How to Convert an Array Into a CSV File in PHP
- Method 1: Using fputcsv()
- Method 2: Manual CSV Generation
- Method 3: Using PHP’s Output Buffering
- Conclusion
- FAQ
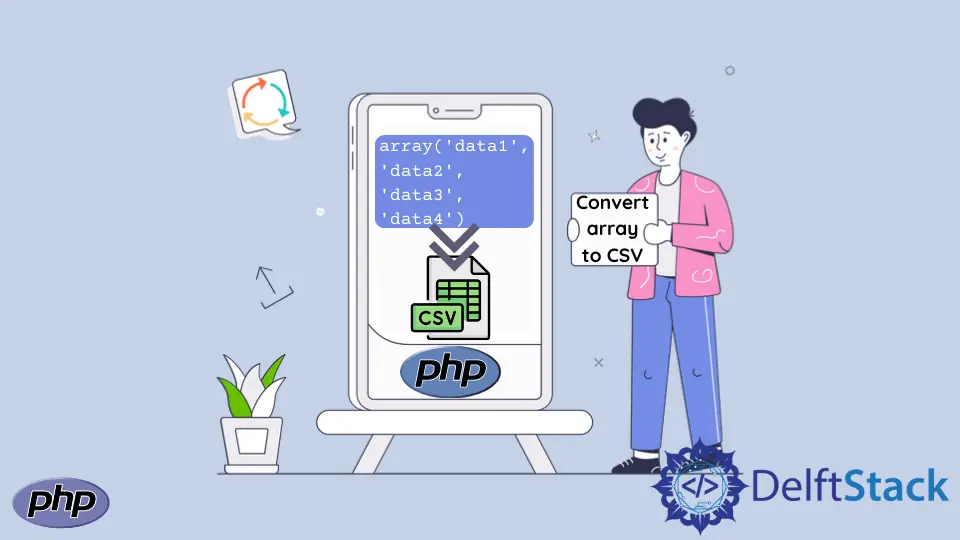
In this tutorial, learn how to convert an array into a CSV file in PHP. CSV (Comma-Separated Values) files are widely used for data exchange and storage. Whether you’re dealing with user data, product inventories, or any other structured information, converting arrays to CSV format can simplify data handling and sharing. PHP provides a straightforward way to accomplish this task.
In this article, we will explore different methods to convert arrays into CSV files, ensuring you have the knowledge to implement this functionality in your projects. Let’s dive into the world of PHP and CSV files!
Method 1: Using fputcsv()
One of the simplest ways to convert an array to a CSV file in PHP is by using the built-in fputcsv()
function. This function takes an array and writes it to a file in CSV format. Below is an example demonstrating how to use fputcsv()
for this purpose.
<?php
$data = [
['Name', 'Age', 'Email'],
['John Doe', 28, 'john@example.com'],
['Jane Smith', 32, 'jane@example.com'],
];
$filename = 'output.csv';
$file = fopen($filename, 'w');
foreach ($data as $row) {
fputcsv($file, $row);
}
fclose($file);
?>
Output:
output.csv created with the array data.
In this code snippet, we first define a multi-dimensional array called $data
. Each inner array represents a row in the CSV file. We then specify the filename for our output file and open it in write mode using fopen()
. By iterating through each row of the array, we use fputcsv()
to write the data to the file. Finally, we close the file with fclose()
. This method is efficient and leverages PHP’s built-in capabilities to handle CSV formatting seamlessly.
Method 2: Manual CSV Generation
While fputcsv()
is convenient, you might prefer to generate the CSV content manually for more control over the output. This method involves concatenating strings and using file operations to create the CSV file. Here’s how you can do it:
<?php
$data = [
['Name', 'Age', 'Email'],
['John Doe', 28, 'john@example.com'],
['Jane Smith', 32, 'jane@example.com'],
];
$filename = 'output_manual.csv';
$file = fopen($filename, 'w');
foreach ($data as $row) {
$line = implode(',', $row) . "\n";
fwrite($file, $line);
}
fclose($file);
?>
Output:
output_manual.csv created with manually generated CSV content.
In this example, we define the same $data
array as before. Instead of using fputcsv()
, we manually construct each line of the CSV by joining the elements of the array with a comma using implode()
. We then append a newline character to separate each row. The fwrite()
function is used to write the constructed line to the file. This method gives you more flexibility to customize the output format if needed.
Method 3: Using PHP’s Output Buffering
Another interesting way to create a CSV file is by utilizing PHP’s output buffering. This technique allows you to write the CSV content directly to the output buffer and then save it as a file. Here’s how to implement this:
<?php
$data = [
['Name', 'Age', 'Email'],
['John Doe', 28, 'john@example.com'],
['Jane Smith', 32, 'jane@example.com'],
];
$filename = 'output_buffer.csv';
ob_start();
$handle = fopen('php://output', 'w');
foreach ($data as $row) {
fputcsv($handle, $row);
}
fclose($handle);
$content = ob_get_clean();
file_put_contents($filename, $content);
?>
Output:
output_buffer.csv created using output buffering.
In this code, we start by defining the $data
array. We then initiate output buffering with ob_start()
. Instead of writing directly to a file, we open php://output
as a file handle. We use fputcsv()
to write each row to the output buffer. After closing the handle, we retrieve the content from the buffer using ob_get_clean()
. Finally, we save the buffered content to a file using file_put_contents()
. This method is particularly useful when you want to manipulate the output before saving it.
Conclusion
Converting an array into a CSV file in PHP is a straightforward process that can be accomplished using various methods. Whether you choose to use the built-in fputcsv()
function, generate the CSV manually, or leverage output buffering, PHP provides the tools you need to handle CSV files effectively. By mastering these techniques, you can streamline data handling in your applications and improve data exchange processes. Start implementing these methods today and enhance your PHP programming skills!
FAQ
-
What is a CSV file?
A CSV file is a text file that uses a comma to separate values, making it easy to store and exchange structured data. -
Can I convert a multidimensional array to CSV in PHP?
Yes, you can convert multidimensional arrays to CSV by iterating through the array and writing each sub-array as a row. -
Is it necessary to use
fputcsv()
for CSV generation?
No, whilefputcsv()
simplifies the process, you can also generate CSV content manually using string manipulation. -
What file permissions are needed to write a CSV file in PHP?
The directory where you are trying to create the CSV file needs to have write permissions for the user running the PHP script. -
How can I handle special characters in CSV files?
You can handle special characters by ensuring they are properly escaped.fputcsv()
automatically takes care of escaping characters like commas and quotes.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook