How to Convert an Array to an Object in PHP
- Use Type Casting to Convert an Array to an Object in PHP
-
Use
json_encode()
andjson_decode()
Function to Convert an Array to an Object in PHP
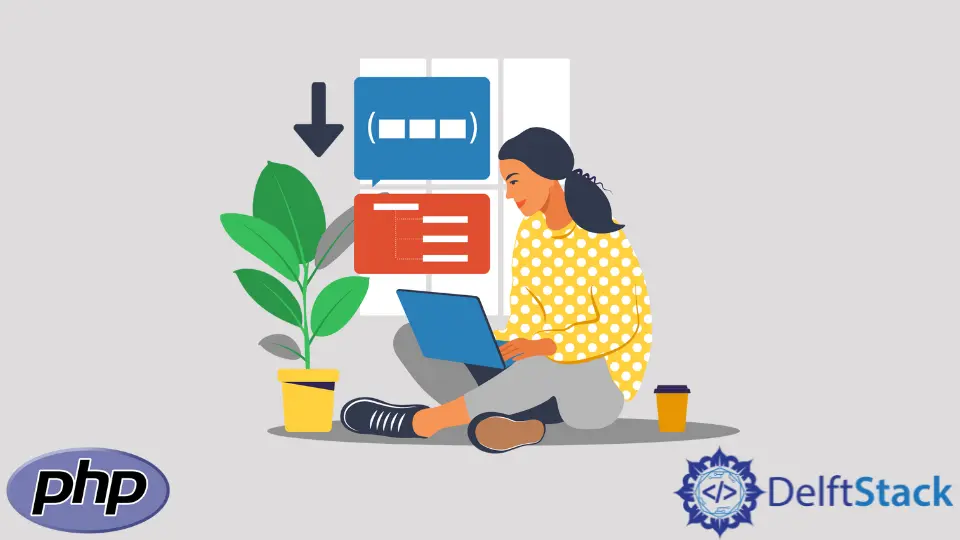
This article introduces methods to convert an array to an object in PHP.
- Using type casting
- Using
json_decode()
andjson_encode()
function
Use Type Casting to Convert an Array to an Object in PHP
Typecasting helps in converting the data type of a variable. We can convert an integer
to a float
, string
, etc using typecasting. Now we will use type casting to convert an array to an object in PHP. The correct method to cast an array to an object is as follows:
$variableName = (object)$arrayName;
The program below shows how we can use type casting to convert an array to an object.
<?php
$array = array("Rose",
"Lili",
"",
"Jasmine",
"Hibiscus",
"Tulip",
"Sun Flower",
"",
"Daffodil",
"Daisy");
$object= (object)$array;
echo("The object is \n");
var_dump($object);
?>
Output:
The object is
object(stdClass)#1 (10) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(0) ""
[3]=>
string(7) "Jasmine"
[4]=>
string(8) "Hibiscus"
[5]=>
string(5) "Tulip"
[6]=>
string(10) "Sun Flower"
[7]=>
string(0) ""
[8]=>
string(8) "Daffodil"
[9]=>
string(5) "Daisy"
}
Use json_encode()
and json_decode()
Function to Convert an Array to an Object in PHP
We can use json_encode()
and json_decode()
functions to convert an array to an object in PHP. The json_encode()
function will convert the array to a JSON string
. Then we will use json_decode()
function to convert this string
to object.
The correct syntax to use json_encode()
function is as follows:
json_encode($variable, $option, $depth)
The function json_encode()
accepts three parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$variable |
mandatory | It is the value that we want to convert to a JSON string . |
$option |
optional | It is the bitmask consisting of multiple constants. You can check these constants here. |
$depth |
optional | It is the depth, it should be greater than zero. |
The correct syntax to use json_decode()
function is as follows:
json_decode($jsonString, $assoc, $depth, $options)
The function json_decode()
accepts four parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$jsonString |
mandatory | It is the JSON string that we want to convert to an object. |
$assoc |
optional | It is a Boolean variable. If set to TRUE, it returns the object as an associative array. |
$depth |
optional | It is the depth, it should be greater than zero. |
$options |
optional | It is the bitmask of JSON_OBJECT_AS_ARRAY, JSON_BIGINT_AS_STRING,, JSON_THROW_ON_ERROR. |
The program that converts an array to an object using these two functions is as follows:
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Tulip","Sun Flower","Daffodil","Daisy");
$object = json_encode($array);
$object1 = json_decode($object);
echo("The object is:\n");
var_dump($object1);
?>
Output:
The object is:
array(8) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(5) "Tulip"
[5]=>
string(10) "Sun Flower"
[6]=>
string(8) "Daffodil"
[7]=>
string(5) "Daisy"
}