How to Loop Through an Array in PHP
-
Use the
foreach
Loop to Loop Through an Array in PHP -
Use the
for
Loop to Loop Through an Array in PHP
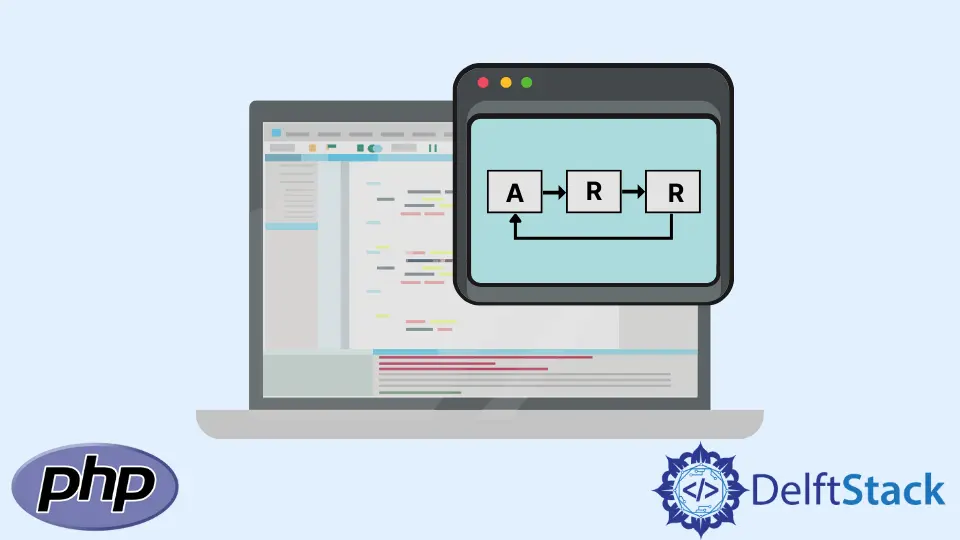
In this article, we will introduce methods to loop through an array in PHP. Using these methods, we will traverse through an array.
- Using the
foreach
loop - Using the
for
loop
Use the foreach
Loop to Loop Through an Array in PHP
We can use a foreach
loop to loop through an array. We can also access array elements using this loop. The correct syntax to use this loop is as follows.
foreach($arrayName as $variableName){
//PHP code
}
If we have an associative array, we can use this loop in the following way:
foreach($arrayName as $key => $variableName){
//PHP code
}
The detail of its parameters is as follows:
Variable | Detail | |
---|---|---|
$arrayName |
mandatory | This is the array we want to traverse. |
$variableName |
mandatory | It is the variable name for array elements. |
$key |
optional | It is the variable name for keys of the array. |
The foreach
loop stops when it traverses the whole array.
We can use the echo()
function to display the array elements.
The program below shows how we can use the foreach
loop to loop through an array.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
foreach($array as $FlowerName){
echo("The flower name is $FlowerName. \n");
}
?>
We have looped through a simple array and displayed its elements.
Output:
The flower name is Rose.
The flower name is Lili.
The flower name is Jasmine.
The flower name is Hibiscus.
The flower name is Tulip.
The flower name is Sun Flower.
The flower name is Daffodil.
The flower name is Daisy.
Now we will loop through an associative array.
<?php
$array = array(
"Flower1"=>"Rose",
"Flower2"=>"Lili",
"Flower3"=>"Jasmine",
"Flower4"=>"Hibiscus",
"Flower5"=>"Tulip",
"Flower6"=>"Sun Flower",
"Flower7"=>"Daffodil",
"Flower8"=>"Daisy");
foreach($array as $key=> $FlowerName){
echo("The $key is $FlowerName. \n");
}
?>
Output:
The Flower1 is Rose.
The Flower2 is Lili.
The Flower3 is Jasmine.
The Flower4 is Hibiscus.
The Flower5 is Tulip.
The Flower6 is Sun Flower.
The Flower7 is Daffodil.
The Flower8 is Daisy.
Use the for
Loop to Loop Through an Array in PHP
We can also use for
loop to traverse through an array. The correct syntax to use for
loop is as follows:
for(initialization, condition, update){
//PHP code
}
The detail of its processes is as follows.
Process | Details | |
---|---|---|
initialization |
mandatory | We initialize the loop counter in this step. |
condition |
mandatory | In this step, we give the condition our loop will iterate. |
update |
mandatory | In this step, we update our counter variable. |
The program that loops through an array using for
loop is as follows:
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$n= sizeof($array);
for($i=0; $i<$n; $i++){
echo("The flower name is $array[$i]. \n");
}
?>
Output:
The flower name is Rose.
The flower name is Lili.
The flower name is Jasmine.
The flower name is Hibiscus.
The flower name is Tulip.
The flower name is Sun Flower.
The flower name is Daffodil.
The flower name is Daisy.