared and assigned value other than null. isset()
will return false when a variable has been assigned to null.
Syntax of isset()
isset(mixed $var, mixed ...$vars): bool
You can pass many parameters, if many parameters are supplied, then isset()
will return true only if all the passed parameters are all set. PHP evaluates from left to right and stops as soon as an unset variable is encountered.
Parameters
$var
: The first variable to be checked.$vars
: Further variables to be checked.
Return Values
It returns true if the variable exists and has any value aside from null, otherwise false.
Example Code
<?php
$search_array = array('first' => 1, 'second' => 2);
if (isset($search_array['first'])) {
echo "The 'first' element is found in the array";
} else {
echo "Key does not exist";
}
?>
Output:
The 'first' element is found in the array
array_key_exists()
vs isset()
in PHP
isset()
does not return true
for array keys that correspond to a null
value, while array_key_exists()
does return true
.
<?php
$search_array = array('first' => null, 'second' => 2);
echo isset($search_array['first']) ? "true" : "false";
echo "\n";
echo array_key_exists('first', $search_array) ? "true" : "false";
?>
Output:
false
true
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn