How to Hash and Verify Password With Php_hash Method
- Understanding Password Hashing
- Hashing a Password
- Verifying a Password
- Best Practices for Password Hashing
- Conclusion
- FAQ
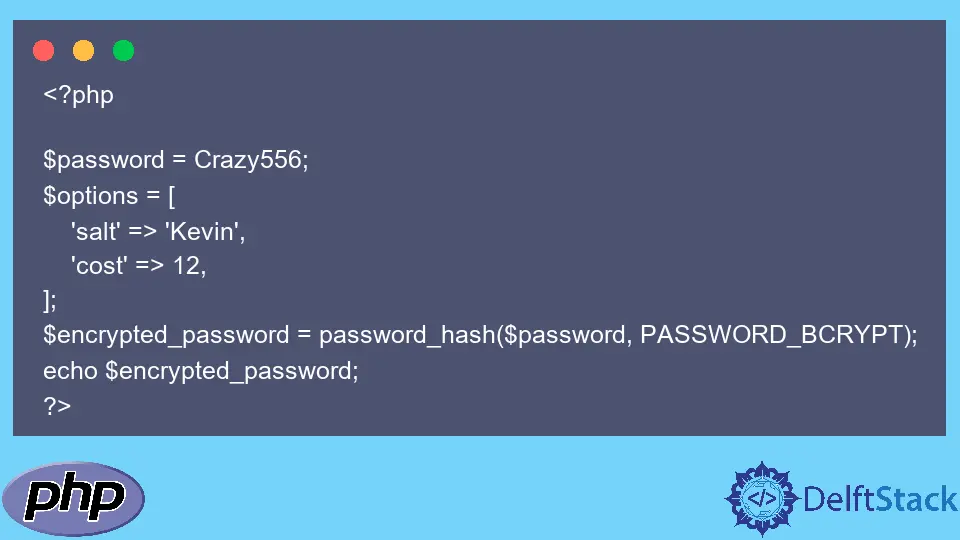
In today’s digital landscape, securing user passwords is more critical than ever. With the increasing number of data breaches, developers must implement robust password hashing techniques to protect user credentials. The PHP hash method is a powerful tool that allows you to securely hash and verify passwords, ensuring that even if a database is compromised, the actual passwords remain safe.
This article will guide you through the process of hashing and verifying passwords using the PHP hash method. Whether you’re a seasoned developer or just starting, you’ll find the information here easy to understand and implement.
Understanding Password Hashing
Before diving into the code, it’s essential to understand what password hashing is and why it’s important. When users create accounts on your platform, they provide passwords that need to be stored securely. Instead of saving these passwords in plain text, which is highly insecure, you should hash them. Hashing transforms the password into a fixed-size string of characters that is nearly impossible to reverse-engineer. This way, even if someone gains access to your database, they cannot retrieve the original passwords.
The PHP hash method provides an easy way to create secure hashes. It uses algorithms like bcrypt, which are designed specifically for hashing passwords. This makes it much more difficult for attackers to crack the passwords, as they would need to compute the hash for every possible password combination.
Hashing a Password
To hash a password in PHP, you can use the password_hash()
function. This function takes the password and an algorithm as parameters, generating a secure hash. Here’s how you can do it:
<?php
$password = "mySecurePassword123";
$hashedPassword = password_hash($password, PASSWORD_BCRYPT);
echo $hashedPassword;
?>
Output:
$2y$10$E9vWq9aQ1s6cF2Zp1cW8Oe8GZy4h1Q7q0tCq5I0w2sP9ZP1yUQZ0u
In this code snippet, we first define a password string. The password_hash()
function is then called with the password and the PASSWORD_BCRYPT
algorithm. This function returns a hashed version of the password, which you can safely store in your database.
When using PASSWORD_BCRYPT
, it applies a salt automatically, which adds an extra layer of security. The generated hash will also include information about the algorithm and the cost factor used, making it easier to verify later.
Verifying a Password
Once you have hashed a password, you need a way to verify it when users log in. The password_verify()
function comes into play here. It checks if the provided password matches the hashed password stored in your database. Here’s how to use it:
<?php
$inputPassword = "mySecurePassword123";
$isPasswordValid = password_verify($inputPassword, $hashedPassword);
if ($isPasswordValid) {
echo "Password is valid!";
} else {
echo "Invalid password.";
}
?>
Output:
Password is valid!
In this example, we simulate a user logging in by providing an input password. The password_verify()
function takes the input password and the hashed password as arguments. If they match, it returns true
, indicating that the password is valid. Otherwise, it returns false
, allowing you to handle invalid login attempts accordingly.
This method is straightforward, and it ensures that you never have to store or compare plain-text passwords, greatly enhancing security.
Best Practices for Password Hashing
While using the PHP hash method is a great start, there are several best practices you should follow to ensure maximum security:
-
Use Strong Passwords: Encourage users to create strong, unique passwords. A strong password typically includes a mix of uppercase and lowercase letters, numbers, and special characters.
-
Regularly Update Algorithms: Security standards evolve, so keep your hashing algorithms up to date. PHP’s
password_hash()
will automatically use the best available algorithm, but it’s good to stay informed. -
Implement Rate Limiting: To prevent brute-force attacks, implement rate limiting on login attempts. This can deter attackers from trying multiple password combinations in a short period.
-
Use HTTPS: Always use HTTPS to encrypt data transmitted between the client and server. This prevents attackers from intercepting sensitive information, including passwords.
By following these best practices, you can significantly enhance the security of your password management system.
Conclusion
Hashing and verifying passwords using the PHP hash method is a crucial step in ensuring the security of user accounts. By utilizing the built-in functions like password_hash()
and password_verify()
, you can effectively protect user credentials from potential threats. Remember to follow best practices and stay informed about the latest security standards to keep your application secure. With the right techniques in place, you can provide a safer experience for your users, building trust and credibility in your platform.
FAQ
-
What is password hashing?
Password hashing is the process of converting plain-text passwords into a fixed-size string of characters, making it difficult to retrieve the original password. -
Why should I use password_hash() in PHP?
The password_hash() function provides a secure way to hash passwords using algorithms like bcrypt, which include built-in mechanisms for salting and cost factors. -
Can I use other hashing algorithms with password_hash()?
Yes, while bcrypt is recommended, you can also use other algorithms supported by PHP, such as Argon2.
-
How do I know if a password is valid after hashing?
You can use the password_verify() function to check if the input password matches the hashed password stored in your database. -
Is it safe to store hashed passwords in my database?
Yes, storing hashed passwords is much safer than storing plain-text passwords. However, ensure you follow best practices for hashing and database security.