How to Check if Array Contains a Value in PHP
-
Use
in_array()
to Check if the Array Contains a Value in PHP -
Use
foreach
Loop to Check if the String Contains a Value From an Array in PHP -
Use
strpos()
andjson_encode()
to Check if String Contains a Value From an Array in PHP
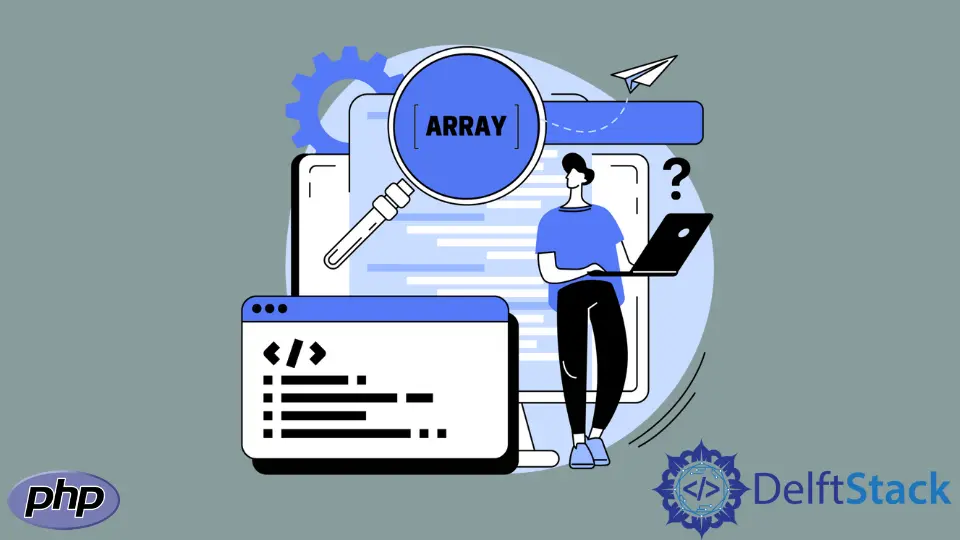
PHP has a few ways to check if an array contains a particular value. The most convenient way is to use the built-in function in_array()
.
in_array()
checks if the array contains a given value. The return type for in_array()
is a boolean.
in_array()
will not look for substrings in the array; the value should match the value in the array. There are other ways to check if the array contains a substring.
This tutorial explores different ways of checking if the array contains the value we are looking for.
Use in_array()
to Check if the Array Contains a Value in PHP
PHP in_array()
takes two mandatory and one optional parameter, the required parameters are the value $value
and the array $array
, and the optional is a boolean $mode
if the boolean is set to true the in_array()
will look for the same type of data.
Example:
<?php
$values = array("demo1", "demo2",10);
if (in_array("10", $values)){
echo "Array contains the value<br>";
}
else{
echo "Array doesn't contains the value<br>";
}
if (in_array("10",$values, TRUE)){
echo "Array contains the value<br>";
}
else{
echo "Array doesn't contains the value<br>";
}
if (in_array(10,$values)){
echo "Array contains the value<br>";
}
else{
echo "Array doesn't contains the value<br>";
}
?>
The above code check if the value 10 exists in the given array. The third default parameter is false, and if we set it to true, it will also look for the same type of value.
Output:
Array contains the value
Array doesn't contains the value
Array contains the value
PHP in_array()
is compatible with all PHP4 and above versions.
Use foreach
Loop to Check if the String Contains a Value From an Array in PHP
The functions foreach
loop and strpos()
can be used together to check if the string contains a value from the array.
Example:
<?php
$values_array = array('John', 'Michelle', 'Shawn');
$demo_str = 'my name is Shawn';
foreach ($values_array as $val) {
if (strpos($demo_str, $val) !== FALSE) {
echo "The string contains a value from the array";
return true;
}
}
echo "The string doesn't contain a value from the array";
return false;
?>
strpos()
checks if the string contains a substring, this code will check if the string contains a value from the given array.
Output:
The string contains a value from the array
PHP strpos()
is compatible with all PHP4 and above versions.
Use strpos()
and json_encode()
to Check if String Contains a Value From an Array in PHP
json_encode()
is a built-in PHP function to convert the array into a JSON
object, in simple words into a string.
Example:
$values_array = ["John is 25 years old", "Shawn", "Michelle"];
if (strpos(json_encode($values_array),"John") !== false) {
echo "Array contains the Name";
}
else{
echo "Array doesn't contains the Name";
}
The above code converts the array into and string and checks if it contains a substring.
Output:
Array contains the Name
PHP jason_encode()
is compatible with all PHP5 and above versions.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook