How to Remove Zeros From a Vector in MATLAB
-
Remove Zero Values From a Vector in MATLAB Using the
find()
Function - Remove Zero Values From a Vector in MATLAB Using Logical Indexing
-
Remove Zero Values From a Vector in MATLAB Using the
nonzeros()
Function -
Remove Zero Values From a Vector in MATLAB Using the
setdiff()
Function - Conclusion
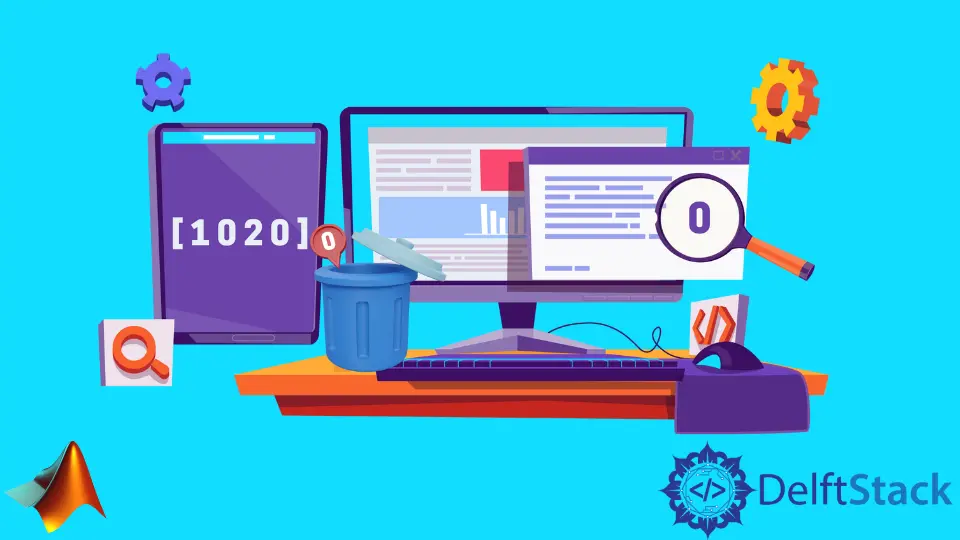
MATLAB, a powerful numerical computing environment, is widely used for data analysis, signal processing, and various scientific and engineering applications. When working with vectors, it’s not uncommon to encounter the need to eliminate zero values for efficient data manipulation and analysis.
Whether you’re dealing with experimental data, simulations, or any other numerical data set, removing zero values from a vector is a fundamental operation. In this article, we will explore different methods and techniques to achieve this task in MATLAB.
Remove Zero Values From a Vector in MATLAB Using the find()
Function
A convenient way to remove zero values from the vector using the find()
function in MATLAB. The find()
function in MATLAB is designed to locate non-zero elements in an array or vector.
It returns the indices of the non-zero elements, making it a handy tool for filtering out specific values. When applied to a vector, the find()
function can be used to identify and remove zero values efficiently.
The find()
function in MATLAB has the following syntax:
indices = find(vector, condition, count, direction);
vector
: The input vector on which the condition is applied.condition
: (Optional) The condition to be satisfied. If not specified, the default is to find nonzero elements.count
: (Optional) The maximum number of indices to be returned.direction
: (Optional) The direction of the search, either'first'
(default) or'last'
.
Remember that the condition
parameter is where you define the criteria for finding elements in the vector. It can involve logical operations (e.g., equality, greater than, less than) or other conditions based on your specific requirements.
Example 1: Removing All Zero Values
Consider a vector n
with some zero values. We want to remove these zeros using the find()
function.
clc
clear
n = [1 0 2 0 3 0];
indices = find(n == 0);
n(indices) = [];
n
In this example, we start by defining a vector n
containing some zero values. The find()
function is then employed with the condition n == 0
to identify the indices of zero values. These indices are stored in the variable indices
.
Finally, the identified indices are used to remove the zero values from the vector, resulting in a modified vector without zeros.
Output:
n =
1 2 3
Example 2: Removing Specific Zero Values
In some cases, we may want to remove only a specific number of zero values from the vector. Here, we remove the first two zeros.
clc
clear
n = [1 0 2 0 3 0];
indices = find(n == 0, 2);
n(indices) = [];
n
In this case, the second argument of the find()
function is utilized to limit the number of output indices to two, resulting in the removal of only the first two zero values from the vector n
.
Output:
n =
1 2 3 0
Example 3: Removing Last Zero Values
Now, let’s demonstrate how to remove the last two zero values from the vector.
clc
clear
n = [1 0 2 0 3 0];
indices = find(n == 0, 2, 'last');
n(indices) = [];
n
In this example, the third argument of the find()
function is used with the string 'last'
to set the direction of searching from the last element towards the first. Consequently, the last two zero values are removed from the vector n
.
Output:
n =
1 0 2 3
These examples demonstrate the flexibility and utility of the find()
function in MATLAB for removing zero values from vectors.
Remove Zero Values From a Vector in MATLAB Using Logical Indexing
While the find()
function provides a powerful approach to removing zero values from a vector in MATLAB, another effective method involves using logical indexing. This technique leverages the logical conditions directly applied to the vector, enabling a concise and intuitive way to filter and manipulate data.
The syntax for removing zero values using logical indexing is straightforward:
vector = vector(vector ~= 0);
This line of code creates a logical condition (vector ~= 0
), which evaluates to a logical array with true
where the condition is satisfied and false
elsewhere. The logical array is then used to index into the original vector, extracting only the elements that meet the specified condition.
Example: Removing Zero Values Using Logical Indexing
Consider a vector n
containing some zero values. We aim to remove these zero values using logical indexing.
clc
clear
n = [1 0 2 0 3 0];
n = n(n ~= 0);
n
In this example, the condition n ~= 0
is applied to the vector n
, creating a logical array with true
at positions where the elements are not equal to zero. The logical array is then used to index into the vector n
, retaining only the elements that satisfy the condition.
This concise approach eliminates the need for a separate function like find()
.
Output:
n =
1 2 3
In this output, we observe that the vector n
now contains only the non-zero values, effectively removing the zeros through logical indexing.
Logical indexing provides a direct and readable way to filter elements based on a condition. It is particularly useful when the condition is simple, such as removing zeros, as shown in this example.
Remove Zero Values From a Vector in MATLAB Using the nonzeros()
Function
In addition to logical indexing and the find()
function, MATLAB offers a specialized function called nonzeros()
that simplifies the process of removing zero values from a vector. This function is designed specifically for extracting the non-zero elements of an array, providing a concise and efficient solution.
The syntax for using the nonzeros()
function is straightforward:
resultVector = nonzeros(originalVector);
This line of code takes an input vector (originalVector
) and returns a new vector (resultVector
) containing only the non-zero elements of the original vector.
Example: Removing Zero Values Using the nonzeros()
Function
Consider a vector n
that includes some zero values. Our objective is to remove these zero values using the nonzeros()
function.
clc
clear
n = [1 0 2 0 3 0];
resultVector = nonzeros(n);
resultVector
In this example, the nonzeros()
function is applied to the vector n
. The function automatically extracts all the non-zero elements from the original vector, creating a new vector (resultVector
) that contains only the non-zero values.
This provides a concise and readable solution for removing zero values without the need for explicit conditions or indexing.
Output:
resultVector =
1
2
3
The output reveals that the nonzeros()
function successfully extracted the non-zero values from the original vector n
, resulting in a new vector containing only these non-zero elements.
The nonzeros()
function offers a streamlined approach for situations where the goal is to isolate non-zero values from a vector. Its simplicity enhances code readability and is particularly useful when the removal of zero values is the primary objective.
Remove Zero Values From a Vector in MATLAB Using the setdiff()
Function
Another effective method for removing zero values from a vector involves the use of the setdiff()
function. This function allows us to find the set difference between two arrays, providing a convenient way to extract elements that are unique to the original vector and not present in the set of zero values.
The syntax for using the setdiff()
function is as follows:
resultVector = setdiff(originalVector, zeroValues);
originalVector
: The vector from which zero values are to be removed.zeroValues
: The values to be excluded from the result, typically set to zero in this case.resultVector
: The vector containing the elements unique tooriginalVector
after removing elements present inzeroValues
.
Example: Removing Zero Values Using the setdiff()
Function
Consider a vector n
that contains some zero values. The goal is to remove these zero values using the setdiff()
function.
clc
clear
n = [1 0 2 0 3 0];
zeroValues = 0;
resultVector = setdiff(n, zeroValues);
resultVector
In this example, the setdiff()
function is applied to the vector n
and the zero values. The function calculates the set difference, providing resultVector
with only the elements unique to n
after excluding the zero values.
Output:
resultVector =
1 2 3
The output reveals that the setdiff()
function successfully removed the zero values from the original vector n
, resulting in resultVector
containing only the non-zero elements.
The setdiff()
function can also handle cases where multiple zero values exist in the vector. For example, if the vector n
had repeated zeros, the function would still return a vector without any duplication of non-zero values.
The order of elements in the resulting vector (resultVector
) is preserved from the original vector (n
), making setdiff()
particularly useful when the order of elements matters.
Conclusion
Efficiently handling zero values in MATLAB vectors is important for accurate data analysis and computation. Each method presented in this article, from logical indexing to the specialized functions nonzeros()
and setdiff()
, offers a unique set of advantages.
Whether prioritizing code simplicity, flexibility, or specific functionality, you can choose the method that best aligns with your project requirements. You can streamline your MATLAB code, ensuring the robustness and accuracy of your data processing workflows.