How to Remove NaN From Vector in MATLAB
-
MATLAB Remove
NaN
Values From Vector Using theisnan()
Function -
MATLAB Remove
NaN
Values From Vector Using thefillmissing()
Function -
MATLAB Remove
NaN
Values From Vector Using thermmissing()
Function - Conclusion
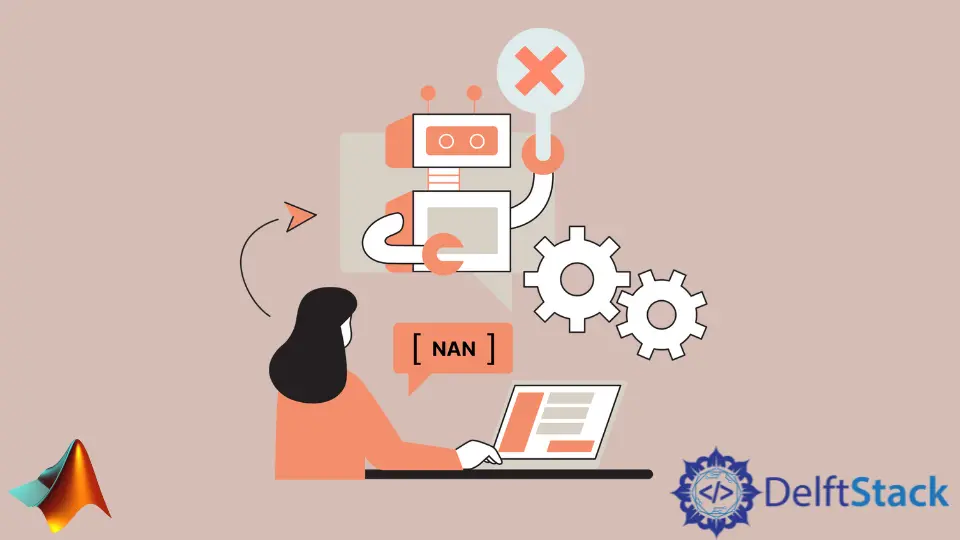
Dealing with NaN
(Not a Number) values is a common task in data analysis, and MATLAB provides several effective methods for handling these missing values in vectors.
In this article, we will explore various techniques, focusing on functions like isnan()
, fillmissing()
, and rmmissing()
to clean vectors from NaN
entries.
MATLAB Remove NaN
Values From Vector Using the isnan()
Function
NaN
values are undefined values that are generated in the case of arithmetic operations. To remove them from a vector, we have to find their position or index, and then we can set the NaN
value equal to null.
To do this, we can use the isnan()
function, which returns a logical array of zeros and ones to find the position of NaN
values. The ones in the logical array represent NaN
values, and the zeros in the array represent other values.
The syntax for the isnan()
function is as follows:
TF = isnan(X)
Here, X
is the input array or matrix.
Suppose the input vector contains complex values; the isnan()
function returns 0
when both the real and imaginary parts do not contain NaN
and 1
when real or imaginary parts contain Nan
. We can use the output of the isnan()
function to assign the NaN
values a null or empty vector.
For example, let’s create a vector containing NaN
values and remove them using the isnan()
function. See the code below.
v = [1 2 nan 2 nan];
i = isnan(v);
v(i) = [];
disp('v = ');
disp(v);
Output:
v =
1 2 2
In the above code, the variable i
is a logical array that contains ones at the position of NaN
values, and we used these index values to set the NaN
values equal to the empty vector. We can see in the above output that the NaN
values are removed from the vector v
.
Moreover, we can also replace the NaN
values with other values. For example, let’s replace the NaN
values in a vector with zero.
v = [1 2 nan 2 nan]
i = isnan(v)
v(i) = 0
Output:
v =
1 2 NaN 2 NaN
i =
0 0 1 0 1
v =
1 2 0 2 0
Now, let’s consider another scenario. Suppose we have two vectors that depend on each other, like the first value of the first vector depends on the first value of the second vector.
There are NaN
values in the vectors, and we want to remove them and the corresponding value of the other vector to maintain their interdependence. We also want the output vectors to have the same size.
One effective method to achieve this is by finding the indices of NaN
values in both vectors and then combining them using logical OR
. This combined result serves as a guide to simultaneously remove NaN
values and their corresponding elements from both vectors.
For example, let’s create two vectors and remove their NaN
value and the corresponding values present in both vectors.
v1 = [1 2 nan 2 nan]
v2 = [NaN 1 0 2 5]
i1 = isnan(v1);
i2 = isnan(v2);
i = i1 | i2
v1(i) = []
v2(i) = []
Output:
v1 =
1 2 NaN 2 NaN
v2 =
NaN 1 0 2 5
i =
1 0 1 0 1
v1 =
2 2
v2 =
1 2
In the above code, we used the symbol |
for logical OR
to combine the two logical arrays. We can see that the output vectors no longer have NaN
values and their size is equal.
If we want to remove NaN
values only if they are present in both vectors at the same position, we can use the logical AND
in place of the logical OR
. For example, let’s create two vectors and remove the NaN
value from them only if the values are present at the same index.
v1 = [1 2 nan 2 nan];
v2 = [1 0 nan 5 6];
i1 = isnan(v1);
i2 = isnan(v2);
i = i1 & i2;
v1(i) = []
v2(i) = []
Output:
v1 =
1 2 2 NaN
v2 =
1 0 5 6
In the above output, both vectors’ first NaN
value is present at the same position and removed in the output. The second NaN
value is not removed because its corresponding value is not NaN
.
MATLAB Remove NaN
Values From Vector Using the fillmissing()
Function
In addition to the isnan()
function, MATLAB provides the fillmissing()
function, introduced in R2019b, to efficiently handle NaN
values in a vector. This function offers a versatile approach by allowing the replacement or interpolation of missing values, including NaN
.
The syntax is as follows:
outputVector = fillmissing(inputVector, 'method');
Here, inputVector
is the original vector containing NaN
values, and the 'method'
parameter specifies how the missing values should be handled. To remove NaN
values, we use the method 'constant'
with a replacement value of NaN
.
The fillmissing()
function works by replacing or interpolating missing values in the input vector based on the specified method. When used with the 'constant'
method, it replaces NaN
values with the specified constant, effectively removing them from the vector.
Now, let’s walk through a complete example to demonstrate the usage of the fillmissing()
function.
originalVector = [1, 2, NaN, 4, NaN, 6];
cleanedVector = fillmissing(originalVector, 'constant', 0);
disp('Original Vector:');
disp(originalVector);
disp('Cleaned Vector:');
disp(cleanedVector);
Here, we start by defining a vector originalVector
containing numerical values and NaN
entries. The fillmissing()
function is then applied to remove NaN
values, and the result is stored in the cleanedVector
variable.
The second argument, 'constant,'
, specifies the method to replace missing values with a constant, and 0
ensures that NaN
values are replaced with 0
.
The disp
function is used to display both the original and cleaned vectors, allowing us to observe the impact of the fillmissing()
function on the input vector.
Output:
Original Vector:
1 2 NaN 4 NaN 6
Cleaned Vector:
1 2 0 4 0 6
Let’s have another code example to showcase the application of fillmissing()
in interpolating NaN
values in a vector.
vector_with_nan = [1, 2, NaN, 4, NaN, 6];
vector_interpolated = fillmissing(vector_with_nan, 'linear');
disp('Original Vector:');
disp(vector_with_nan);
disp('Cleaned Vector:');
disp(vector_interpolated);
Output:
Original Vector:
1 2 NaN 4 NaN 6
Cleaned Vector:
1 2 3 4 5 6
In this example, we begin with a vector, vector_with_nan
, containing NaN
values at specific positions. To address these missing entries, we utilize the fillmissing()
function with the 'linear'
method.
The 'linear'
method performs linear interpolation, calculating intermediate values for NaN
entries based on the surrounding non-NaN
values. This results in a vector, vector_interpolated
, where the NaN
values have been effectively interpolated.
The fillmissing()
function in MATLAB proves to be a valuable tool for data preprocessing, offering multiple methods for handling NaN
values. Whether you prefer to replace NaN
with constants or interpolate values, the fillmissing()
function streamlines the process of working with incomplete datasets in MATLAB.
MATLAB Remove NaN
Values From Vector Using the rmmissing()
Function
In MATLAB versions R2020a and later, the rmmissing()
function offers a concise way to remove missing values, including NaN
, from a vector. This function simplifies the process by eliminating NaN
entries without the need for additional logical indexing or replacement.
The basic syntax of the rmmissing()
function is:
output_vector = rmmissing(input_vector);
Here, input_vector
is the vector containing NaN
values, and output_vector
is the resulting vector with NaN
values removed.
Let’s explore several working code examples illustrating the application of rmmissing()
in removing NaN
values from a vector.
vector_with_nan = [1, 2, NaN, 4, NaN, 6];
vector_without_nan = rmmissing(vector_with_nan);
disp('Original Vector:');
disp(vector_with_nan);
disp('Cleaned Vector:');
disp(vector_without_nan);
Output:
Original Vector:
1 2 NaN 4 NaN 6
Cleaned Vector:
1 2 4 6
In this example, we start with a vector vector_with_nan
containing NaN
values. The rmmissing()
function is applied to vector_with_nan
to efficiently remove any missing values, including NaN
, from the vector, creating a new vector named vector_without_nan
.
Now, let’s see another example of how to independently remove the NaN
values from each vector using the rmmissing()
function.
v1 = [1, 2, NaN, 4, NaN, 6];
v2 = [NaN, 1, 0, 2, 5, NaN];
v1_cleaned = rmmissing(v1);
v2_cleaned = rmmissing(v2);
disp('Vector 1 (without NaN):');
disp(v1_cleaned);
disp('Vector 2 (without NaN):');
disp(v2_cleaned);
Output:
Vector 1 (without NaN):
1 2 4 6
Vector 2 (without NaN):
1 0 2 5
Here, we initialize two vectors, v1
and v2
, with numerical values, including NaN
entries. The rmmissing()
function is then applied to v1
to remove any missing values, including NaN
, from v1
, resulting in a new vector named v1_cleaned
.
Similarly, the rmmissing()
function is applied to v2
. It removes any missing values, including NaN
, from v2
, resulting in a new vector named v2_cleaned
.
Conclusion
Dealing with NaN
values in MATLAB is a crucial step in ensuring the accuracy and reliability of data analysis. Whether you choose logical indexing, interpolation, or specialized functions like rmmissing()
, MATLAB provides a powerful set of tools to handle missing values effectively.
Choose the method that best suits your data and analysis requirements for a cleaner and more robust dataset.