How to Add Elements to an Array in PHP
-
Use the
array_push()
Function to Add Elements to an Array in PHP - Use the Direct Assignment Method to Add Elements to an Array in PHP
-
Use the
array_unshift
Function to Add Elements to an Empty Array in PHP
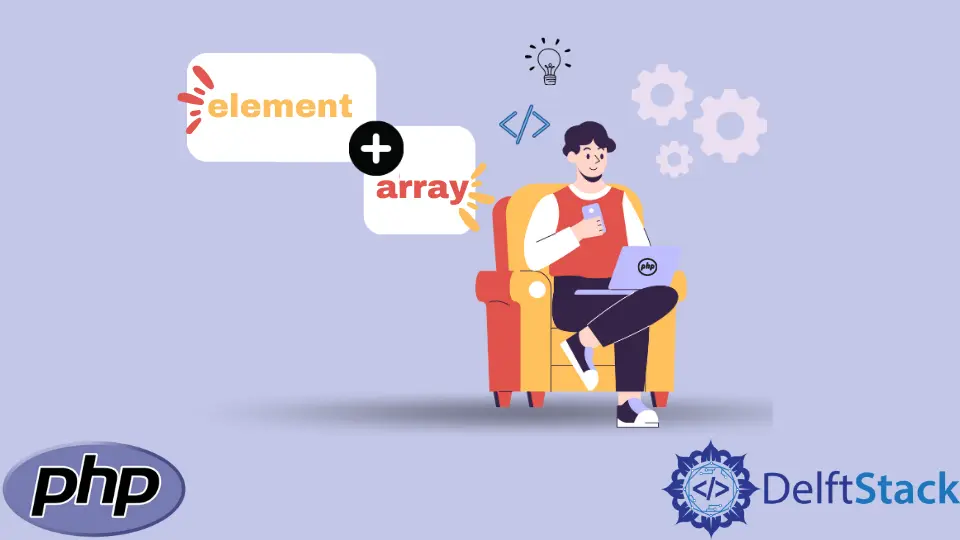
This article will introduce how you can add elements to an array in PHP using different procedures, such as using the array_push()
function and the Direct Assignment Method.
Use the array_push()
Function to Add Elements to an Array in PHP
One way to add elements to an array is to use the array_push
function. First, we will create an array using the array()
function. After that, we will add elements to that array using the given command. The function array_push()
adds elements to the array just like a stack; the correct syntax to execute this is as follows:
array_push($array, $value1, $value2, ..., $valueN);
The built-in function array_push()
has N+1 parameters; N is the number of values we want to add to an array. The details of its parameters are as follows:
Parameters | Description | |
---|---|---|
$array |
mandatory | It’s the array where we’re adding values to. |
$value1 , $value2 , $valueN |
mandatory | These are the values that we’re adding to an array; it can be a string, integer, float, etc. |
This function returns an integer value, which shows the number of elements added to the array. The program below presents how we can use the array_push()
function to add elements to an array in PHP.
<?php
$flowers = array();
echo("The array is empty, as you can see. \n");
print_r($flowers);
echo("Now, we have added the values. \n");
array_push($flowers, "Rose", "Jasmine", "Lili", "Hibiscus", "Tulip");
print_r($flowers);
?>
We have passed 5 values as elements to add to the array.
Output:
The array is empty, as you can see.
Array
(
)
Now, we have added the values.
Array
(
[0] => Rose
[1] => Jasmine
[2] => Lili
[3] => Hibiscus
[4] => Tulip
)
Now, we check the return value of the function, following the syntax:
<?php
$flowers = array();
echo("The array is empty, as you can see. \n");
print_r($flowers);
echo("Now, we have added the values. \n");
echo(array_push($flowers, "Rose", "Jasmine", "Lili", "Hibiscus", "Tulip"));
echo("\n");
print_r($flowers);
?>
Output:
The array is empty, as you can see.
Array
(
)
Now, we have added the values.
5
Array
(
[0] => Rose
[1] => Jasmine
[2] => Lili
[3] => Hibiscus
[4] => Tulip
)
The output shows the value 5, which is the number of the elements added to the array.
Use the Direct Assignment Method to Add Elements to an Array in PHP
In PHP, we can also use the Direct Assignment Method to add elements to an array. We will directly assign values to an array just like we assign values to an integer or string; the correct syntax to execute this is as follows:
$array[] = $value;
The program that applies this method to add elements to an array is as follows:
<?php
$flowers = array();
echo("The array is empty, as you can see. \n");
print_r($flowers);
echo("Now, we have added the values. \n");
$flowers[] = "Rose";
$flowers[] = "Jasmine";
$flowers[] = "Lili";
$flowers[] = "Hibiscus";
$flowers[] = "Tulip";
print_r($flowers);
?>
Output:
The array is empty, as you can see.
Array
(
)
Now, we have added the values.
Array
(
[0] => Rose
[1] => Jasmine
[2] => Lili
[3] => Hibiscus
[4] => Tulip
)
Use the array_unshift
Function to Add Elements to an Empty Array in PHP
In PHP, we can also use the array_unshift()
function to add elements to an array. This function adds values at the beginning of the array. The correct syntax to assign a value to an array is as follows:
array_unshift($array, $value1, $value2, ..., $valueN);
The built-in function array_unshift()
has N+1 parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$array |
mandatory | It is the array in which we want to add the values. |
$value1 , $value2 , $valueN |
mandatory | It is the value or values that we want to add. At least one value is mandatory. |
The program that applies this function to add elements to an array is as follows:
<?php
$flowers = array();
echo("The array is empty as you can see. \n");
print_r($flowers);
echo("Now we have added the values. \n");
echo(array_unshift($flowers, "Rose", "Jasmine", "Lili", "Hibiscus", "Tulip"));
echo("\n");
print_r($flowers);
?>
Output:
The array is empty as you can see.
Array
(
)
Now we have added the values.
5
Array
(
[0] => Rose
[1] => Jasmine
[2] => Lili
[3] => Hibiscus
[4] => Tulip
)