How to Concat PHP Arrays
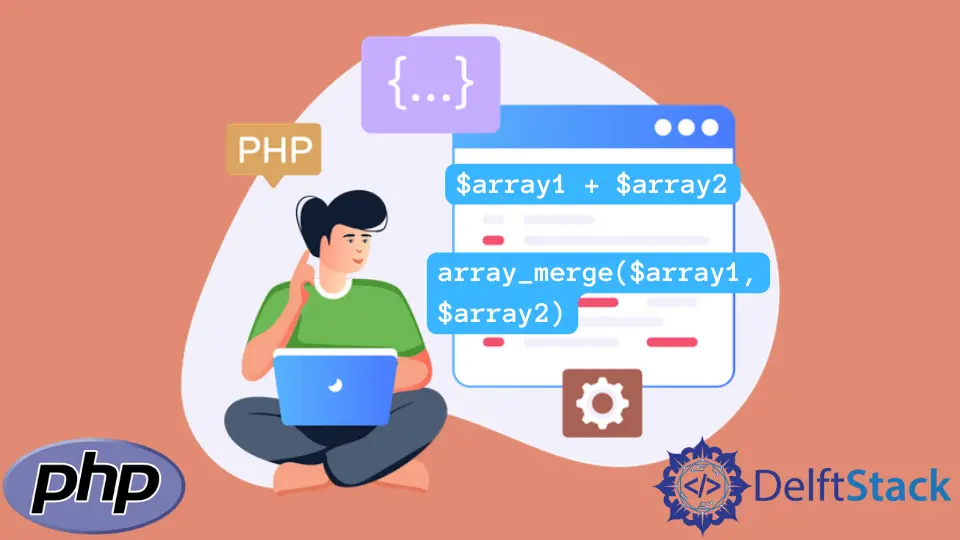
PHP has two ways to concatenate arrays; one is the simple union +
, and the other way is the built-in function array_merge()
.
The concat append the members of the given array at the end of the first array.
This tutorial demonstrates how to concatenate two or more arrays in PHP.
Use the Simple Union +
to Concat Arrays in PHP
Array union can concat two or more arrays, but the arrays should be associative arrays. The union cannot concat simple arrays.
<?php
//Simple one dimensional array
$demo_array1 = array('Jack', 'Shawn', 'Michelle');
$demo_array2 = array('John', 'Joey', 'Maria');
//associative array
$ac_array1 = array(1 => 'Jack', 2 => 'Shawn', 3 => 'Michelle');
$ac_array2 = array(4 => 'John', 1 => 'Joey', 6 => 'Maria');
$combined_array1 = $demo_array1 + $demo_array2;
$combined_array2 = $ac_array1 + $ac_array2;
echo "The values for simple array: <br>";
foreach($combined_array1 as $value){
echo $value."<br>";
}
echo "The values for associative array: <br>";
foreach($combined_array2 as $value){
echo $value."<br>";
}
?>
The code above first tries to concat two simple and associative arrays.
If the key in associative is the same at any point, that element will not be added to the new array.
Output:
The values for simple array:
Jack
Shawn
Michelle
The values for associative array:
Jack
Shawn
Michelle
John
Maria
As we can see union didn’t concat two simple arrays and, in associative, deleted the member with the same key.
Use the array_merge()
to Concat Arrays in PHP
The array_merge
is a built-in function in PHP to merge multiple arrays. The parameters can be as many arrays as you want to concat.
<?php
//Simple one dimensional array
$demo_array1 = array('Jack', 'Shawn', 'Michelle');
$demo_array2 = array('John', 'Joey', 'Maria');
$demo_array3 = array('Robert', 'Jimmy', 'Mike');
//associative array
$ac_array1 = array(1 => 'Jack', 2 => 'Shawn', 3 => 'Michelle');
$ac_array2 = array(4 => 'John', 5 => 'Joey', 6 => 'Maria');
$ac_array3 = array(7 => 'Robert', 1 => 'Jimmy', 8 => 'Mike');
// concat arrays using array_merge
$combined_array1 = array_merge($demo_array1 , $demo_array2, $demo_array3) ;
$combined_array2 = array_merge($ac_array1 , $ac_array2, $ac_array3) ;
echo "The values for simple array: <br>";
foreach($combined_array1 as $value){
echo $value."<br>";
}
echo "The values for associative array: <br>";
foreach($combined_array2 as $value){
echo $value."<br>";
}
?>
The code above tries to concat a set of three simple arrays and a set of three associative arrays.
The array_merge()
will concat arrays irrespective of their type. The array_merge()
will keep all the elements, unlike the union.
Output:
The values for simple array:
Jack
Shawn
Michelle
John
Joey
Maria
Robert
Jimmy
Mike
The values for associative array:
Jack
Shawn
Michelle
John
Joey
Maria
Robert
Jimmy
Mike
As the output shows, no single member is deleted while concatenating the arrays.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook