Array_map and array_walk Functions in PHP
-
Use PHP
array_map()
Function to Modify Elements in an Array With a User-Defined Function -
Use
array_walk()
to Run an Array With a Function Including Keys in PHP
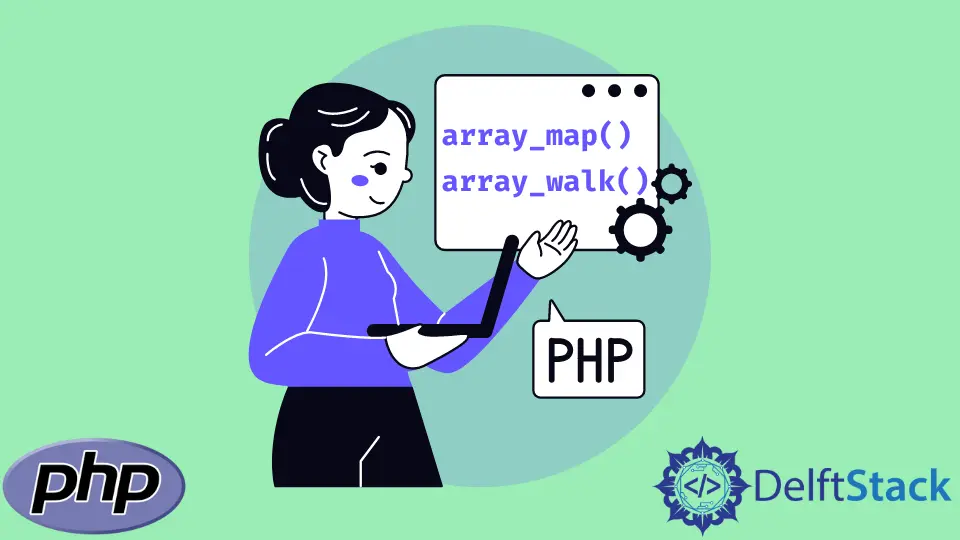
In this tutorial, we will introduce the array_map()
and array_walk()
functions. We will see how you can modify all elements in an array with a user-defined function.
Also, It will cover the different ways you can use the above functions.
Use PHP array_map()
Function to Modify Elements in an Array With a User-Defined Function
You can use the array_map()
function to send all values in an array to a user-defined function.
In the example code below, we send the values in our array $arr
to the function divide
and return the new values.
Example code 1:
<?php
// Define the function divide
function divide($n) {
return($n/$n);
}
$a = array(2, 4, 6, 8, 10);
print_r(array_map("divide", $a));
?>
Output:
Array ( [0] => 1 [1] => 1 [2] => 1 [3] => 1 [4] => 1 )
In the above code, our function divide
divides all the values in the array $a
by the corresponding values; hence the output 1
.
Let’s try using multiple arrays.
Example code 2:
<?php
// Define the function minusAdd
function minusAdd($t, $r, $k) {
return($t - $r + $k);
}
$arr1 = array(28, 14, 76, 38, 10);
$arr2 = array(4, 6, 20, 12, 3);
$arr3 = array(12, 6, 7 ,8 ,9);
print_r(array_map("minusAdd", $arr1, $arr2, $arr3));
?>
Output:
Array ( [0] => 36 [1] => 14 [2] => 63 [3] => 34 [4] => 16 )
As shown in the example below, we can use the array_map()
function to change the elements in an array.
Example code 3:
<?php
function synonym($s) {
if ($s === "Male Horse") {
return "Stallion";
}
return $s;
}
$arr = array("Female Horse", "Male Horse", "Pony");
print_r(array_map("synonym", $arr));
?>
Output:
Array ( [0] => Female Horse [1] => Stallion [2] => Pony )
From the above code, we change the Male Horse
to Stallion
with the help of the array_map
function.
You can also create an array using the array_map
function. For the code to work, you will have to use the null
parameter in the place of the defined function.
Example code 4:
<?php
// Create an array of an array
$a1 = array('Apple', 'Eggplant', 'Cucumber', 'Mango');
$a2 = array('Fruit', 'Vegetable', 'Vegetable', 'Fruit');
$result = array_map( null, $a1, $a2);
print_r($result);
?>
Output:
Array ( [0] => Array ( [0] => Apple [1] => Fruit ) [1] => Array ( [0] => Eggplant [1] => Vegetable ) [2] => Array ( [0] => Cucumber [1] => Vegetable ) [3] => Array ( [0] => Mango [1] => Fruit ) )
Use array_walk()
to Run an Array With a Function Including Keys in PHP
Since the array_map()
function does not handle keys and parameters, we use array_walk()
as shown in the example below.
Example code 6:
<?php
function assign($color, $key) {
echo "The $key is $color <br>";
}
$arr = array("Apple" => "red", "Eggplant" => "purple");
array_walk($arr, "assign");
?>
Output:
The Apple is red
The Eggplant is purple
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn