How to Calculate Cumulative Sum Using MATLAB
-
Get the Cumulative Sum of a Vector or Matrix Using the
cumsum()
Function in MATLAB - Get the Cumulative Sum of a Vector or Matrix Using a Loop in MATLAB
- Conclusion
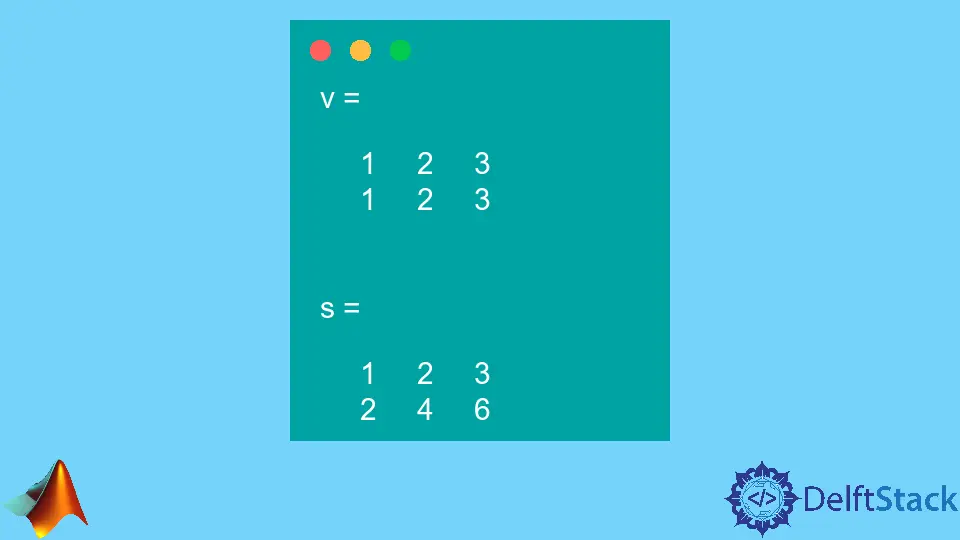
This tutorial will discuss finding the cumulative sum of a vector or matrix using the cumsum()
function and loops in MATLAB.
Get the Cumulative Sum of a Vector or Matrix Using the cumsum()
Function in MATLAB
We can use MATLAB’s built-in function cumsum()
to find the cumulative sum of a vector or matrix. The cumsum()
function is useful for tasks where the running total of elements is required, such as financial calculations, signal processing, and time-series analysis.
The cumsum()
function in MATLAB is a built-in function that calculates the cumulative sum of elements in an array along a specified dimension. The term “cumulative sum” refers to the running total of the elements in the array.
Basic Syntax:
S = cumsum(A)
Parameters:
A
: The input array (vector or matrix) for which the cumulative sum is calculated.S
: The output array containing the cumulative sum of elements along the specified.
By default, cumsum()
operates column-wise on a matrix, providing the cumulative sum for each column. For vectors, it returns the cumulative sum of the entire vector.
The function is versatile and can handle multidimensional arrays as well.
Optional Syntax:
S = cumsum(A, dim)
Where the dim
specifies the dimension along which the cumulative sum is calculated. If omitted, the default is the first non-singleton dimension.
Let’s find the cumulative sum of a vector.
Example Code:
v = 1:8
s = cumsum(v)
Output:
v =
1 2 3 4 5 6 7 8
s =
1 3 6 10 15 21 28 36
In the code above, we create a vector v
ranging from 1
to 9
. By applying the cumsum()
function to this vector, we efficiently calculate the cumulative sum.
Then, the resulting array, stored in s
, exhibits the running total of the vector’s elements. The output reflects the cumulative summation, illustrating each element’s sum with all preceding ones.
Now, let’s find the cumulative sum of a matrix.
Example Code:
v = [1:3; 1:3]
s = cumsum(v)
Output:
v =
1 2 3
1 2 3
s =
1 2 3
2 4 6
In this second example, we construct a 2x3 matrix v
with the first row ranging from 1
to 3
and the second row mirroring the first. Applying the cumsum()
function to the matrix calculates cumulative sums column-wise.
Then, the resulting 2x3 matrix s
reveals the running totals of each column in v
. The output provides a clear representation of the cumulative summation for each column.
We can also find the cumulative sum of rows in a column by passing an integer as a second argument in the cumsum()
function. For example, let’s find the cumulative sum of rows of the above matrix.
Example Code:
v = [1:3; 1:3]
s = cumsum(v,2)
Output:
v =
1 2 3
1 2 3
s =
1 3 6
1 3 6
In this third example, we generate a 2x3 matrix v
with the first row ranging from 1
to 3
and the second row mirroring the first. By using the cumsum()
function with the argument 2
, we perform row-wise cumulative summation.
Then, the resulting 2x3 matrix s
succinctly displays the running totals for each row in the original matrix v
. The output offers a clear overview of cumulative summation across the rows.
We can also reverse the cumulative sum in a vector or matrix. By default, the cumsum()
function finds a cumulative sum starting from the left side of a vector or matrix, but we can also find the cumulative sum starting from the right side of a vector or matrix by using a string reverse inside the cumsum()
function.
For example, let’s find the cumulative sum starting from the right side of a vector.
Example Code:
v = 1:3
s = cumsum(v,'reverse')
Output:
v =
1 2 3
s =
6 5 3
In this fourth example, we generate a vector v
with elements from 1
to 3
. Using cumsum()
with the 'reverse'
argument, we compute the reversed cumulative sum.
The output [6 5 3]
reflects the running total starting from the vector’s last element towards the first, and the cumulative sum starts from the right side. If we have NaN values inside a vector or matrix and find its cumulative sum, the cumsum()
function will turn the output values to NaN as soon as the first NaN value is encountered.
To avoid the NaN value, we can tell the function to ignore the NaN values using the omitnan
string as an argument in the cumsum()
function. The optional argument 'omitnan'
is used to handle NaN (Not a Number) values in the input array, and when 'omitnan'
is specified, the cumsum()
function ignores NaN values during the cumulative sum calculation.
For example, let’s create a vector containing some NaN values and find its cumulative sum using the cumsum()
function.
Example Code:
v = [1 NaN 5 6 7 NaN NaN]
s = cumsum(v,'omitnan')
Output:
v =
1 NaN 5 6 7 NaN NaN
s =
1 1 6 12 19 19 19
In this last example, we create a vector v
with a sequence of elements, including NaN values. Using cumsum()
with the argument 'omitnan'
, we calculate the cumulative sum, excluding NaN values.
The output [1 1 6 12 19 19 19]
reflects the running total while handling NaN values appropriately.
Get the Cumulative Sum of a Vector or Matrix Using a Loop in MATLAB
While MATLAB provides built-in functions like cumsum()
for efficient cumulative sum calculations, you can also implement this operation using loops.
For example, let’s find the cumulative sum of the elements of a vector.
When dealing with a vector, a loop can be employed to iterate through each element, accumulating the sum up to the current index. The loop structure typically involves initializing an array to store cumulative sums and updating it within the loop.
Example Code 1:
v = 1:5;
n = length(v);
cumulativeSum = zeros(1, n);
for i = 1:n
cumulativeSum(i) = sum(v(1:i));
end
disp(cumulativeSum);
Output:
1 3 6 10 15
In this first example, we create a vector v
with elements ranging from 1
to 5
. We then initialize an array cumulativeSum
with zeros to store the cumulative sums.
Next, using a for
loop, we iterate through each element of the vector, progressively calculating the cumulative sum up to the current index and storing the result in the cumulativeSum
array. Finally, we display the array to showcase the cumulative sum of the vector.
The output [1 3 6 10 15]
effectively represents the running total of the elements in the given vector. Now, let’s find the cumulative sum of a matrix (column-wise) using a Loop.
For a matrix, a nested loop structure is often used to iterate through both rows and columns. The outer loop iterates over columns, and the inner loop calculates cumulative sums for each column.
Example Code 2:
A = [1:3; 4:6; 7:9];
[m, n] = size(A);
cumulativeSumMatrix = zeros(m, n);
for j = 1:n
for i = 1:m
cumulativeSumMatrix(i, j) = sum(A(1:i, j));
end
end
disp(cumulativeSumMatrix);
Output:
1 2 3
5 7 9
12 15 18
In this code example, we initialize a matrix A
with three rows. We determine the size of the matrix with size(A)
and store the number of rows in m
and columns in n
.
Next, we create an array cumulativeSumMatrix
filled with zeros to store the cumulative sums. Using nested for
loops, we iterate through each column and row, progressively calculating the cumulative sum for each element and storing it in the cumulativeSumMatrix
array.
Finally, we display the resulting matrix, illustrating the cumulative sum for each column. The output effectively represents the running totals across the matrix columns.
While loops are effective for achieving cumulative sums, MATLAB’s built-in functions, such as cumsum()
, offer optimized and concise alternatives for this specific task. In practice, it’s recommended to use built-in functions like cumsum()
for common operations whenever possible, as they are often more efficient and less error-prone than implementing the logic with explicit loops.
Conclusion
In summary, this tutorial explored two methods for calculating cumulative sums in MATLAB: using the cumsum()
function and employing loops. The cumsum()
function offers an efficient and concise solution for both vectors and matrices, handling various scenarios such as reversing sums and handling NaN values.
On the other hand, while loops provide an alternative approach, the tutorial emphasized the advantages of utilizing built-in functions for improved efficiency and code simplicity.