How to Reverse Array in PHP
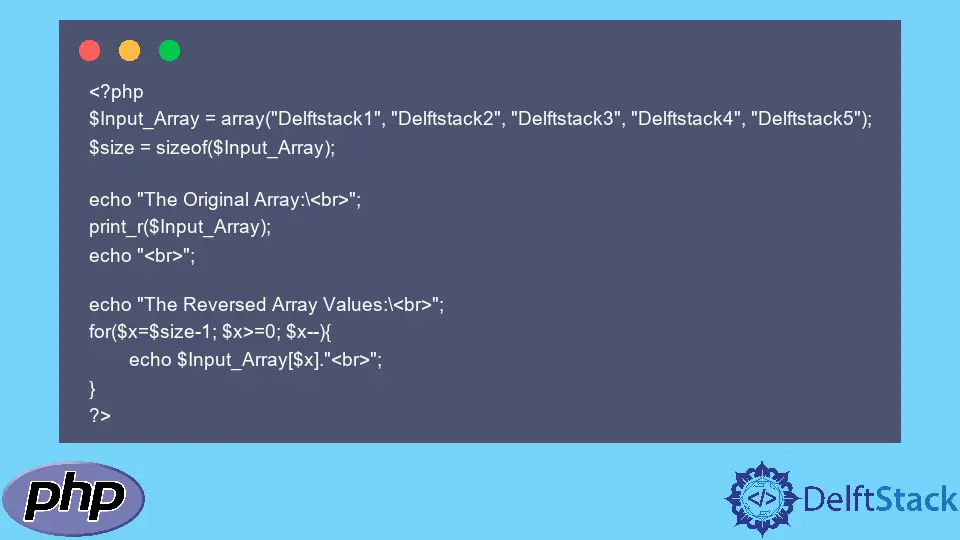
This tutorial demonstrates how to reverse the array in PHP.
Array Reverse in PHP
Reversing an array in PHP is an easy operation done by the built-in function array_reverse()
. This built-in function can reverse an array’s elements, including the nested arrays.
The array_reverse()
also provides functionality to preserve the key elements according to the user. This built-in function will take an array as a parameter and return the reversed array.
The syntax for this method is:
array array_reverse($Input_Array, $Key_to_Preserve)
Where the $Input_Array
is an array that will be reversed which is a mandatory parameter, and the $Key_to_Preserve
is optional, which will notify the method to preserve the keys, this parameter is either true
or false
.
For example, we apply the array_reverse()
method on the following arrays.
Input : $Input_Array = (10, 20, 30, 40, 50)
Output :
Array
(
[0] => 50
[1] => 40
[2] => 30
[3] => 20
[4] => 10
)
Input :
Array
(
[0] => delftstack1
[1] => delftstack2
[2] => delftstack3
[3] => delftstack4
)
Output :
Array
(
[3] => delftstack4
[2] => delftstack3
[1] => delftstack2
[0] => delftstack1
)
Now we know the array_reverse()
method works, let’s try an example in PHP.
<?php
$Input_Array = array("Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5");
echo "The Original Array:<br>";
print_r($Input_Array);
echo "The Array After Reverse:<br>";
print_r(array_reverse($Input_Array));
The code above will reverse the given array using the array_reverse()
method. See output:
The Original Array:
Array (
[0] => Delftstack1
[1] => Delftstack2
[2] => Delftstack3
[3] => Delftstack4
[4] => Delftstack5 )
The Array After Reverse:
Array (
[0] => Delftstack5
[1] => Delftstack4
[2] => Delftstack3
[3] => Delftstack2
[4] => Delftstack1 )
Let’s try the same example by setting the $Key_to_Preserve
parameter as true
. See example:
<?php
$Input_Array = array("Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5");
echo "The Original Array:<br>";
print_r($Input_Array);
echo "The Array After Reverse:<br>";
print_r(array_reverse($Input_Array, true));
Setting the $Key_to_Preserve
as true
will also reverse the keys with the values; the default value for this is false
; that is the first example that didn’t reverse the keys.
See the output:
The Original Array:
Array (
[0] => Delftstack1
[1] => Delftstack2
[2] => Delftstack3
[3] => Delftstack4
[4] => Delftstack5 )
The Array After Reverse:
Array (
[4] => Delftstack5
[3] => Delftstack4
[2] => Delftstack3
[1] => Delftstack2
[0] => Delftstack1 )
The array_reverse()
method is not the only method to reverse an array in PHP; the for
loop can also be used to perform the reverse operation on an array in PHP. Let’s see the example.
<?php
$Input_Array = array("Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5");
$size = sizeof($Input_Array);
echo "The Original Array:\<br>";
print_r($Input_Array);
echo "<br>";
echo "The Reversed Array Values:\<br>";
for ($x=$size-1; $x>=0; $x--) {
echo $Input_Array[$x]."<br>";
}
The code above uses a for
loop to echo
the array values reversely. See the output:
The Original Array:\
Array (
[0] => Delftstack1
[1] => Delftstack2
[2] => Delftstack3
[3] => Delftstack4
[4] => Delftstack5 )
The Reversed Array Values:\
Delftstack5
Delftstack4
Delftstack3
Delftstack2
Delftstack1
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook