How to Filter Arrays in PHP
-
Filter Arrays With
array_intersect_keys
andarray_flip
in PHP -
Filter Arrays With
array_filter
andARRAY_FILTER_USE_KEY
in PHP -
Filter Arrays With
array_filter
andARRAY_FILTER_USE_BOTH
in PHP - Filter Arrays Based on Key Length in PHP
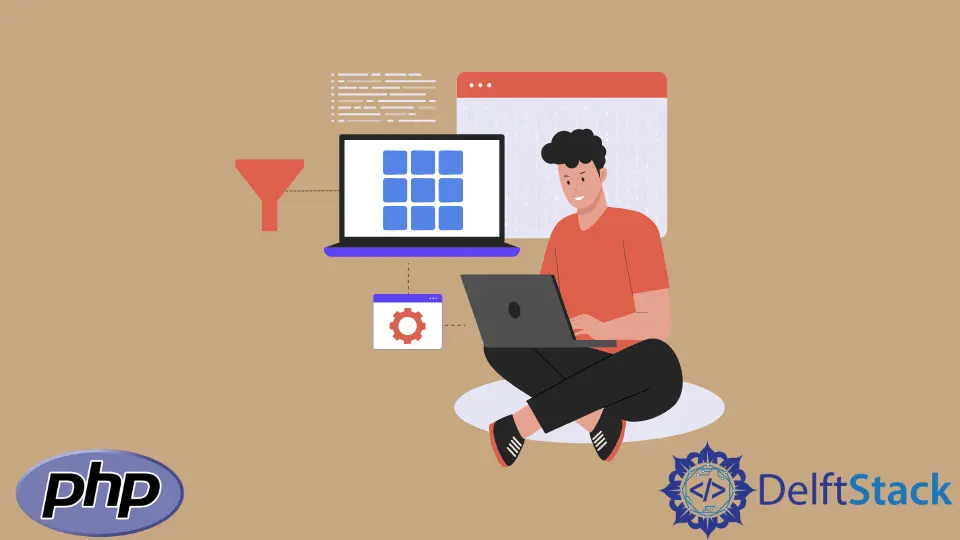
This tutorial will teach different methods you can use to filter arrays in PHP. These methods will use built-in PHP functions like array_intersect_key
, array_flip
, array_filter
and array_keys
.
Filter Arrays With array_intersect_keys
and array_flip
in PHP
The array_intersect_keys
is a PHP built-in function that uses keys to compute the intersection of arrays. It accepts two arrays as parameters
.
The first array should be an array with master keys. While the second array should contain keys that you’ll compare against.
array_flip
is a built-in PHP function. It exchanges all keys with their associated values in an array.
With these two combined, you can filter arrays. First, you create an array that will contain the master keys.
The second array will contain the keys you’ll check against. The following code block shows how to filter arrays with array_intersect_keys
and array_flip
.
Sample:
<?php
$array_master = array (
"author_name" => "John",
"discipline" => "Computer Science",
"Date_of_Birth" => "01/01/1970",
"Programming Languages" => "PHP, Objective-C"
);
$allowed_keys = array(
"author_name",
"discipline"
);
echo "<pre>";
var_dump(array_intersect_key($array_master, array_flip($allowed_keys)));
echo "</pre>";
?>
Output:
array(2) {
["author_name"]=>
string(4) "John"
["discipline"]=>
string(16) "Computer Science"
}
Filter Arrays With array_filter
and ARRAY_FILTER_USE_KEY
in PHP
array_filter
is another built-in function. It uses a callback function to filter elements of an array.
array_filter
function accepts a flag like ARRAY_FILTER_USE_KEY
. This flag will let you filter an array by its keys.
The code block below will provide details on how to use array_filter()
with ARRAY_FILTER_USE_KEY
.
Sample:
<?php
$array_master = array (
"author_name" => "John",
"discipline" => "Computer Science",
"Date_of_Birth" => "01/01/1970",
"Programming Languages" => "PHP, Objective-C"
);
$allowed_keys = array(
"author_name",
"discipline"
);
$filtered = array_filter(
$array_master,
function ($key) use ($allowed_keys) {
return in_array($key, $allowed_keys);
},
ARRAY_FILTER_USE_KEY
);
echo "<pre>";
echo var_dump($filtered);
echo "</pre>";
?>
Output:
array(2) {
["author_name"]=>
string(4) "John"
["discipline"]=>
string(16) "Computer Science"
}
Since PHP 7.4, you can rewrite the previous code with arrow functions:
<?php
$array_master = array (
"author_name" => "John",
"discipline" => "Computer Science",
"Date_of_Birth" => "01/01/1970",
"Programming Languages" => "PHP, Objective-C"
);
$allowed_keys = array(
"author_name",
"discipline"
);
// Here we are using an arrow
// function in array_filter
$filtered = array_filter(
$array_master,
fn ($key) => in_array($key, $allowed_keys),
ARRAY_FILTER_USE_KEY
);
echo "<pre>";
echo var_dump($filtered);
echo "</pre>";
Output:
array(2) {
["author_name"]=>
string(4) "John"
["discipline"]=>
string(16) "Computer Science"
}
Filter Arrays With array_filter
and ARRAY_FILTER_USE_BOTH
in PHP
The ARRAY_FILTER_USE_BOTH
is a flag that you can use with the array_filter()
function. With it, you can pass the value and key to your callback
function.
One of the advantages of this approach is the ability to perform an arbitrary test
against the key.
The next code block shows how to utilize ARRAY_FILTER_USE_BOTH
with array_filter()
.
Sample:
<?php
$array_master = array (
"author_name" => "John",
"discipline" => "Computer Science",
"Date_of_Birth" => "01/01/1970",
"Programming Languages" => "PHP, Objective-C"
);
$allowed_keys = array(
"author_name" => true,
"discipline" => true
);
$filtered = array_filter(
$array_master,
fn ($val, $key) => isset($allowed_keys[$key]) && ($allowed_keys[$key] === true || $allowed_keys[$key] === $val) ,
ARRAY_FILTER_USE_BOTH
);
echo "<pre>";
echo var_dump($filtered);
echo "</pre>";
?>
Output:
array(2) {
["author_name"]=>
string(4) "John"
["discipline"]=>
string(16) "Computer Science"
}
Filter Arrays Based on Key Length in PHP
You can filter an array based on its key length. You can do this with these PHP built-in functions array_filter
, array_keys
, array_intersect_key
, array_flip
, and strlen
.
The array you want to filter should have its keys defined in strings. Also, each string should contain at least three characters long.
As a first step, use a combination of array_filter()
, array_flip
and strlen
functions. Use these functions to return an array that matches your criteria.
Afterward, use array_intersect_key
and array_flip
on the resulting array.
<?php
$array_master = array (
"author_name" => "John",
"discipline" => "Computer Science",
"Date_of_Birth" => "01/01/1970",
"Programming Languages" => "PHP, Objective-C"
);
$filter = array_filter(
array_keys($array_master), function ($key) {
return strlen($key) <= 10;
});
$flip_array = array_intersect_key($array_master, array_flip($filter));
echo "<pre>";
echo var_dump($flip_array);
echo "</pre>";
?>
Output:
array(1) {
["discipline"]=>
string(16) "Computer Science"
}
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn