MATLAB Norm() Function
-
Understanding the
norm()
Function in MATLAB -
Find the Euclidean and Frobenius Norm of a Vector Using the
norm()
Function in MATLAB - Computing Norms for Vectors and Matrices
- Practical Applications
- Additional Options and Considerations
- Conclusion
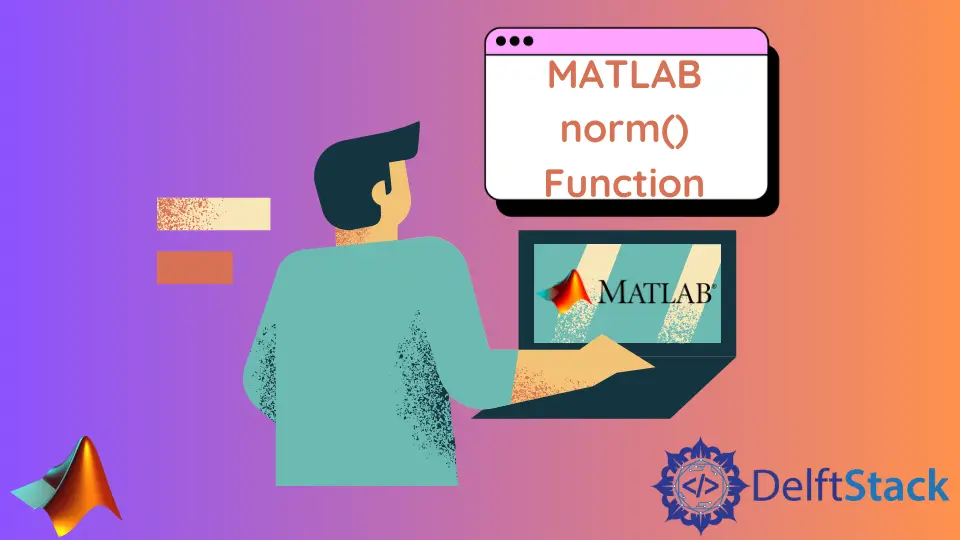
MATLAB, a powerful numerical computing environment widely used in science and engineering, offers a plethora of functions for mathematical operations. One such fundamental function is norm()
, which plays a crucial role in linear algebra, signal processing, and various other mathematical applications.
In this article, we will delve into the details of the norm()
function, exploring its syntax, applications, and options. We will discuss finding the Euclidean and Frobenius norm of a vector or matrix using the norm()
function in MATLAB.
Understanding the norm()
Function in MATLAB
The norm()
function in MATLAB is designed to compute various matrix norms and vector norms. A norm is a mathematical concept that measures the size or length of a mathematical object, such as a vector or a matrix.
Different norms are applicable in different contexts, and MATLAB’s norm()
function provides a versatile tool for handling various norm types.
The basic syntax of the norm()
function is as follows:
n = norm(X, p)
X
: The input matrix or vector for which the norm is calculated.p
: The order of the norm. It can be a specific norm type or an integer specifying the order of the norm. Common choices include:2
: Euclidean norm (default ifp
is not specified).1
: 1-norm (sum of absolute values of elements).inf
: Infinity norm (maximum absolute row sum for matrices, maximum absolute element for vectors).
Find the Euclidean and Frobenius Norm of a Vector Using the norm()
Function in MATLAB
The norm()
function of MATLAB is used to find the Euclidean and Frobenius norm of a vector or matrix. The Euclidean norm is the Euclidean distance of a vector from its origin, which is equal to the magnitude of the vector, 2-norm, or Euclidean length.
The Euclidean distance is equal to the length of a line segment in Euclidean space and between two points. We can also find the Euclidean norm by finding the inner product of a vector with itself and then taking its square root.
The Frobenius norm is the Euclidean norm of a matrix. If we pass a vector inside the norm()
function, it will return the Euclidean norm of that vector, but in the case of a matrix, the norm()
function will return the Frobenius norm.
The norm()
function has five different syntaxes, shown below.
nm = norm(vec)
nm = norm(vec,p)
nm = norm(mat)
nm = norm(mat,p)
nm = norm(mat,"fro")
The first syntax will return the 2-norm or Euclidean norm of the given vector. The second syntax will return the p
-norm of the given vector, in which the p
-norm can be 1-norm, 2-norm, 3-norm, and so on.
The third syntax will return the maximum singularity value or the Euclidean norm of a matrix. The fourth syntax will return the p
-norm of the given matrix, and p
can be 1
, 2
, or Inf
.
If p
is 1
, the syntax will return the maximum absolute sum of columns of the given matrix, and if p
is 2
, the 2-norm will be returned. If p
is Inf
, the syntax will return the maximum absolute sum of rows of the given matrix.
The last syntax will return the Frobenius norm of the given matrix. For example, let’s find the Euclidean norm of a vector and a matrix using the norm()
function.
See the code below:
clc
clear
v1 = 1:5;
m = [1:10;21:30];
n1 = norm(v1)
n3 = norm(m)
Output:
n1 = 7.4162
n3 = 83.201
In the above output, the norm of the whole matrix is returned, which is 83
. If we want to find the norm of each row or column present in a matrix, we can use the vecnorm()
function, which will treat each row or column of the given matrix as a separate vector and compute its norm.
For example, if we pass a matrix inside the vecnorm()
function, it will return a vector containing the 2-norm of each column present in the given matrix. We can also set the norm number as the second argument and the dimension along which we want to take the norm as the third argument inside the vecnorm()
function.
Check this link for more details about the norm()
function. Also, check this link for more details about the vecnorm()
function.
Computing Norms for Vectors and Matrices
for Vectors:
v = [3, -4, 5];
norm_v = norm(v);
This example calculates the Euclidean norm of a vector v
using the default order (2
). The result (norm_v
) would be the square root of the sum of the squares of the vector’s elements.
for Matrices:
A = [1, 2; 3, 4];
norm_A = norm(A, 'fro');
This example calculates the Frobenius norm of a matrix A
using the 'fro'
option. The Frobenius norm is the square root of the sum of the squares of all elements in the matrix.
Practical Applications
1. Error Estimation
Norms are commonly used in error analysis. For example, when comparing two vectors or matrices, the norm of their difference can indicate the error between them.
A = randn(3, 3);
B = randn(3, 3);
error_norm = norm(A - B);
The error_norm
in this case represents the Euclidean norm of the difference between matrices A
and B
.
2. Optimization Problems
In optimization problems, norms are often used as objective functions or constraints. The choice of norm can impact the behavior of the optimization algorithm.
x = fminunc(@(x) norm(x, 1), [1; 2]);
This example minimizes the 1-norm of a vector x
using the fminunc
optimization function.
3. Signal Processing
In signal processing, norms play a role in measuring the magnitude of signals. For instance, the 2-norm is often used to calculate the energy of a signal.
signal = sin(2*pi*0.1*(1:100));
signal_energy = norm(signal, 2);
Here, signal_energy
represents the 2-norm of the signal, providing a measure of its energy.
Additional Options and Considerations
Matrix Norms
For matrices, the norm()
function supports additional norm options beyond the default Euclidean and Frobenius norms. These include the 1-norm, infinity norm, and others.
The choice of a norm depends on the specific requirements of the application.
A = [1, -2; 3, 4];
norm_1 = norm(A, 1); % 1-norm
norm_inf = norm(A, inf); % Infinity norm
Vector Norms
For vectors, the norm()
function can be applied along a specified dimension, allowing for flexibility in calculations.
M = randn(3, 5);
norm_along_columns = norm(M, 2, 1); % 2-norm along columns
norm_along_rows = norm(M, 'fro', 2); % Frobenius norm along rows
Conclusion
MATLAB’s norm()
function is a versatile and powerful tool for calculating norms of vectors and matrices, with various options to suit different applications. Whether used in error estimation, optimization, or signal processing, understanding the available norm types and their implications is crucial for effective utilization.
The examples provided in this guide offer a starting point for incorporating the norm()
function into your MATLAB workflows, providing a deeper insight into the mathematical properties of your data.
Related Article - MATLAB Vector
- How to Remove NaN From Vector in MATLAB
- How to Remove Zeros From a Vector in MATLAB
- 2D Interpolation Using MATLAB
- How to Calculate Cumulative Sum Using MATLAB
- How to Make a Column Vector in MATLAB